Creating Django Models with GPT-Assisted Code Generation
Updated on March 30, 2025

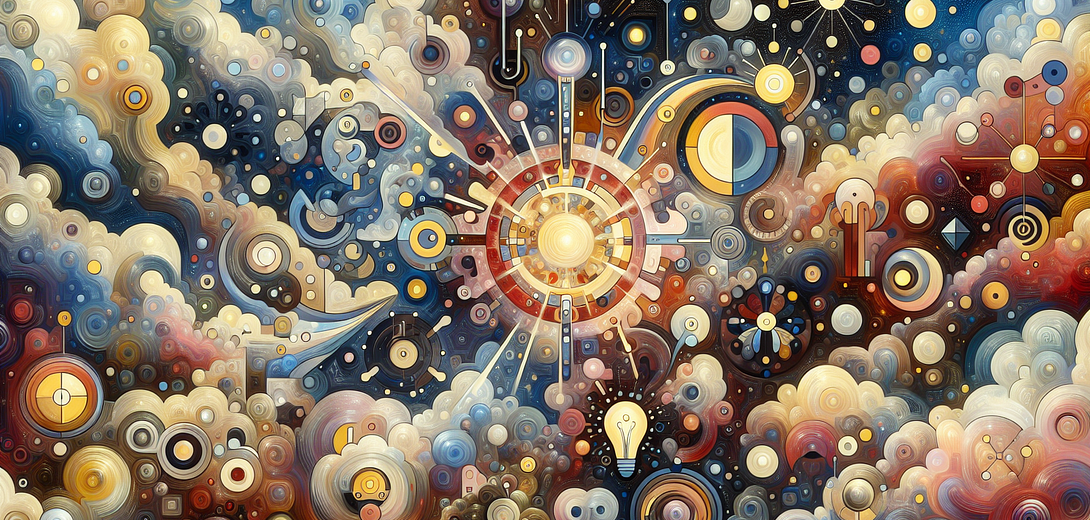
For developers working with Django, creating models often forms the backbone of application logic. Yet, drafting these models can be time-consuming, especially for intricate databases. The Cloving CLI tool offers an AI-powered way to streamline this process, allowing developers to focus more on functionality and less on boilerplate code. Let’s dive into how you can leverage Cloving CLI for creating Django models efficiently.
Understanding Cloving CLI
Cloving is an innovative command-line tool that integrates AI to assist developers by generating relevant code. It comprehends project contexts, making it an ideal partner for generating Django models seamlessly.
1. Setting Up Cloving CLI
Before you begin using Cloving for Django models, ensure it’s installed and configured correctly:
Installation:
Install Cloving globally via npm:
npm install -g cloving@latest
Configuration:
You’ll need to set up your API key and preferred models:
cloving config
Follow the interactive steps to configure your SDKs and other settings.
2. Getting Started with Cloving in Your Project
Initialization assists Cloving in understanding project-specific contexts:
cloving init
This command creates a cloving.json
file containing metadata about your project, aiding Cloving in crafting tailored suggestions.
3. Using Cloving to Generate Django Models
Suppose you need to create Django models for a blog application with posts and comments features. Here’s how to use Cloving for quick generation:
Example:
Run the following command to generate models:
cloving generate code --prompt "Create Django models for a blog with posts and comments" --files blog/models.py
This triggers Cloving’s AI to understand your needs and the context:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
published_date = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
class Comment(models.Model):
post = models.ForeignKey(Post, related_name='comments', on_delete=models.CASCADE)
author = models.CharField(max_length=100)
text = models.TextField()
created_date = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.text
The generated code provides Django models aligned with your requirements. You can refine these models as needed.
4. Reviewing and Modifying Generated Models
After generation, you can use Cloving to review or further edit your code. You can prompt the tool for explanations:
Refine the Comment model to include a status field with 'pending' and 'approved' options.
Cloving will update the model with your refinements making it more cohesive with your functionality requirements.
5. Generating Unit Tests for Django Models
Testing Django models ensures their reliability and functionality align with expectations. Use Cloving to simplify this task:
cloving generate unit-tests -f blog/models.py
It will create unit test templates specific to each model.
from django.test import TestCase
from blog.models import Post, Comment
class PostModelTest(TestCase):
def setUp(self):
self.post = Post.objects.create(title='Sample Post', content='Just a sample post content.')
def test_post_creation(self):
self.assertEqual(self.post.title, 'Sample Post')
class CommentModelTest(TestCase):
def setUp(self):
self.post = Post.objects.create(title='Sample Post', content='Just a sample post content.')
self.comment = Comment.objects.create(post=self.post, author='John Doe', text='Sample comment.')
def test_comment_creation(self):
self.assertEqual(self.comment.text, 'Sample comment.')
The tests are tailored to validate fundamental functionality.
6. Interactive Development with Cloving Chat
Leverage Cloving’s chat feature for deeper insights or complex code assistance:
cloving chat -f blog/models.py
In chat mode, you can interactively request revisions, ask questions, or seek detailed explanations.
7. Creating Informative Commit Messages with Cloving
Cloving can also help craft meaningful Git commit messages, improving version control and collaboration:
cloving commit
It analyzes your code changes and proposes relevant commit messages.
Conclusion
Integrating the Cloving CLI into your Django development routine rearranges the focus from repetitive coding to creative problem-solving, enhancing both productivity and code quality. With the AI-driven assistance in generating models, crafting tests, or revising code, Cloving unleashes a new level of efficiency for developers.
Explore the possibilities with Cloving and revolutionize how you code — smarter, faster, and more accurately. Remember, Cloving is a tool to assist your expertise, complementing your skillset with AI capabilities.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.