Building Reactive Java Systems with GPT's Guidance in Code Development
Updated on April 21, 2025

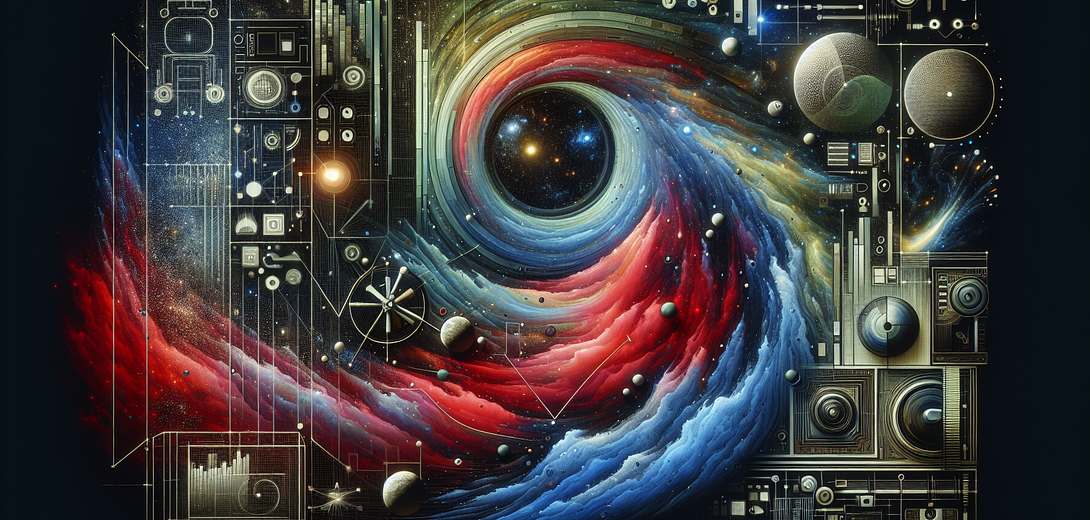
Incorporating AI into the software development life cycle is transformative, offering developers a sophisticated ally for building reactive systems. The Cloving CLI tool leverages the power of AI to help developers write efficient and high-quality code, perfect for crafting reactive Java systems. This guide will show you how to harness Cloving for Java development, optimizing your workflow and code quality significantly.
Getting Started with Cloving CLI
Cloving is a revolutionary command-line tool that acts as an AI-powered assistant, making development more efficient and rewarding. Before diving into building Java reactive systems, ensure Cloving is properly installed and configured on your system.
Installation
First, install the Cloving CLI tool globally via npm:
npm install -g cloving@latest
Configuration
Next, configure Cloving to use your favorite AI models:
cloving config
Follow the on-screen instructions to set up your API key and choose the AI model that best suits your needs. Proper setup ensures seamless integration as you use Cloving in your Java projects.
Setting Up Your Java Project with Cloving
Begin by initializing your Java project for Cloving:
cloving init
This command will analyze your project and create a cloving.json
file, storing metadata essential for context-aware code generation and assistance.
Generating Java Code
One of the most powerful features of Cloving is its ability to generate code snippets based on specific prompts, aiding in developing reactive Java systems.
Example: Creating Reactive Streams
Consider you want to create a reactive stream for processing data. Use the following Cloving command:
cloving generate code --prompt "Construct a reactive stream for processing order data in Java" --files src/main/java/com/example/reactive/OrderProcessor.java --model <your-model>
Cloving will generate a Java class leveraging the Project Reactor or RxJava library:
package com.example.reactive;
import reactor.core.publisher.Flux;
public class OrderProcessor {
public static void main(String[] args) {
Flux<String> orderStream = Flux.just("Order1", "Order2", "Order3")
.map(order -> "Processed " + order);
orderStream.subscribe(System.out::println);
}
}
This snippet shows a basic reactive processing of an order stream, illustrating Cloving’s capability to understand and assist with reactive programming patterns.
Enhance Development with AI Chat
For ongoing development and complex algorithm implementations, the Cloving chat feature offers a dynamic AI-assisted coding session.
Chat Example: Improving a Java Service
Start a Cloving chat session to enhance a Java service:
cloving chat -f src/main/java/com/example/service/OrderService.java
In this interactive session, ask for code refactoring or optimization suggestions. Use special commands to integrate changes directly into your codebase:
Review the current order processing logic and suggest improvements for handling concurrency.
Cloving’s AI will provide insights and code recommendations, improving your service architecture effectively.
Automating Test Generation
Cloving can also generate unit tests, ensuring code reliability and robustness in Java applications.
Example: Generating Tests for the OrderProcessor
Generate JUnit tests to validate the OrderProcessor
logic:
cloving generate unit-tests -f src/main/java/com/example/reactive/OrderProcessor.java
Cloving will output JUnit test skeletons tailored for your class:
package com.example.reactive;
import org.junit.jupiter.api.Test;
import reactor.core.publisher.Flux;
import reactor.test.StepVerifier;
class OrderProcessorTest {
@Test
void shouldProcessOrders() {
Flux<String> orderStream = Flux.just("Order1", "Order2", "Order3")
.map(order -> "Processed " + order);
StepVerifier.create(orderStream)
.expectNext("Processed Order1", "Processed Order2", "Processed Order3")
.verifyComplete();
}
}
This auto-generated test ensures your processing logic works as expected.
Conclusion
Utilizing Cloving CLI for constructing reactive Java systems exemplifies the fusion of AI and development, bringing efficiency and intelligence to your workflow. Whether generating code, refining algorithms, or producing tests, Cloving is an indispensable tool for modern Java development.
Remember, Cloving assists you, enhancing and complementing your coding expertise to create stunning and efficient Java systems. Embrace AI-powered development, and transform how you build software.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.