Crafting Interactive UI Components with GPT in React
Updated on March 30, 2025

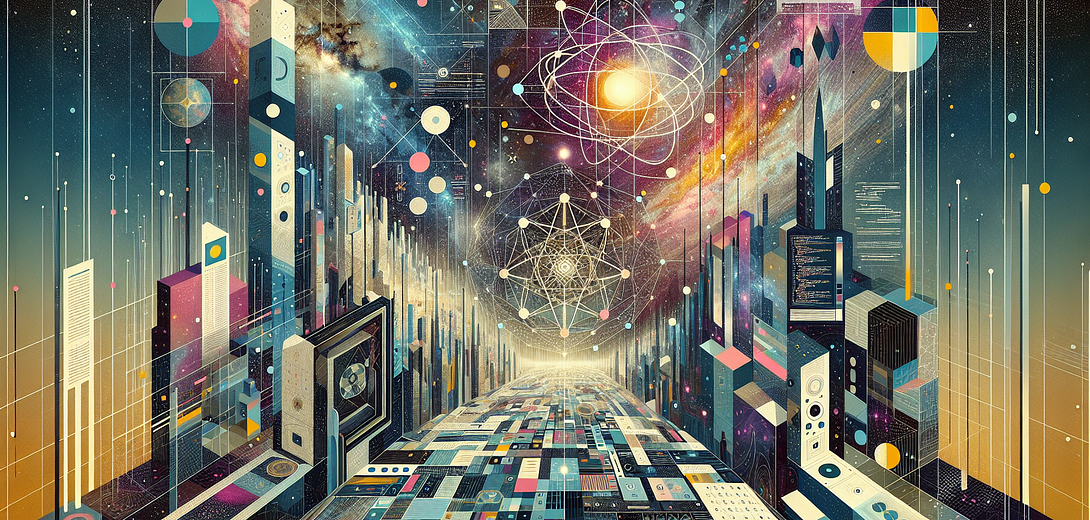
In the realm of modern web development, crafting interactive UI components is as much an art as it is a science. By leveraging powerful tools like the Cloving CLI, you can harness the prowess of AI—such as GPT—to assist in building dynamic and interactive React components. In this guide, I’ll walk you through using the Cloving CLI to enhance your React component development workflow.
Understanding the Cloving CLI
Cloving acts as an AI-powered command-line tool that supplements your programming tasks. From generating code snippets to interactive chats, Cloving adds a new dimension to developer productivity and efficiency.
Setting Up Cloving
Before diving into the React component creation, ensure that Cloving is correctly configured in your environment.
Installation:
To install Cloving globally via npm, execute:
npm install -g cloving@latest
Configuration:
Begin with configuring Cloving to use the preferred AI model:
cloving config
Follow the on-screen instructions to set up your API key, model preferences, and other configurations.
Initializing Your Project
For Cloving to access your project context, initialize it in your working directory:
cloving init
This prepares your project for Cloving, allowing it to generate contextually aware solutions, thanks to the cloving.json
file created during initialization.
Crafting React Components with Cloving
Now that Cloving is set up, let’s see how we can craft an interactive UI component—a button with a chat-like interaction in React.
Generating a React Component
To generate a React component, use Cloving’s generate code feature. For our interactive button functionality:
cloving generate code --prompt "Create a React button component that toggles a chat window" --files src/components/ChatButton.tsx
Cloving intelligently produces relevant React code based on the project context and prompt. Here’s a potential outcome:
// src/components/ChatButton.tsx
import React, { useState } from 'react';
const ChatButton: React.FC = () => {
const [isOpen, setIsOpen] = useState(false);
const toggleChat = () => {
setIsOpen(!isOpen);
};
return (
<div>
<button onClick={toggleChat}>
{isOpen ? 'Close Chat' : 'Open Chat'}
</button>
{isOpen && (
<div className="chat-window">
<h2>Chat with us!</h2>
<p>How can we assist you today?</p>
</div>
)}
</div>
);
};
export default ChatButton;
This component toggles the chat window visibility based on the button state, an essential interactive feature.
Tailoring and Reviewing Generated Code
Use Cloving’s interactive features to tailor generated components. After generating the code, you can refine, review, or even seek explanations:
Revise the ChatButton to include a smooth transition when toggling the chat window.
With Cloving, refinement becomes an iterative and straightforward task.
Generating Unit Tests
Quality is vital when crafting UI components. Cloving simplifies the process of generating unit tests, crucial for verifying your components’ functionality:
cloving generate unit-tests -f src/components/ChatButton.tsx
This creates robust unit tests to ensure your interactive components respond correctly to user actions.
Interactive Support via Cloving Chat
For intricate assistance, Cloving’s chat feature proves invaluable. Use it to explore complex requirements seamlessly:
cloving chat -f src/components/ChatButton.tsx
During the session, pose questions, ask for further features, and integrate AI-generated suggestions into your workflow effortlessly.
Using Cloving for Automated Commit Messages
When changes are made, Cloving aids in generating insightful commit messages, adding context and clarity to version histories:
cloving commit
You’ll receive a thoughtful, AI-generated commit message that encapsulates the current changes succinctly.
Conclusion
Integrating AI-powered tools like the Cloving CLI into your development process augments your ability to craft interactive React components efficiently. With Cloving’s features for code generation, unit testing, and interactive chat, your React workflow becomes more streamlined, allowing creativity and productivity to flourish in tandem.
Embrace the Cloving CLI as a collaborator enhancing your engineering practices, leveraging AI to refine the art of developing dynamic and interactive UI components in React.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.