Crafting Efficient RESTful Endpoints in Node.js with GPT's Guidance
Updated on April 01, 2025

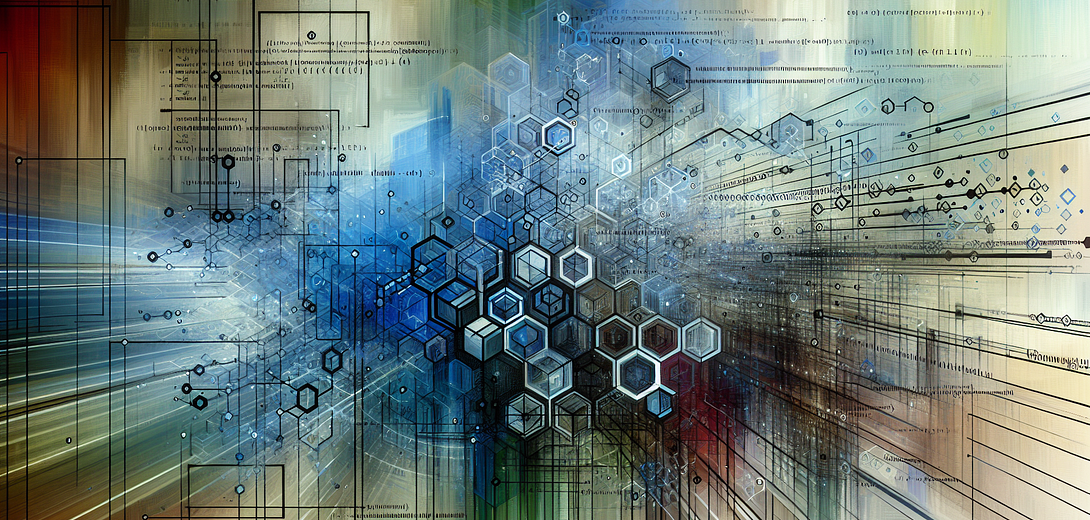
In the modern web development landscape, creating efficient RESTful endpoints is essential for building robust and scalable APIs. By leveraging the power of Cloving CLI, an AI-powered command-line tool, developers can enhance their productivity and code quality when crafting RESTful endpoints in Node.js. In this tutorial, we’ll explore how Cloving can be used to streamline your development process and provide guidance when building RESTful services.
Introduction to Cloving CLI
Cloving is a versatile CLI tool that integrates AI into your development workflow. It acts as a powerful AI pair programmer that assists with code generation, code reviews, and more. Whether you’re setting up a new Node.js project or adding new features to an existing one, Cloving can help you write cleaner, more efficient code.
1. Setting Up Cloving
First, ensure that Cloving is installed and configured on your machine.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to use your preferred AI model:
cloving config
This command will guide you through setting up your API key and selecting the AI model that suits your needs.
2. Initializing a Node.js Project with Cloving
To let Cloving understand the context of your project, initialize it in your Node.js project directory:
cloving init
This command generates a cloving.json
file in your project directory, which contains metadata about your application. This file helps Cloving tailor its suggestions to your project’s specific requirements.
3. Generating RESTful Endpoints
Now let’s dive into generating RESTful endpoints using Cloving.
Example:
Suppose you’re building a Node.js API for a book store and need to create an endpoint to fetch book details. You can use the cloving generate code
command:
cloving generate code --prompt "Create a RESTful endpoint to get book details by ID in Express" --files routes/books.js
Cloving will analyze your project context (Express in this case) and generate the relevant code. Here’s what the generated code might look like:
// routes/books.js
const express = require('express');
const router = express.Router();
// Mock database
const books = [
{ id: 1, title: '1984', author: 'George Orwell' },
{ id: 2, title: 'Brave New World', author: 'Aldous Huxley' }
];
// Get book details by ID
router.get('/books/:id', (req, res) => {
const bookId = parseInt(req.params.id);
const book = books.find(b => b.id === bookId);
if (book) {
res.json(book);
} else {
res.status(404).send('Book not found');
}
});
module.exports = router;
4. Reviewing and Customizing Generated Code
Once Cloving generates the endpoint code, take the opportunity to review and customize it to fit your specific project needs. You can refine the logic, handle errors more gracefully, or optimize performance as needed.
5. Generating Unit Tests for Endpoints
To ensure your endpoint is working as expected, use Cloving to generate unit tests:
cloving generate unit-tests -f routes/books.js
Cloving will generate tailored unit tests to verify your endpoint’s functionality:
// tests/routes/books.test.js
const request = require('supertest');
const express = require('express');
const booksRouter = require('../routes/books');
const app = express();
app.use('/api', booksRouter);
describe('GET /api/books/:id', () => {
it('responds with book details when book exists', async () => {
const res = await request(app).get('/api/books/1');
expect(res.statusCode).toBe(200);
expect(res.body).toEqual({ id: 1, title: '1984', author: 'George Orwell' });
});
it('responds with 404 when book does not exist', async () => {
const res = await request(app).get('/api/books/999');
expect(res.statusCode).toBe(404);
});
});
6. Using Cloving Chat for Interactive Guidance
If you encounter challenges or need ongoing assistance while developing your endpoints, the Cloving chat feature can be immensely helpful:
cloving chat -f routes/books.js
This command opens an interactive chat session with the AI, allowing you to ask questions, request code snippets, or get explanations about your Node.js project.
7. Enhancing Endpoint Efficiency
Beyond code generation, Cloving can assist with revising your endpoints for better performance. Use the interactive prompt to request optimization tips or security enhancements for your Node.js API:
cloving> Optimize the endpoint to handle large request payloads efficiently
8. Crafting Meaningful Commit Messages
Finally, as you make changes to your Node.js project, Cloving can help you craft meaningful commit messages:
cloving commit
This command generates a contextual commit message based on your code changes, which you can review and edit before finalizing.
Conclusion
Crafting efficient RESTful endpoints with the Cloving CLI tool demonstrates the true potential of integrating AI into your development workflow. By leveraging Cloving’s capabilities, you can enhance API design, improve code quality, and boost your productivity when working with Node.js. Embrace Cloving and transform how you interact with code, making your development process more intuitive and efficient.
Remember, Cloving is a powerful aid designed to complement your programming skills. Use it as a reliable partner to help you build high-quality, responsive APIs.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.