Crafting AI-Enhanced Frontend Components with GPT for Single Page Applications
Updated on April 21, 2025

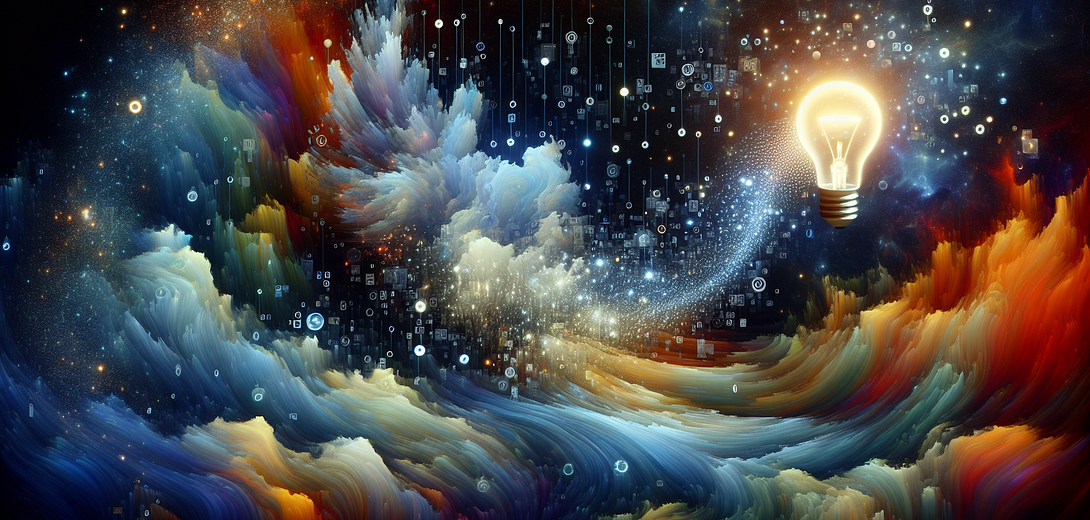
As a frontend developer, creating robust and efficient components is crucial for building seamless and responsive single page applications (SPAs). With the rise of AI technologies, tools like the Cloving CLI can radically optimize this process by integrating AI-powered code generation directly into your workflow. In this tutorial, we’ll explore how Cloving can assist you in crafting AI-enhanced frontend components for SPAs with higher efficiency and quality.
Getting Started with Cloving CLI
Installation and Configuration
First, ensure that you have Cloving installed and configured in your development environment. Follow these steps:
Install Cloving:
npm install -g cloving@latest
Configure Cloving:
To interact with Cloving, you’ll need to configure it with your API key and preferred AI model:
cloving config
Follow the prompts to input your API key and select the model you wish to use.
Initializing Your Project
Before generating any code, initialize Cloving in your project directory to set the context for the AI:
cloving init
This will create a cloving.json
file containing metadata about your project.
Crafting Frontend Components with Cloving
Now that we have Cloving set up, let’s delve into generating a frontend component for a SPA.
1. Generating a Component
Suppose you’re developing a SPA using React and need a new component for a shopping cart. Here’s how you can leverage Cloving to generate it:
cloving generate code --prompt "Create a React component for a dynamic shopping cart" --files src/components/ShoppingCart.jsx
Output Example:
import React, { useState } from 'react';
const ShoppingCart = ({ items }) => {
const [cartItems, setCartItems] = useState(items);
const handleRemove = (id) => {
setCartItems(cartItems.filter(item => item.id !== id));
};
return (
<div className="shopping-cart">
<h2>Your Shopping Cart</h2>
<ul>
{cartItems.map(item => (
<li key={item.id}>
{item.name} - ${item.price}
<button onClick={() => handleRemove(item.id)}>Remove</button>
</li>
))}
</ul>
</div>
);
};
export default ShoppingCart;
2. Enhancing the Component with AI Suggestions
After you generate the initial code, Cloving provides options to enhance and refine your components. For example, you can run the following to enter an interactive session and request further changes:
cloving chat -f src/components/ShoppingCart.jsx
You might ask for performance optimizations or additional features like sorting items:
cloving> Can you make the shopping cart sort items by price?
Cloving will then provide suggestions or directly modify the code accordingly.
3. Generating Unit Tests
Once your component is ready, ensure its reliability with unit tests:
cloving generate unit-tests -f src/components/ShoppingCart.jsx
Generated Tests:
import { render, screen, fireEvent } from '@testing-library/react';
import ShoppingCart from './ShoppingCart';
test('removes item from cart', () => {
const items = [{ id: 1, name: 'Book', price: 9.99 }];
render(<ShoppingCart items={items} />);
fireEvent.click(screen.getByText(/Remove/i));
expect(screen.queryByText(/Book/i)).toBeNull();
});
4. Leveraging AI for Commit Messages
To maintain clear and precise version control, use Cloving to create commit messages:
cloving commit
Cloving analyzes changes and generates a contextual commit message, such as:
Added ShoppingCart component with basic functionality and remove feature
Best Practices for Using Cloving
- Initialize Regularly: Run
cloving init
whenever starting new components or projects to ensure Cloving understands the context. - Interactive Development: Use
cloving chat
for iterative improvements, leveraging the AI’s suggestions. - Thoroughly Test: Always generate unit tests for any component changes to maintain code quality.
- Contextual Commits: Utilize
cloving commit
to generate clear, descriptive commit messages for better project management.
Conclusion
Integrating AI into your development workflow with Cloving CLI streamlines the process of crafting frontend components for SPAs. By automating repetitive tasks and enhancing code quality, Cloving allows developers to focus on creative problem solving and user-centric design. Harness the power of AI to transform your coding experience and boost productivity in your frontend projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.