Crafting High-Performance C++ Algorithms Using GPT Assistance
Updated on February 06, 2025

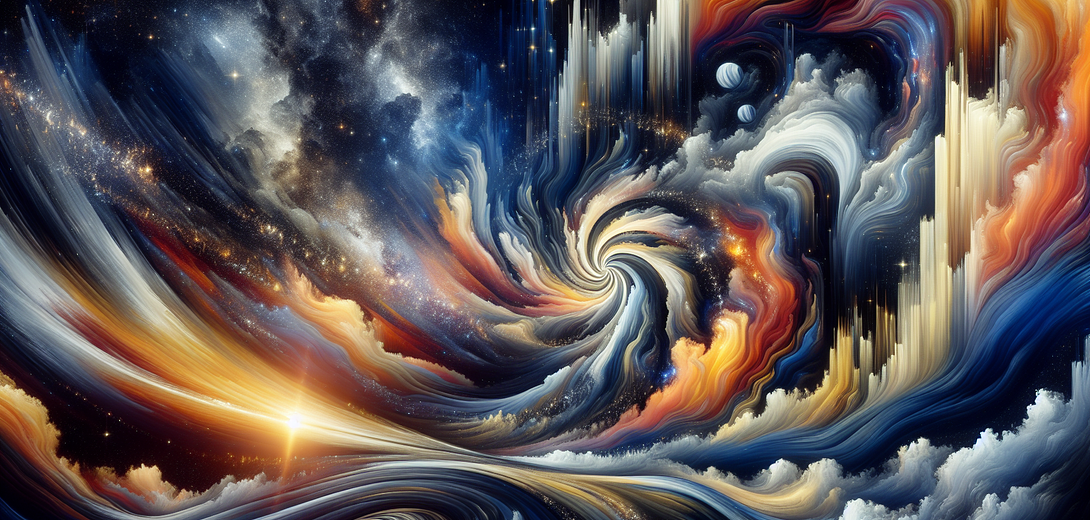
In the world of software development, crafting efficient and high-performance algorithms is vital, especially in languages like C++. With AI tools becoming more prevalent, leveraging such tools can significantly enhance your workflow. One such tool is the Cloving CLI—a command-line interface powered by AI that can assist in various coding tasks, from generating code to creating unit tests and more. In today’s post, we’ll explore how you can craft high-performance C++ algorithms with the assistance of Cloving CLI.
Understanding Cloving CLI
Cloving CLI is an open-source tool that integrates AI into your development workflow. It serves as an AI-powered pair programmer, offering suggestions, generating code, conducting code reviews, and much more. This can be particularly helpful in C++, where performance and precision matter greatly.
1. Setting Up Cloving
Before diving into algorithm development, you need to set up Cloving in your development environment.
Installation:
To install Cloving, run the following command:
npm install -g cloving@latest
Configuration:
Once installed, configure Cloving by selecting your AI model and entering your API key:
cloving config
Follow the interactive prompts to complete the configuration and tailor Cloving to your project needs.
2. Initializing Your C++ Project
To provide Cloving with the necessary context about your C++ project, initialize it in your project directory:
cloving init
This sets up a cloving.json
file that contains metadata about your project’s structure, enhancing Cloving’s ability to assist you effectively.
3. Generating C++ Algorithms
Let’s look at an example of generating a high-performance C++ algorithm using Cloving.
Example:
Suppose you’re tasked with developing an efficient sorting algorithm. You can use the Cloving CLI to generate a C++ implementation:
cloving generate code --prompt "Develop a high-performance quicksort algorithm in C++" --files src/SortAlgorithm.cpp
This command will utilize the context from src/SortAlgorithm.cpp
and generate a quicksort algorithm optimized for C++. Here’s a basic implementation that may be generated:
#include <vector>
void quicksort(std::vector<int>& arr, int left, int right) {
int i = left, j = right;
int pivot = arr[(left + right) / 2];
while (i <= j) {
while (arr[i] < pivot) i++;
while (arr[j] > pivot) j--;
if (i <= j) {
std::swap(arr[i], arr[j]);
i++; j--;
}
}
if (left < j) quicksort(arr, left, j);
if (i < right) quicksort(arr, i, right);
}
4. Reviewing and Optimizing Code
Once you have generated the code, it’s critical to review and optimize it. Utilize Cloving’s interactive prompts to request revisions or explanations:
Optimize the quicksort algorithm for better performance
Cloving will analyze the code and provide suggestions or make changes to improve the algorithm’s performance.
5. Generating Unit Tests
Testing your algorithm is critical to ensure correctness and performance. Use Cloving to create unit tests for your sorting algorithm:
cloving generate unit-tests -f src/SortAlgorithm.cpp
This will generate tests that validate your quicksort implementation, ensuring it handles various edge cases gracefully.
6. Utilizing Cloving Chat for Complex Issues
For more intricate algorithmic challenges, initiate a Cloving chat session:
cloving chat -f src/SortAlgorithm.cpp
This opens an interactive session where you can probe the AI for insights, alternative approaches, or debugging help related to your algorithm.
7. Crafting Precise Commit Messages
After developing and testing your algorithm, use Cloving to generate thoughtful commit messages:
cloving commit
This command generates a commit message that summarizes your changes effectively, maintaining a clear historical record of your project’s development.
Conclusion
By integrating Cloving CLI into your C++ development workflow, you can harness the power of AI to streamline the process of crafting high-performance algorithms. From generating initial code to refining and testing your algorithms, Cloving offers invaluable assistance, allowing you to focus on optimizing and polishing your code.
Remember, while Cloving can significantly boost productivity, it is a tool meant to augment your expertise. Use its capabilities wisely, and let it enhance, not replace, your critical thinking and problem-solving skills.
Explore Cloving CLI today and transform your C++ algorithm development process!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.