Creating Custom GraphQL Resolvers with GPT-Driven Code Generation
Updated on March 05, 2025

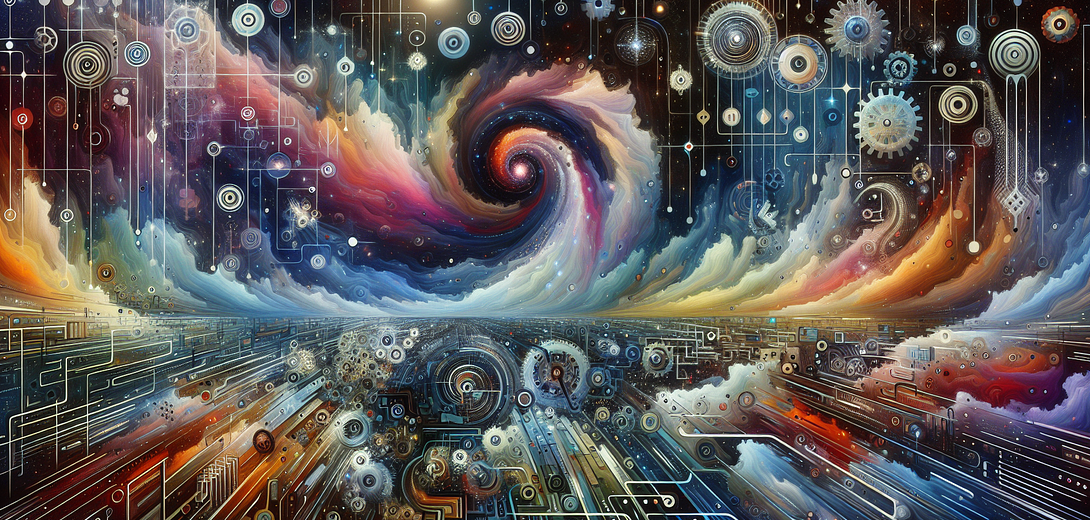
Boosting GraphQL Resolver Development with Cloving CLI
GraphQL has revolutionized how developers structure APIs by allowing precise data fetching, reduced network overhead, and better client-server interactions. However, writing and maintaining custom GraphQL resolvers can be time-consuming—especially in complex domains. Cloving CLI, an AI-powered command-line interface integrating GPT-driven code generation, streamlines the process by automating much of the boilerplate and scaffolding work. This tutorial walks you through using Cloving CLI effectively to build custom resolvers, highlighting best practices and advanced use cases.
1. Setting Up Cloving
Before diving into resolver generation, ensure that Cloving CLI is installed and correctly configured in your environment.
1.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
1.2 Configuration
Configure Cloving with your API key and preferred GPT model:
cloving config
Follow the interactive prompts to complete the setup, including specifying your API key and AI model (e.g., GPT-3.5 or GPT-4).
1.3 Initializing the Project
Initialize Cloving in your project directory to give it the necessary context:
cloving init
This command creates a cloving.json
file, storing metadata about your project and code structure. With this information, Cloving can tailor its generated output to match your coding conventions.
2. Generating Custom GraphQL Resolvers
With Cloving configured, you can generate GraphQL resolvers for common tasks, whether they’re simple read operations or complex write/update flows.
2.1 Example: Creating a User Profile Resolver
Imagine you’re building a social media application with a GraphQL API that fetches user profiles. Instead of manually writing the resolver, you can let Cloving generate the boilerplate:
cloving generate code --prompt "Create a GraphQL resolver to fetch user profile by ID" --files src/resolvers/userResolver.ts
Expected Output:
// src/resolvers/userResolver.ts
import { users } from '../data/users';
const getUserProfile = {
Query: {
userProfile: (_: any, { id }: { id: string }) => {
return users.find(user => user.id === id);
},
},
};
export default getUserProfile;
Cloving references your existing project structure or hypothetical data/users
to produce relevant code. If your structure or data files differ, Cloving can adapt based on the information in cloving.json
.
3. Refining Generated Code
3.1 Revising and Adding Features
After generating resolvers, you may need additional logic, such as error handling, authentication checks, or field validations. Simply instruct Cloving to revise your code:
Revise the resolver to include error handling if the user is not found.
Cloving responds with:
const getUserProfile = {
Query: {
userProfile: (_: any, { id }: { id: string }) => {
const user = users.find(u => u.id === id);
if (!user) {
throw new Error(`User with ID ${id} not found.`);
}
return user;
},
},
};
3.2 Authentication and Authorization
Many GraphQL resolvers require authentication checks to control data access. You can prompt Cloving to enforce user roles or tokens:
Add an authentication check that throws an error if there is no valid auth token.
