Building Robust Django Middleware with GPT-Driven Automation
Updated on March 05, 2025

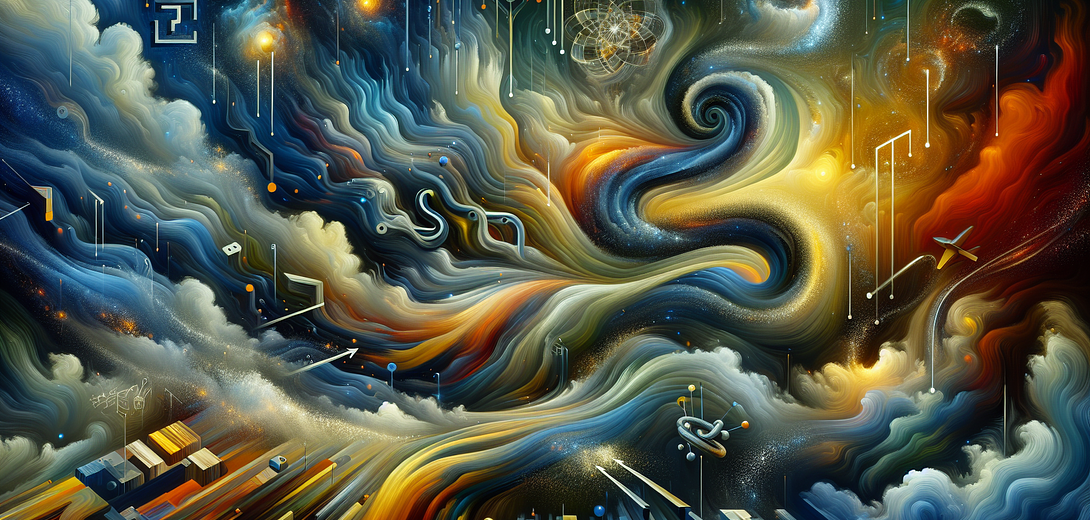
Streamlining Django Middleware Creation with Cloving CLI
Developing middleware in Django can sometimes be challenging, particularly when you need to optimize performance and maintain high code quality. By integrating Cloving CLI into your workflow, you can harness AI-driven automation to generate and refine middleware more efficiently. In this post, we’ll explore how Cloving can help you build, test, and enhance your Django middleware, leaving you free to focus on higher-level design and application logic.
1. Introduction to Cloving CLI
Cloving is a free, open-source command-line tool that uses generative AI to assist developers in writing code more efficiently. It can:
- Generate new code (like Django middleware)
- Refine and revise existing code (e.g., adding features or optimizing logic)
- Perform AI-powered code reviews to detect areas for improvement
- Create unit tests to ensure functionality and reliability
- Suggest commit messages aligned with your changes
By understanding your project’s context, Cloving provides relevant boilerplate and insights that speed up your Django development process.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
2.2 Configuration
Next, configure Cloving to use your API key and preferred AI model:
cloving config
Follow the interactive prompts to supply your credentials and set default AI model settings. Options might include GPT-3.5, GPT-4, or any model supported by Cloving.
2.3 Initializing Cloving in Your Django Project
Navigate to the root directory of your Django project and initialize Cloving:
cd /path/to/django/project
cloving init
This command generates a cloving.json
file that stores metadata about your project’s code structure, enabling Cloving to produce tailored, context-aware suggestions.
3. Generating Django Middleware Code
Once you’ve configured Cloving, generating Django middleware becomes a straightforward process.
3.1 Example: Logging Request & Response Times
Let’s say you need middleware to log request and response times for performance analysis. You can prompt Cloving as follows:
cloving generate code --prompt "Create Django middleware to log request and response times" --files middleware.py
Sample Output:
# middleware.py
import time
from django.utils.deprecation import MiddlewareMixin
class RequestTimingMiddleware(MiddlewareMixin):
def process_request(self, request):
request.start_time = time.time()
def process_response(self, request, response):
duration = time.time() - getattr(request, 'start_time', time.time())
response["X-Response-Time"] = f"{duration:.4f}s"
return response
Key Notes:
- This middleware records the start time in
process_request
. - In
process_response
, it calculates the elapsed time, storing it in a custom response header.
