Building Scalable Server Architectures with TypeScript and GPT
Updated on April 17, 2025

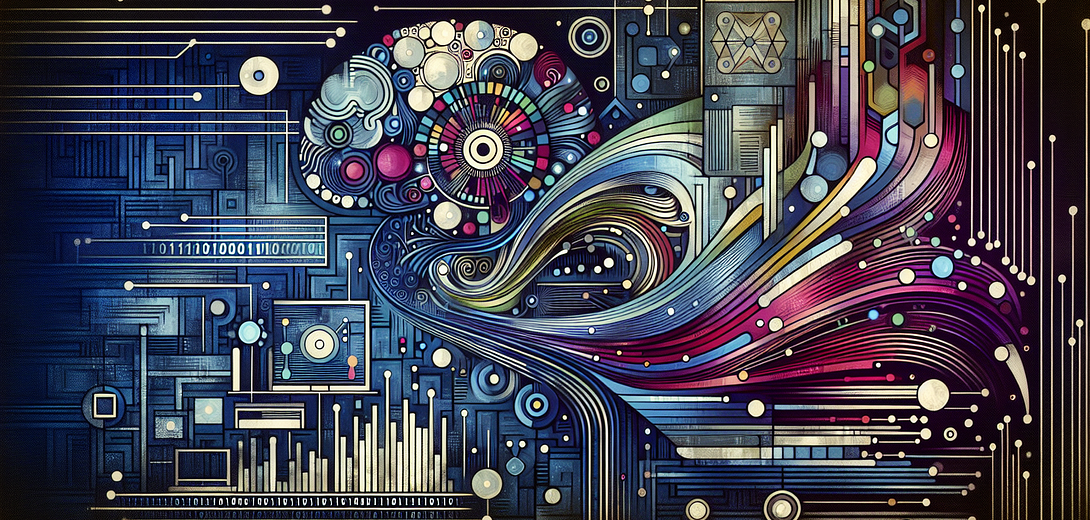
With the surge of AI-driven tools enhancing software development, Cloving CLI offers a unique approach to building scalable server architectures. This AI-powered command-line tool integrates seamlessly into your workflow, providing guidance, code snippets, and even entire modules to elevate productivity. In this tutorial, we’ll delve into how you can leverage Cloving CLI to design and implement scalable server architectures using TypeScript and GPT.
Setting Up Cloving CLI
Before we dive into server architecture, let’s set up Cloving CLI in your environment.
Installation
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration
Configure Cloving with your API key and preferred model:
cloving config
Follow the prompts to set up your API key and favorite model.
Initializing Your Project
To harness Cloving’s capabilities, initialize it within your project directory. This allows Cloving to understand your project’s context and tailor its output accordingly.
cloving init
This command creates a cloving.json
file that stores project metadata and configuration settings.
Building Your Server Architecture
Now, let’s get into building a scalable server architecture by utilizing Cloving CLI.
1. Designing the Base Architecture
First, we’ll generate the foundational code for a simple server using TypeScript. Use the generate code
command to start:
cloving generate code --prompt "Create a basic HTTP server using Express in TypeScript" --files src/index.ts
This command will produce a basic Express server setup in TypeScript, and you can expect output similar to this:
// src/index.ts
import express from 'express';
const app = express();
const PORT = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
2. Expanding the Architecture with GPT
With the base server in place, let’s enhance its capabilities using GPT. We will add an AI-driven endpoint for generating text:
cloving generate code --prompt "Add a POST /generateText endpoint that uses GPT to generate responses based on a prompt" --files src/server.ts
This command yields a new endpoint in your server:
// src/server.ts
import axios from 'axios';
app.post('/generateText', async (req, res) => {
try {
const { prompt } = req.body;
const response = await axios.post('https://api.openai.com/v1/completions', {
model: 'gpt-4o', // Replace with your selected model
prompt,
max_tokens: 150
}, {
headers: {
'Authorization': `Bearer YOUR_API_KEY`
}
});
res.json(response.data.choices[0].text);
} catch (error) {
res.status(500).send('Error generating text');
}
});
3. Introducing Middleware for Scalability
To make our server more scalable, introduce middleware for logging and error handling:
cloving generate code --prompt "Add middleware for request logging and error handling" --files src/middleware.ts
The resulting code should resemble:
// src/middleware.ts
import { Request, Response, NextFunction } from 'express';
export const logger = (req: Request, res: Response, next: NextFunction) => {
console.info(`${req.method} ${req.path}`);
next();
};
export const errorHandler = (err: Error, req: Request, res: Response, next: NextFunction) => {
console.error(err.stack);
res.status(500).send('Something broke!');
};
4. Integrating Middleware
Add this middleware to your Express server:
// src/index.ts
import { logger, errorHandler } from './middleware';
app.use(logger);
app.use(express.json());
app.use(errorHandler);
Using Cloving Chat for Continuous Development
For ongoing assistance and enhancements, employ Cloving’s chat feature:
cloving chat -f src/server.ts
In this session, you can interact with the AI to:
- Optimize existing code
- Request explanations
- Generate new endpoints or features
Example Input:
cloving> Optimize the /generateText endpoint for performance
The AI will provide suggestions and optimizations, helping you refine your server architecture.
Generating Tests to Ensure Quality
Ensuring your server runs accurately is crucial. Use Cloving to create unit tests and maintain server reliability:
cloving generate unit-tests -f src/server.ts
This command generates relevant unit tests helping you maintain robust server operations.
Conclusion
By leveraging Cloving CLI, you integrate AI into your development workflow efficiently, transforming how you approach server architecture. Whether it’s creating new endpoints, optimizing existing modules, or ensuring quality with tests, Cloving CLI brings AI-driven enhancements to your fingertips. Embrace Cloving and elevate your server building experience with TypeScript and GPT.
With Cloving at your side, you’re not just writing code, you’re crafting intelligent architecture designed to scale and succeed in today’s AI-driven landscape. Prepare to streamline your development process and transform the way you build software.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.