Cloving might produce:
userProfile: (_: any, { id }: { id: string }, context: any) => {
if (!context.user) {
throw new Error('Unauthorized');
}
// ...
}
By iterating with Cloving, you can incorporate best practices without re-writing large sections of code manually.
4. Testing Your Resolvers
Unit tests ensure your resolvers behave correctly under various scenarios—such as invalid input, missing data, or permission failures. Cloving can automatically generate these tests:
cloving generate unit-tests -f src/resolvers/userResolver.ts
Sample Test:
// src/resolvers/userResolver.test.ts
import getUserProfile from './userResolver';
describe('getUserProfile', () => {
it('should return a user profile for a valid ID', () => {
const result = getUserProfile.Query.userProfile({}, { id: '1' });
expect(result).toEqual({ id: '1', name: 'Alice', age: 25 });
});
it('should throw an error if the user is not found', () => {
expect(() => {
getUserProfile.Query.userProfile({}, { id: '999' });
}).toThrow('User with ID 999 not found.');
});
});
Cloving leverages your existing code to create a meaningful test suite, covering edge cases like user-not-found scenarios.
5. Engaging Cloving Chat for Advanced Queries
Some coding tasks or design decisions may not be suited for a single prompt. If you’re dealing with complex GraphQL features—like batched resolvers, subscriptions, or file uploads—Cloving’s interactive chat helps you refine ideas in real time.
cloving chat -f src/resolvers/userResolver.ts
Within this interactive session, you can request:
How can I optimize this resolver to batch multiple user lookups using DataLoader?
Cloving can then suggest code changes, best practices, or references to libraries like DataLoader for more efficient data fetching.
6. Advanced Use Cases
6.1 Mutations with Complex Logic
From creating nested objects to handling multi-step transactions, certain resolvers can become quite intricate. For instance, you could prompt:
cloving generate code --prompt "Create a GraphQL mutation resolver for creating a new post with tags, ensuring database transactions are handled properly" --files src/resolvers/postResolver.ts
Cloving can scaffold the mutation logic, including calls to your ORM or database transaction patterns—shortening the time you spend writing boilerplate or looking up reference code.
6.2 Subscriptions for Real-Time Features
If your GraphQL API supports subscriptions, Cloving can help build subscription resolvers that push real-time updates to clients. Just specify your requirements:
Implement a subscription that notifies clients whenever a new user is created.
This approach can quickly produce standard subscription patterns, which you can refine (e.g., filtering by user roles or merging data from different sources).
6.3 Schema Merging and Federation
If your setup involves multiple microservices or a schema federation approach (like Apollo Federation), you can prompt Cloving to generate resolvers that integrate seamlessly with your gateway:
Generate an Apollo Federation-compatible resolver for the User entity with external fields.
Cloving can produce code that references @key
or @extends
directives, ensuring compliance with Federation’s extended schema features.
7. Best Practices for Successful GraphQL + Cloving Workflows
- Maintain a Clear Schema: Cloving relies on your codebase structure. If your GraphQL schema is well-organized (using
.graphql
files or annotated TypeScript classes), Cloving will provide more accurate scaffolding. - Incremental Prompts: Ask for small, iterative changes rather than large leaps, ensuring Cloving’s code remains clean and cohesive.
- Refine and Review: AI-generated code is a strong starting point—but always review logic, apply domain knowledge, and verify security or performance considerations.
- Use Context: Keep your directory structure consistent. If Cloving can see your data models (e.g.,
User
orPost
), it can generate resolvers with the correct references automatically. - Combine Tools: Integrate Cloving with other GraphQL dev tools such as GraphQL Code Generator or testing libraries to build a robust ecosystem.
8. Committing AI-Generated Code
Once you’re satisfied with newly generated or refined resolvers, let Cloving help you create context-aware commit messages:
cloving commit
Cloving will analyze the changes and propose a summary message, like:
Add custom user profile resolver with enhanced error handling and unit tests
You can accept or modify this message before confirming the commit, keeping your version history clear and concise.
9. Conclusion
By embracing Cloving CLI for GraphQL resolver generation, you leverage an AI-powered pair programmer to speed up mundane tasks, ensure coding best practices, and stay focused on the core logic of your application. Whether you’re creating a single field-level resolver or orchestrating complex multi-step mutations, Cloving significantly reduces boilerplate and fosters code quality.
Remember: Cloving complements—rather than replaces—your expertise. You remain the architect of your API’s design and logic, while Cloving jumpstarts implementation details. As you refine your GraphQL approach, consider using Cloving’s iterative refinement, interactive chat, and unit test generation features to maintain a clean, efficient, and scalable codebase.
Happy coding! Let Cloving handle the boilerplate so you can deliver robust, high-quality GraphQL APIs with ease.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.