Crafting Dynamic Web Pages Using Next.js and GPT Insights
Updated on January 07, 2025

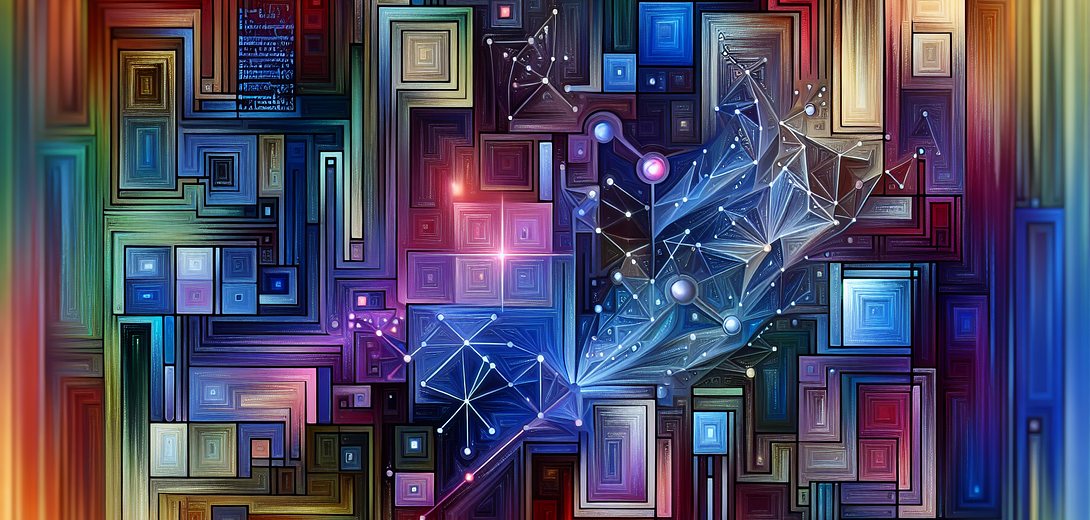
Crafting Dynamic Web Pages Using Next.js and GPT Insights
In the modern web development landscape, Next.js stands out as a powerful framework for building dynamic web pages. By integrating AI-powered insights with Cloving CLI, developers can craft web experiences that are both reactive and intelligent. This post will walk you through leveraging Cloving CLI to supercharge your Next.js development, optimizing both productivity and code quality.
Setting the Stage with Cloving CLI
Before diving into AI-enhanced web development, ensure Cloving is installed and configured in your environment. This tool will be pivotal in integrating AI workflows into your next.js project.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Set up your AI model preferences with Cloving:
cloving config
Follow the prompts to input your API key and choose models suited to your workflow.
Initializing Your Next.js Project with Cloving
Kickstart your Next.js project setup by initializing Cloving in your project’s root directory:
cloving init
This command will establish a cloving.json
as well as gather essential project metadata for context-aware operations.
Generate a Dynamic Page with Cloving
Let’s explore how Cloving can streamline page creation in a Next.js project. Suppose you’re tasked with creating a dynamic product detail page:
Code Generation:
Prompt Cloving to generate a Next.js page component:
cloving generate code --prompt "Create a Next.js page component for displaying product details dynamically" --files pages/product/[id].tsx
Generated Component:
// pages/product/[id].tsx
import { useRouter } from 'next/router';
import { useEffect, useState } from 'react';
export default function ProductPage() {
const router = useRouter();
const { id } = router.query;
const [product, setProduct] = useState(null);
useEffect(() => {
if (id) {
// Fetch product details dynamically based on id
fetch(`/api/product/${id}`)
.then(response => response.json())
.then(data => setProduct(data));
}
}, [id]);
if (!product) return <div>Loading...</div>;
return (
<div>
<h1>{product.name}</h1>
<p>{product.description}</p>
<p>Price: ${product.price}</p>
</div>
);
}
This code sets up a basic dynamic page that fetches product data based on the route parameter.
Optimize Component with AI-Powered Insights
Enhance your component by incorporating AI suggestions. Use the cloving chat
feature for interactive feedback:
cloving chat -f pages/product/[id].tsx
Within the chat, request improvements:
cloving> How can I improve the loading state and error handling for this product page?
Cloving might suggest enhancing the UX with loading indicators or adding error boundaries.
Generate a Smart API Route with Cloving
Beyond the frontend, Cloving aids in backend API creation:
Example:
Generate a simple API route for products:
cloving generate code --prompt "Create an API route in Next.js for fetching product details by ID" --files pages/api/product/[id].ts
Generated API Code:
// pages/api/product/[id].ts
import type { NextApiRequest, NextApiResponse } from 'next';
export default function handler(req: NextApiRequest, res: NextApiResponse) {
const { id } = req.query;
// Simulated database call
const product = getProductFromDatabase(id);
if (product) {
res.status(200).json(product);
} else {
res.status(404).json({ error: 'Product not found' });
}
}
// Mock function to simulate database call
function getProductFromDatabase(id: string) {
const products = [
{ id: '1', name: 'Product A', description: 'Description A', price: 10 },
{ id: '2', name: 'Product B', description: 'Description B', price: 20 },
];
return products.find(product => product.id === id);
}
Review and Finalize Code
After generating your code, leverage Cloving’s review capabilities:
cloving generate review
This will provide AI-driven suggestions to refine your code further, improving performance or adhering to best practices.
Leveraging Cloving for Effective Git Management
Craft precise, meaningful commit messages with Cloving when committing code changes:
cloving commit
This command analyzes changes in your git repository and generates suggestions for insightful commit messages.
Conclusion
The combination of Next.js and Cloving CLI elevates dynamic web page development. By mastering Cloving’s code generation and AI-assisted features, you unlock a streamlined, intelligent development process ready for 21st-century web demands. Integrate Cloving into your workflow today and supercharge your coding efficiency and quality.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.