Building Your First Python Web Scraper with GPT
Updated on April 14, 2025

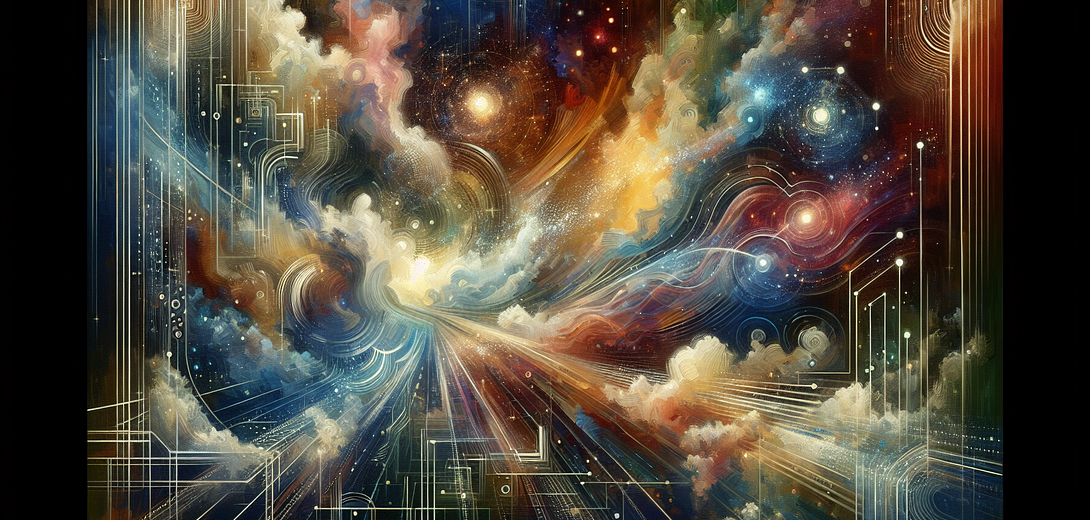
Web scraping is a powerful technique used to gather data from websites. In this tutorial, we’ll demonstrate how to build your first Python web scraper using the Cloving CLI tool. By leveraging Cloving’s AI capabilities, you’ll efficiently create a web scraper to extract data at scale.
Getting Started with Cloving
Before embarking on creating a web scraper, let’s set up Cloving to streamline the development process.
1. Installation and Configuration
First, you need to install Cloving and configure it to fit your needs:
Installation:
Install Cloving CLI globally using npm:
npm install -g cloving@latest
Configuration:
Run the Cloving configuration command to set your preferred AI model and API key:
cloving config
Follow the interactive prompts to finalize your setup. This establishes a solid foundation for integrating Cloving’s AI into your workflow.
2. Project Initialization
You need to initialize Cloving in your project directory to allow it to understand your project context:
cloving init
This step gives Cloving the ability to integrate seamlessly with your project by recognizing its structure and relevant files.
Building the Web Scraper
3. Creating the Web Scraper
With the setup complete, we can start creating our web scraper. Let’s generate a basic Python web scraper using the Cloving CLI.
Example:
Suppose you want to scrape data from a website. Use the cloving generate code
command with an appropriate prompt:
cloving generate code --prompt "Create a Python web scraper that extracts titles and links from a blog page" --files scraper.py
Cloving uses the context of your project to generate a complete scraping script. Here is a possible generated code snippet:
# scraper.py
import requests
from bs4 import BeautifulSoup
def scrape_blog_titles_links(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
articles = soup.find_all('article')
for article in articles:
title_tag = article.find('h2')
link_tag = article.find('a', href=True)
title = title_tag.text if title_tag else "No Title"
link = link_tag['href'] if link_tag else "No Link"
print(f'Title: {title}\nLink: {link}\n')
# Example usage
scrape_blog_titles_links('https://example-blog.com')
This script utilizes requests
for making HTTP requests and BeautifulSoup
for parsing HTML content to extract blog titles and their corresponding links.
4. Revising the Web Scraper
You may want to enhance your web scraper with additional features, like handling pages with AJAX content. Use the interactive Cloving chat feature to iterate on the script:
$ cloving chat -f scraper.py
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
cloving> Add functionality to handle AJAX loaded content
The AI will provide insightful iterations to transform your script into one more capable of handling dynamic websites loading content via AJAX.
Using Cloving’s Features and Best Practices
5. Interactive Chat for Problem Solving
Cloving’s chat option offers enhanced collaboration:
cloving chat --files scraper.py
Engage interactively to fine-tune your script, diagnose errors, or handle complex scraping situations.
Additional Commands:
- Save your progress at any stage
- Revise based on AI suggestions
- Commit changes with
cloving commit
to maintain clean commit history
6. Estimate Tokens for API Py Loads
Before running large-scale scrapes, estimate API usage with:
cloving tokens
This helps in optimizing and budgeting API requests efficiently.
Completing Your Web Scraper
7. Unit Testing
Create tests to ensure your scraper’s robustness:
cloving generate unit-tests --files scraper.py
Running this command generates unit tests tailored to your scraper, verifying its functionality and reliability.
Conclusion
By following this tutorial, you’ve successfully built a Python web scraper using Cloving CLI and AI. Cloving enhances productivity through recommendations, refining your script with quality code snippets and insights. Remember, the integration of AI augments your development capabilities, sets you on course for greater efficiency, and ensures adaptability to dynamic web environments.
Embrace Cloving’s features to explore more sophisticated applications, build efficient automation solutions, and continuously refine your development workflow. Happy scraping!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.