Building Scalable Data Pipelines with GPT and Apache Kafka
Updated on December 05, 2024

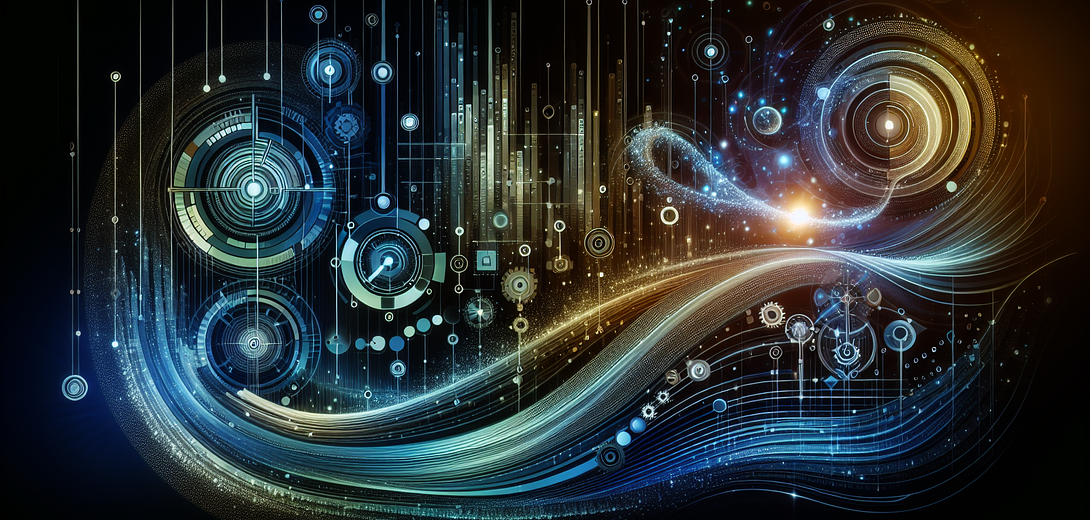
In today’s data-driven world, efficiently processing and analyzing vast amounts of data is crucial for the success of any tech-driven business. One popular combination for handling massive data streams is leveraging the power of Apache Kafka and the advanced AI capabilities of the GPT (Generative Pre-trained Transformers) model. In this blog post, we will explore how to build scalable data pipelines using Cloving CLI integrated with GPT and Apache Kafka, enhancing your workflow through AI-powered insights.
Introduction to Apache Kafka
Apache Kafka is an open-source stream-processing platform designed for handling real-time data feeds. Its core strengths lie in:
- Decoupling producers and consumers of data,
- Handling high-throughput and low-latency data flows,
- Offering a distributed, fault-tolerant architecture.
Leveraging GPT with Cloving CLI
By using Cloving CLI, you can seamlessly integrate GPT, an AI model whose capabilities range from natural language understanding to code generation, into your Kafka data pipeline. The Cloving tool acts as an AI-powered assistant, simplifying complex tasks like stream processing and real-time analytics.
1. Setup and Configuration
Install Cloving:
Ensure Cloving is installed in your environment to start building with GPT and Kafka:
npm install -g cloving@latest
Configure Cloving:
Set up Cloving with your preferred API key and model:
cloving config
Follow the prompts to provide your API key and choose the GPT model for your data pipeline tasks.
2. Initializing the Project
Before making the most of Cloving and GPT, initialize it in your Kafka project directory:
cloving init
This command collects the necessary project settings and creates a cloving.json
file for easy access and context utilization during command execution.
3. Data Pipeline Use Case
Suppose you need to process incoming log data from Kafka for analysis and prediction. This can be achieved through:
Data Ingestion:
First, create a data ingestion module to read data from Kafka:
const { Kafka } = require('kafkajs');
const kafka = new Kafka({
clientId: 'my-app',
brokers: ['kafka-broker1:9092', 'kafka-broker2:9092'],
});
const consumer = kafka.consumer({ groupId: 'log-group' });
const run = async () => {
await consumer.connect();
await consumer.subscribe({ topic: 'log-data', fromBeginning: true });
await consumer.run({
eachMessage: async ({ topic, partition, message }) => {
console.log({
value: message.value.toString(),
});
// Process data using GPT
let processedData = await processDataWithGPT(message.value.toString());
console.log(processedData);
},
});
};
run().catch(console.error);
Data Processing with GPT:
Incorporate GPT for processing:
cloving chat -f path/to/ingestion-module.js
🍀🍀🍀 Welcome to Cloving REPL 🍀🍀🍀
cloving> Generate insights from log data using GPT
Cloving offers AI-driven suggestions to extract meaningful insights or anomalies from the log data directly within the chat session, allowing seamless integration of AI with your data processing logic.
4. Enhancing Pipeline with Code Generation
With Cloving, add features like predictive analysis and anomaly detection to your data pipeline using code generation:
cloving generate code --prompt "Add anomaly detection to our Kafka data processing script" --files path/to/processor.js
Cloving will generate a script for detecting anomalies based on historical data trends, which you can customize and integrate into your pipeline.
const detectAnomalies = (data) => {
// GPT-generated function to identify unusual patterns
const anomalies = []
data.forEach(entry => {
if (entry.value > threshold) {
anomalies.push(entry)
}
});
return anomalies;
};
const processedData = detectAnomalies(logData);
5. Creating Unit Tests
Implement Cloving’s unit test generation to verify data processing modules:
cloving generate unit-tests -f path/to/processor.js
This generates tests to ensure the integrity and reliability of your data processing operations.
6. Review and Commit Automation
For reviewing code changes or generating meaningful commit messages, leverage Cloving’s commit functionalities:
cloving commit
This analyzes your changes and crafts insightful commit messages, boosting maintainability and collaboration within your development team.
Conclusion
Utilizing Cloving CLI to build scalable data pipelines by integrating GPT into Apache Kafka streams offers a powerful and dynamic approach for real-time data processing. From initial setup to data ingestion, processing, and automating tasks, the combination allows for rapid development cycles, along with AI-driven enhancements. Start integrating Cloving into your data workflows and witness the accelerated transformation of data into actionable insights.
Transform your pipelines now
Explore the vast capabilities of combining Cloving CLI, GPT, and Apache Kafka to level up your data processing projects and unlock new potential insights from your data streams.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.