Building Secure Rails Applications with GPT-Driven Code Generation
Updated on February 06, 2025

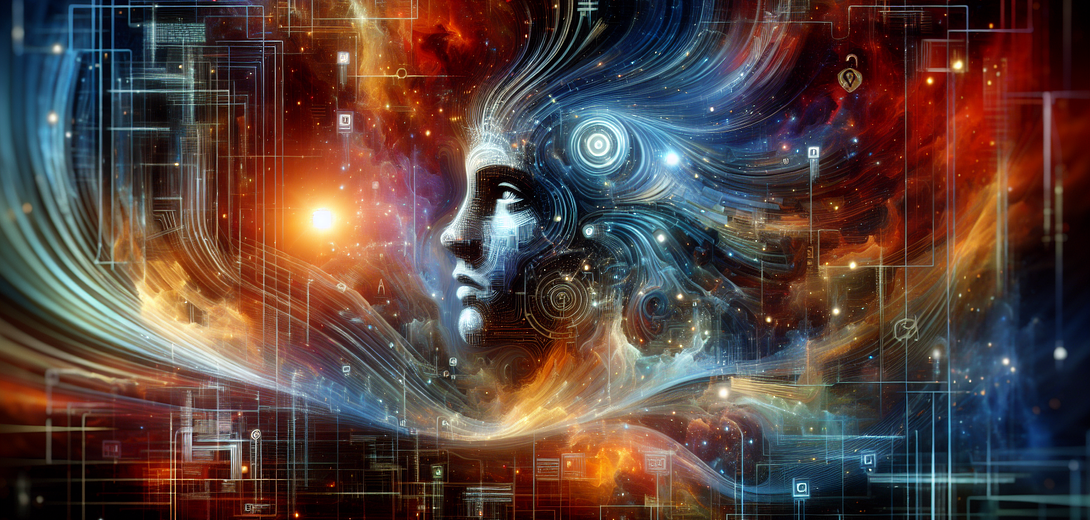
In the fast-evolving world of web development, security is paramount. With the increasing number of cyber threats, it’s vital to ensure your Rails applications are secure from the get-go. Enter Cloving CLI – an AI-powered command-line interface designed to integrate GPT-driven code generation into your workflow, enhancing code quality and boosting productivity. This tutorial will guide you through using Cloving CLI to build secure Rails applications efficiently.
Getting Started with Cloving CLI
Before you can leverage Cloving CLI for building secure Rails applications, you need to set it up in your development environment.
Installation and Configuration
Installing Cloving:
First, install Cloving globally using npm:
npm install -g cloving@latest
Configuring Cloving:
Next, you’ll need to configure Cloving by specifying your API key and preferred models:
cloving config
Follow the onscreen prompts to input your API key and select the models you prefer.
Initializing Your Rails Project with Cloving
Before any code generation can happen, you need to initialize Cloving in your Rails project:
cloving init
This command will analyze your project’s current setup and create a cloving.json
file that contains metadata about your Rails application.
Generating Secure Code with Cloving
With Cloving set up, you can now generate secure code components for your Rails application, addressing common security concerns.
1. Routine Code Generation for Security
For instance, if you’re creating a user authentication system, you might start by generating a secure login component:
cloving generate code --prompt "Create a secure login system for a Rails application" --files app/controllers/sessions_controller.rb
Cloving understands Rails contexts and will generate a secure login system leveraging best practices such as bcrypt for password hashing.
Example Code Snippet:
# app/controllers/sessions_controller.rb
class SessionsController < ApplicationController
def create
user = User.find_by(email: params[:email])
if user && user.authenticate(params[:password])
log_in user
redirect_to user
else
flash.now[:alert] = "Invalid email/password combination"
render 'new'
end
end
end
2. Generating Secure API Endpoints
If your Rails application uses APIs, Cloving can also help you generate secure endpoints:
cloving generate code --prompt "Create a secure API endpoint for user data retrieval with JSON Web Token authentication" --files app/controllers/api/users_controller.rb
Example Code Snippet:
# app/controllers/api/users_controller.rb
class Api::UsersController < ApplicationController
before_action :authenticate_with_jwt
def show
render json: current_user
end
private
def authenticate_with_jwt
jwt = request.headers['Authorization']
decoded_token = JWT.decode(jwt, ENV['JWT_SECRET'], true, algorithm: 'HS256')
@current_user = User.find_by(id: decoded_token[0]['user_id'])
rescue JWT::DecodeError, JWT::ExpiredSignature
render json: { error: 'Unauthorized access' }, status: :unauthorized
end
end
3. Enhancing Security with Unit Tests
To ensure the security features are robust, Cloving can generate unit tests for your Rails application:
cloving generate unit-tests -f app/controllers/sessions_controller.rb
Example Test Snippet:
# test/controllers/sessions_controller_test.rb
require 'test_helper'
class SessionsControllerTest < ActionDispatch::IntegrationTest
test "should get login page" do
get login_path
assert_response :success
end
test "should login with valid credentials" do
post login_path, params: { email: '[email protected]', password: 'password' }
assert_redirected_to user_path(@user)
end
end
Using Cloving Chat for Real-Time Assistance
For more elaborate or challenging tasks, leverage the Cloving chat feature which acts as an AI-powered pair programmer:
cloving chat -f app/models/user.rb
In this interactive session, you can ask for security-related advice, code snippets, or explanations that pertain specifically to your Rails project.
Best Practices and Tips
-
Contextual Awareness: Always include relevant files with the
--files
option to provide Cloving with enough context, ensuring accurate and secure code generation. -
Review Generated Code: Review AI-generated code carefully to ensure it meets your security standards. AI can aid, but human oversight is crucial.
-
Iterate Through Interactive Modes: Utilise the interactive and silent modes when generating code to streamline the review process.
-
Regular Updates: Keep Cloving CLI updated to benefit from the latest security enhancements and coding practices it incorporates.
Conclusion
Integrating Cloving CLI into your Rails development workflow can significantly enhance the security, quality, and effectiveness of your code. By leveraging GPT-driven code generation, you can proactively address security concerns, save time, and improve productivity. Remember, Cloving is not a replacement but a powerful extension of your existing coding skills. Utilize it to build robust, secure Rails applications with confidence.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.