Crafting Effective Error-Handling Routines in C++ Using GPT
Updated on January 07, 2025

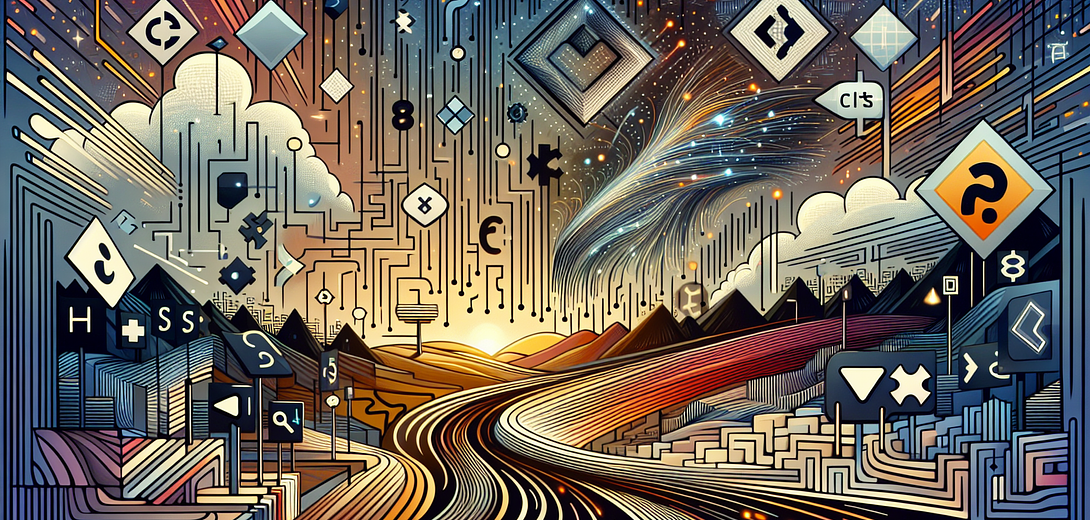
Error handling is a critical aspect of software development, especially in a language like C++ where manual memory management can introduce a myriad of issues. The Cloving CLI, equipped with AI-powered capabilities, can significantly aid developers in crafting effective error-handling routines. In this guide, we’ll demonstrate how to leverage the Cloving CLI to enhance your error-management strategies in C++.
1. Setting Up Cloving
Before exploring error-handling routines, ensure that Cloving is installed and configured properly in your environment.
Installation:
Begin by installing Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving with your preferred AI model:
cloving config
Follow the interactive prompts to enter your API key, select an AI model, and adjust additional settings for personalized recommendations.
2. Initializing Your C++ Project
Cloving requires a contextual understanding of your project to provide relevant code suggestions. Initialize Cloving in your C++ project directory as follows:
cloving init
This command will create a cloving.json
file with essential metadata and preferences for your project.
3. Using Cloving for Error-Handling Enhancements
Example Scenario
Consider a scenario where you’re managing exceptions in a file I/O operation in C++. Let’s see how Cloving can assist in generating robust error-handling routines.
Generating Code:
Use the cloving generate code
command to create error-handling routines:
cloving generate code --prompt "Generate error-handling code for file operations in C++" --files src/file_manager.cpp
This command will leverage AI to analyze your file’s context (file_manager.cpp
in this case) and create error-handling routines tailored to your project. The output might look something like this:
#include <iostream>
#include <fstream>
#include <string>
#include <exception>
void readFile(const std::string& fileName) {
try {
std::ifstream file(fileName);
if (!file.is_open()) {
throw std::ios_base::failure("File not found or unable to open");
}
// Read file contents
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
// Further handling
}
}
This generated code includes exception handling for file operations, significantly improving robustness.
4. Refining and Revising Error-Handling Code
Cloving CLI provides interactive options for revising and refining the generated code. After generation, engage with Cloving to improve your error-handling routines:
Interactive Revision:
Use the interactive prompt to request changes or additions:
add recovery logic for failed file open attempts
Cloving will provide additional code or suggestions to help manage such scenarios more effectively.
5. Generating Explanations and Documentation
Understanding the generated code is crucial. Cloving can also generate explanations or detailed documentation for your solutions:
cloving generate code --prompt "Explain the error-handling logic in readFile function" --files src/file_manager.cpp
This will break down the logic and provide insightful commentary, aiding in better comprehension and maintenance of your code.
6. Leveraging Cloving Chat for Complex Error-Handling Scenarios
For intricate error-handling logic, initiate a chat session with Cloving AI:
cloving chat -f src/file_manager.cpp
In this interactive session, ask questions, request code snippets, or seek advice on handling specific exceptions efficiently. This chat mode provides a dynamic space for complex problem-solving.
7. Integrating Cloving for Code Review
Ensure your error-handling logic is up to par by conducting an AI-powered code review:
cloving generate review
Cloving will analyze your entire project and provide feedback, helping to identify potential issues and suggesting enhancements for better error management.
Conclusion
By integrating Cloving CLI into your development workflow, you can significantly enhance your error-handling routines in C++. Cloving provides tailored code generation, interactive revisions, and insightful reviews—transforming AI into a powerful tool in your toolkit. Whether you’re hardening your file operations or ensuring comprehensive exception handling, Cloving’s capabilities will augment your programming skills and improve code quality.
Utilize Cloving not just as a code generator but as a continuous learning and improvement tool. Embrace AI-powered assistants like Cloving to effectively manage the nuances of C++ error handling and become a more efficient and effective programmer.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.