Building Scalable Node.js Microservices with GPT-Powered Automation
Updated on April 17, 2025

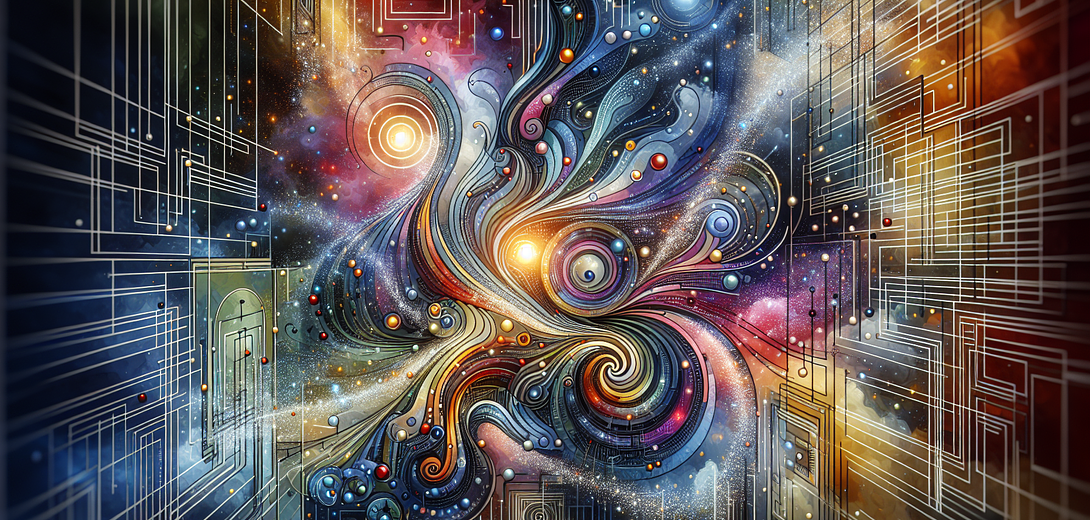
Building scalable microservices can be a daunting task, but with the Cloving CLI GPT-powered automation tool, you can streamline the process and enhance productivity. In this blog post, we’ll dive into using the Cloving CLI to efficiently build and manage Node.js microservices, leveraging AI capabilities to automate repetitive tasks and improve code quality.
Introduction to Cloving CLI
The Cloving CLI tool acts as an AI-driven assistant in your command-line environment. It enhances your development workflow by providing intelligent suggestions, generating code, and improving overall productivity. Before diving in, you’ll need to ensure Cloving is set up correctly in your development environment.
1. Installation and Configuration
First, you’ll need to install Cloving and configure it with your AI model and API key.
Installation:
Use npm to install Cloving globally:
npm install -g cloving@latest
Configuration:
Configure Cloving with your API key and selected model:
cloving config
Follow the interactive prompts to complete the setup.
2. Initializing Your Microservice Project
With Cloving installed, you’ll want to initialize it within your project directory to provide context for AI-powered features.
cloving init
Executing this command inside your microservice project directory creates a cloving.json
file. This file contains metadata about your project, helping the AI understand its context.
3. Generating Code for Microservices
One of the powerful features of Cloving is the ability to generate boilerplate code components, which is invaluable for microservice architectures. Let’s create an express-based HTTP service for a microservice.
Example:
Create an HTTP server for a “user” service in your Node.js microservice architecture.
cloving generate code --prompt "Create a Node.js express server for user service" --files src/index.js
The AI will provide code specific to initiating a Node.js express server:
// src/index.js
const express = require('express');
const app = express();
const port = process.env.PORT || 3000;
app.get('/users', (req, res) => {
res.send('Hello, this is the Users service!');
});
app.listen(port, () => {
console.log(`User service listening at http://localhost:${port}`);
});
4. AI-Assisted Code Review and Refactor
Once you have your initial code, refining it can be done using Cloving’s AI capabilities. You don’t need to generate everything from scratch; Cloving can assist in reviewing and refactoring existing code.
Perform a Code Review:
cloving generate review
Cloving will provide insights and suggestions to improve your code quality.
5. Generating Unit Tests for Microservices
With microservices, each service should ideally have thorough unit tests to ensure reliability and maintainability. Cloving can automate the creation of these tests.
Example:
Generate tests for the “user” service handler:
cloving generate unit-tests -f src/index.js
The output will contain tests specific to your existing code:
// tests/index.test.js
const request = require('supertest');
const app = require('../src/index');
describe('GET /users', () => {
it('responds with a message', async () => {
const response = await request(app).get('/users');
expect(response.statusCode).toBe(200);
expect(response.text).toBe('Hello, this is the Users service!');
});
});
6. Interactive Chat for On-the-Go AI Assistance
For more personalized, on-the-go assistance, you can interact with Cloving using chat mode. This functionality is especially useful for complex scenarios or when you’re blocked and need quick guidance.
Start a Chat Session:
cloving chat -f src/index.js
This command opens a session where you can pose specific questions or requests to the AI, and make real-time adjustments based on its feedback.
7. Automating Git Operations
Efficient microservice development often involves frequent commits. Cloving can help generate meaningful commit messages based on changes:
cloving commit
This outputs:
Implement user service endpoint with Express.js
A well-crafted commit message reflects the updates you’ve made, making collaboration and version tracking easier.
Conclusion
Building scalable Node.js microservices can be significantly enhanced with the AI capabilities provided by the Cloving CLI tool. By automating the generation of code, unit tests, commit messages, and leveraging AI-driven reviews and chat features, Cloving allows you to focus on what matters most—designing effective, maintainable microservices at scale.
Embrace Cloving as part of your development toolkit to unlock new levels of productivity and code quality in your microservice architecture.
By following this guide, you’re equipped with the knowledge needed to efficiently build and scale Node.js microservices using Cloving CLI’s GPT-powered automation. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.