3.2 Integrating Middleware in Django Settings
After creating your middleware, add it to the MIDDLEWARE
list in settings.py
:
MIDDLEWARE = [
# ...
'myapp.middleware.RequestTimingMiddleware',
]
Ensure you place it in the correct order relative to other middleware (e.g., before or after authentication-related middleware, depending on your use case).
4. Refining and Improving Middleware
Cloving’s strength lies in its iterative capabilities. Once the boilerplate is in place, you can refine or extend it in real time.
4.1 Adding Logging Details
For instance, you might want to log request method and endpoint along with response times. Simply instruct Cloving to revise the code:
Add logging to capture request method and endpoint along with response times
Cloving might update process_request
or process_response
to print or log additional details:
import logging
logger = logging.getLogger(__name__)
class RequestTimingMiddleware(MiddlewareMixin):
def process_request(self, request):
request.start_time = time.time()
def process_response(self, request, response):
duration = time.time() - getattr(request, 'start_time', time.time())
logger.info(
f"{request.method} {request.path} took {duration:.4f}s"
)
response["X-Response-Time"] = f"{duration:.4f}s"
return response
5. Testing Django Middleware Automatically
Robust testing is crucial for middleware, ensuring it behaves correctly in real-world scenarios.
5.1 Example: Unit Test Generation
To create unit tests for your newly generated or revised middleware, run:
cloving generate unit-tests -f middleware.py
Sample Output:
# test_middleware.py
from django.test import TestCase, RequestFactory
from django.http import HttpResponse
from .middleware import RequestTimingMiddleware
class MiddlewareTest(TestCase):
def setUp(self):
self.factory = RequestFactory()
self.middleware = RequestTimingMiddleware()
def test_request_logging(self):
# Simulate a request
request = self.factory.get('/')
response = HttpResponse()
# Pass request through middleware
self.middleware.process_request(request)
response = self.middleware.process_response(request, response)
# Check if 'X-Response-Time' header was set
self.assertIn('X-Response-Time', response)
self.assertTrue(response['X-Response-Time'].endswith('s'))
Here, Cloving’s generated test ensures the middleware adds the X-Response-Time
header. You can expand these tests to verify logging or extended functionality (e.g., error handling, requests to different endpoints, etc.).
6. Using Cloving Chat for Advanced Enhancements
Some tasks require more contextual guidance or deeper conversation, such as debugging complex performance issues or designing multi-step logic in your middleware. With Cloving’s chat mode, you can iterate on the code or ask best-practice questions:
cloving chat -f middleware.py
In this interactive environment, you can:
- Request performance improvements
- Ask for advanced logging or integration with external monitoring services
- Seek advice on ordering constraints among multiple Django middleware classes
7. Automating Git Commit Messages
After refining your middleware and verifying its functionality, commit your changes with Cloving’s help:
cloving commit
Cloving examines staged changes and proposes a descriptive commit message, for example:
Add request timing middleware to log response times and request info
You can accept or modify this message before finalizing the commit.
8. Best Practices for Cloving + Django
-
Initiate Early
Usecloving init
at the start of your project to maximize context awareness and get relevant suggestions. -
Granular Prompts
When generating or revising middleware, provide specific instructions (e.g., “Add IP address logging,” “Return error responses if time exceeds threshold”). -
Leverage Chat
Complex refactoring or advanced features often benefit from an interactive approach. Chat mode fosters a conversation that leads to optimal solutions. -
Test Thoroughly
Always generate or extend unit tests for your middleware. This ensures new logic remains stable and doesn’t interfere with other parts of your Django app. -
Stay Organized
Keep your middleware in dedicated files or modules, likemyapp/middleware/
. Cloving can then easily locate them and produce context-sensitive code. -
Review Logging Levels
If you add logging to your middleware, ensure you use the appropriate logging level (info, warning, error) and that your production environment handles logs responsibly.
9. Conclusion
Building robust Django middleware doesn’t have to be daunting. With Cloving CLI, you can:
- Generate essential middleware features
- Refine logic interactively
- Automate testing
- Receive AI-driven commit messages and code reviews
By integrating Cloving, you offload repetitive tasks to an AI co-pilot, freeing yourself to focus on design, performance optimization, and overall application strategy.
Remember: Cloving is here to augment—not replace—your expertise. Combining your domain knowledge with AI-driven code generation fosters a more efficient workflow, higher code quality, and ultimately, faster and better Django development.
Happy coding with Cloving and Django!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.