Building Serverless Architectures with Automated Code Generation by GPT
Updated on November 17, 2024

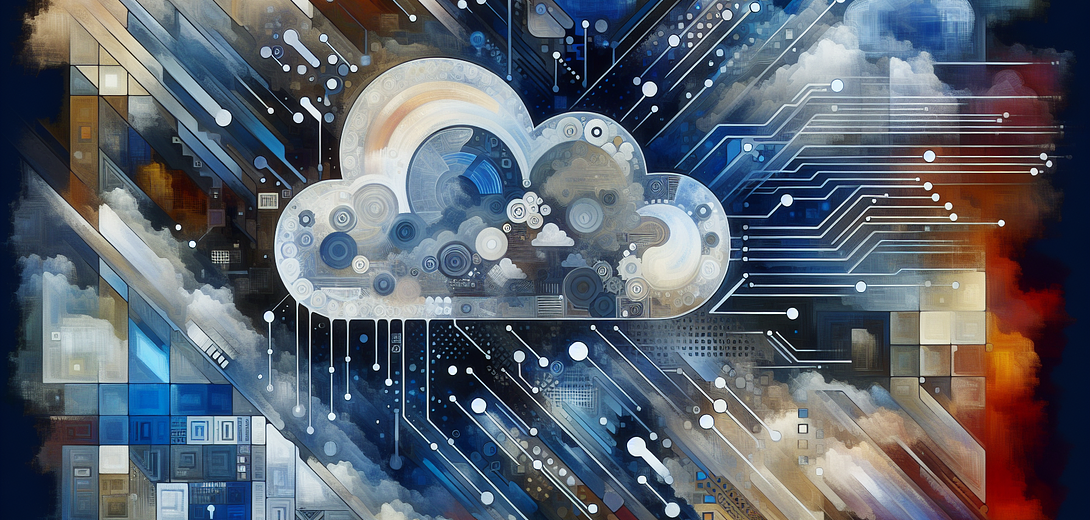
In recent years, serverless architectures have gained significant traction due to their scalability, cost-efficiency, and ease of deployment. The Cloving CLI tool, powered by AI, offers developers a seamless way to build serverless applications by automating code generation and streamlining the development workflow. In this post, we’ll explore how to leverage the Cloving CLI to construct serverless architectures with AI-assisted code generation.
Getting Started with Cloving CLI for Serverless
Before diving into serverless architectures, let’s ensure we have Cloving properly set up.
Step 1: Installing Cloving
To begin, install Cloving globally using npm:
npm install -g cloving@latest
Step 2: Configuring Cloving
Once installed, configure Cloving by setting your API key and choosing the AI model:
cloving config
Follow the interactive prompts to complete the configuration process.
Step 3: Initializing a Serverless Project
Initialize Cloving in the root of your serverless project:
cloving init
This sets up the cloving.json
file, capturing essential context about your project.
Automating Code Generation for Serverless Functions
Once initialized, you can start generating serverless function code tailored to your needs.
Example: Creating an AWS Lambda Function
Suppose you want to create an AWS Lambda function that processes incoming events and logs them to CloudWatch. Use the cloving generate code
command:
cloving generate code --prompt "Create an AWS Lambda function that logs incoming events to CloudWatch" --files src/lambda.js
Cloving leverages the context to generate relevant code:
// src/lambda.js
const AWS = require('aws-sdk');
const cloudwatchlogs = new AWS.CloudWatchLogs();
exports.handler = async (event) => {
console.log('Received event:', JSON.stringify(event, null, 2));
// Log to CloudWatch
const params = {
logEvents: [
{
message: JSON.stringify(event),
timestamp: Date.now()
}
],
logGroupName: 'your-log-group',
logStreamName: 'your-log-stream'
};
await cloudwatchlogs.putLogEvents(params).promise();
return { statusCode: 200, body: 'Event logged successfully' };
};
Reviewing and Saving the Function
After generating the function, you can review it in Cloving’s interactive session. Opt to save the code if it meets your requirements:
What would you like to do?
1. Revise
2. Explain
3. Save a Source Code File
4. Save All Source Code Files
5. Copy Source Code to Clipboard
6. Copy Entire Response to Clipboard
7. Done
Automating the Creation of Infrastructure as Code
Serverless applications often require infrastructure setup. Cloving can help automate the generation of cloud configuration templates using Infrastructure as Code (IaC) tools.
Example: Creating a CloudFormation Template
To generate an AWS CloudFormation template for deploying the Lambda function:
cloving generate code --prompt "Generate a CloudFormation template to deploy an AWS Lambda that logs events" --files src/template.yaml
Cloving generates a preliminary CloudFormation template:
# src/template.yaml
Resources:
LambdaFunction:
Type: AWS::Lambda::Function
Properties:
Handler: lambda.handler
Role: arn:aws:iam::123456789012:role/lambda-execution-role
Code:
S3Bucket: your-code-bucket
S3Key: lambda.zip
Runtime: nodejs12.x
LogGroup:
Type: AWS::Logs::LogGroup
Properties:
LogGroupName: /aws/lambda/your-log-group
Utilizing Cloving Chat for Ongoing Guidance
For more complex serverless implementations, Cloving’s chat feature is invaluable. Start an interactive session to explore and refine serverless configurations:
cloving chat -f src/lambda.js
This opens a session where you can iterate on code design, get explanations, and make adjustments collaboratively with the AI.
Example Instruction
Imagine you want to modify the existing Lambda function to include error handling when logging to CloudWatch. You might instruct GPT with a prompt like:
Add error handling to the Lambda function when logging events to CloudWatch.
Resulting Change
Based on the GPT instruction, the updated code might look like this:
// src/lambda.js
const AWS = require('aws-sdk');
const cloudwatchlogs = new AWS.CloudWatchLogs();
exports.handler = async (event) => {
console.log('Received event:', JSON.stringify(event, null, 2));
// Log to CloudWatch
const params = {
logEvents: [
{
message: JSON.stringify(event),
timestamp: Date.now()
}
],
logGroupName: 'your-log-group',
logStreamName: 'your-log-stream'
};
try {
await cloudwatchlogs.putLogEvents(params).promise();
return { statusCode: 200, body: 'Event logged successfully' };
} catch (error) {
console.error('Error logging to CloudWatch:', error);
return { statusCode: 500, body: 'Failed to log event' };
}
};
Leveraging Generated Unit Tests
High-quality serverless code is verified with robust tests. Cloving facilitates unit test generation to automate testing of Lambda functions:
cloving generate unit-tests -f src/lambda.js
Cloving produces tailored unit tests to ensure your function operates as expected:
// src/lambda.test.js
const { handler } = require('./lambda');
describe('Lambda Function', () => {
it('should log incoming events', async () => {
const event = { test: 'event' };
const result = await handler(event);
expect(result.body).toBe('Event logged successfully');
});
});
Conclusion
Cloving CLI harnesses AI to transform how developers build serverless architectures. By automating code generation, infrastructure setup, and unit tests, Cloving streamlines workflows and boosts developer productivity. Embrace these AI-powered tools to optimize your serverless development endeavors.
Through interactive chats, automated code reviews, and commit generation, Cloving enables developers to focus on innovation, ensuring high-quality, efficient serverless architectures. Welcome Cloving into your workflow and elevate your serverless development to new heights.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.