Creating Dynamic React Hooks with the Help of GPT
Updated on March 31, 2025

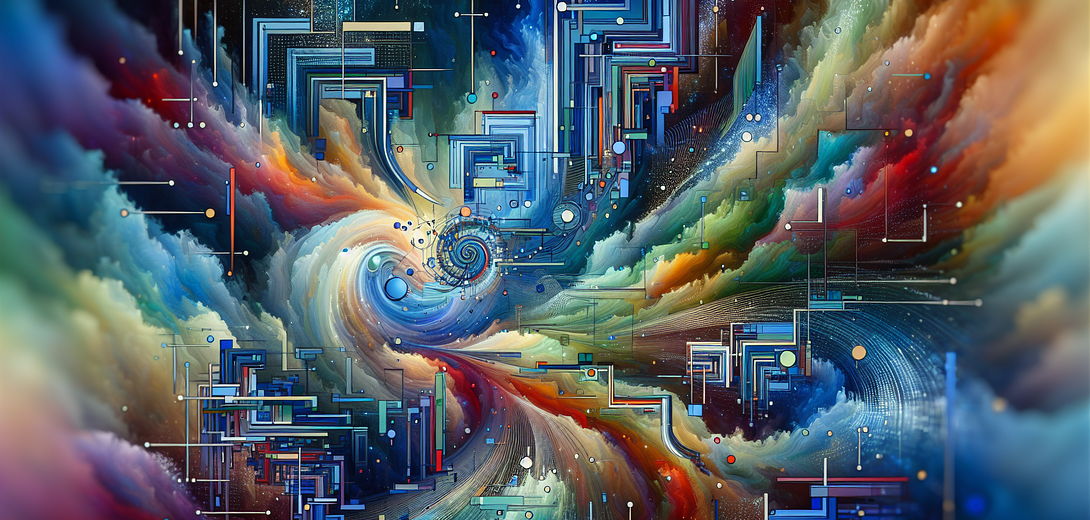
In the modern web development space, React hooks provide a powerful way to work with stateful logic in function components. However, writing hooks from scratch can sometimes be complex and time-consuming. In this tutorial, we’ll explore how you can leverage the Cloving CLI to create dynamic React hooks efficiently and with enhanced quality.
By integrating Cloving into your development workflow, you can supercharge your productivity and take full advantage of AI to assist in coding tasks.
Understanding Cloving CLI
Cloving is an AI-powered command-line interface tool that augments the coding experience. It assists developers by generating relevant code snippets, providing intelligently crafted code reviews, and more, all thanks to AI capabilities.
1. Setting Up Cloving
To get started with Cloving, we need to install and configure it.
Installation:
npm install -g cloving@latest
Configuration:
Run the configuration command to set up Cloving with your preferred AI model and API key:
cloving config
Follow the interactive prompts to complete the setup.
2. Initializing Your Project
Before generating React hooks, ensure Cloving is initialized in your project directory:
cloving init
This creates a cloving.json
file that includes metadata about your project, allowing Cloving to craft contextually relevant code.
3. Generating Dynamic React Hooks
Let’s say you want to create a custom React hook to manage a counter state with the ability to increment, decrement, and reset it. You can achieve this with Cloving’s code generation feature.
Command:
cloving generate code --prompt "Create a custom React hook to manage a counter with increment, decrement, and reset functionality" --files src/hooks/useCounter.ts
Generated Code:
// src/hooks/useCounter.ts
import { useState } from 'react';
function useCounter(initialValue: number = 0) {
const [count, setCount] = useState(initialValue);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
const reset = () => setCount(initialValue);
return { count, increment, decrement, reset };
}
export default useCounter;
The above code creates a basic yet functional custom hook that can be easily imported and utilized across your React components.
4. Reviewing and Refining Hooks
Cloving allows you to review generated code and make further modifications if needed. You can enhance the hook with more features or tailor it to specific needs using the interactive prompt:
Revise the hook to include a step increment feature and return a function to directly set the new value.
5. Generating Unit Tests for Hooks
Ensure your hooks are robust by generating unit tests with Cloving. This helps validate the behavior of your custom hooks:
cloving generate unit-tests -f src/hooks/useCounter.ts
Sample Unit Test Code:
// src/hooks/useCounter.test.ts
import { renderHook, act } from '@testing-library/react-hooks';
import useCounter from './useCounter';
describe('useCounter', () => {
it('should increment the counter', () => {
const { result } = renderHook(() => useCounter(0));
act(() => {
result.current.increment();
});
expect(result.current.count).toBe(1);
});
it('should decrement the counter', () => {
const { result } = renderHook(() => useCounter(5));
act(() => {
result.current.decrement();
});
expect(result.current.count).toBe(4);
});
it('should reset the counter', () => {
const { result } = renderHook(() => useCounter(5));
act(() => {
result.current.increment();
result.current.reset();
});
expect(result.current.count).toBe(5);
});
});
6. Leveraging Cloving Chat for Assistance
For more complex hooks or when you require detailed guidance, utilize Cloving’s interactive chat:
cloving chat -f src/hooks/useCounter.ts
This allows you to ask questions, receive explanations, or iterate on the hooks development with real-time AI assistance.
7. Best Practices for Using Cloving with React Hooks
- Initialization: Always initialize Cloving in your project folder to maintain context.
- Prompts: Be specific with your prompts for precise code generation.
- Reviews: Take advantage of Cloving’s code review feature to ensure quality and adherence to best practices.
- Testing: Generate and validate unit tests to ensure the integrity and reliability of your hooks.
Conclusion
Harnessing the power of Cloving CLI to create dynamic React hooks can greatly enhance your productivity and ensure high code quality. By integrating AI into your coding process, you can focus more on creative and complex problems, leveraging automated processes for repetitive and foundational tasks.
With Cloving, take your React development to the next level by effortlessly incorporating AI-driven code generation, reviews, and testing. Experiment with Cloving’s features and see how much more efficient your workflow can become!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.