Building Reactive and Scalable Vuex Stores with GPT's Help
Updated on March 05, 2025

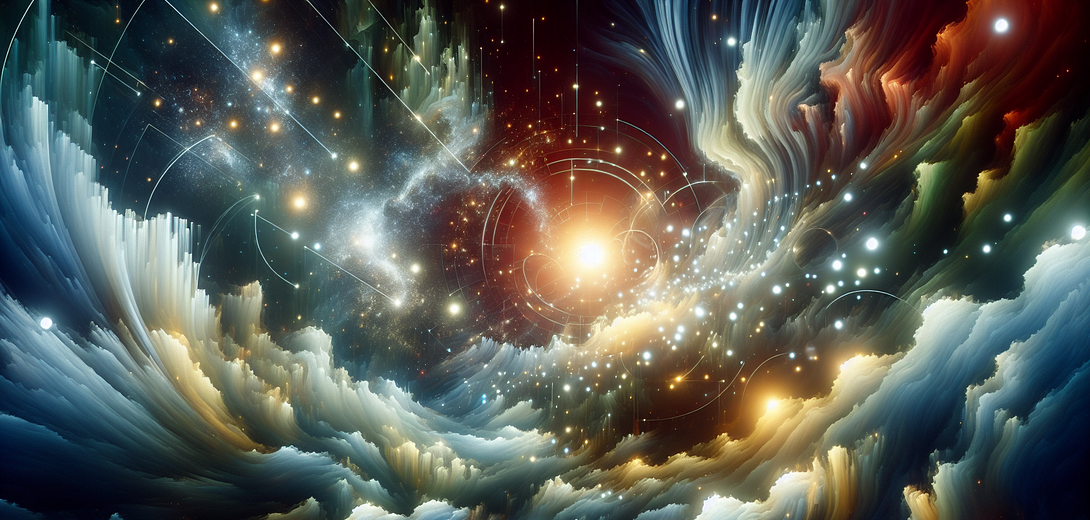
Supercharging Vuex State Management with Cloving CLI
As your Vue.js application grows, Vuex provides a robust and predictable way to manage state across components. Even so, setting up modules, actions, and mutations for a large codebase can be time-consuming. Cloving CLI – an AI-empowered command-line interface – reduces manual coding effort by automating code generation, testing, and more. In this post, we explore how to build effective and scalable Vuex stores using Cloving’s advanced capabilities.
1. Introduction to Cloving CLI
Cloving is a command-line tool that integrates AI (e.g., GPT models) into your developer workflow to:
- Generate code for new features or refactors
- Provide real-time assistance via an interactive chat
- Create unit tests automatically
- Review code for possible improvements or security issues
- Suggest meaningful commit messages
By understanding your project context, Cloving helps ensure that generated code aligns with your existing structure and standards.
2. Setting Up Your Environment with Cloving
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
Set up Cloving with your API key and preferred AI model:
cloving config
Follow the interactive steps to finalize your environment. This ensures Cloving is ready to generate relevant code customized to your project.
2.3 Initializing Your Project
Navigate to your Vue.js app folder and initialize Cloving so it can analyze your codebase:
cd my-vue-app
cloving init
Cloving scans your directory and creates a cloving.json
file, storing essential metadata about your project to improve the AI’s code generation accuracy.
3. Building a Vuex Store with Cloving
With Cloving configured, you can begin creating or enhancing Vuex modules in your application rapidly.
3.1 Example: Creating a Store Module
Suppose you have an e-commerce app that needs a new Vuex module for products. You can generate it as follows:
cloving generate code --prompt "Create a Vuex store module for handling products in an ecommerce app" -f store/modules/products.js
Sample Output:
// store/modules/products.js
export const state = () => ({
products: [],
selectedProduct: null,
});
export const getters = {
allProducts: (state) => state.products,
productCount: (state) => state.products.length,
};
export const mutations = {
SET_PRODUCTS(state, products) {
state.products = products;
},
SET_SELECTED_PRODUCT(state, product) {
state.selectedProduct = product;
},
};
export const actions = {
fetchProducts({ commit }) {
// Placeholder: fetch from an API
const products = [];
commit('SET_PRODUCTS', products);
},
selectProduct({ commit }, product) {
commit('SET_SELECTED_PRODUCT', product);
},
};
Notes:
- Cloving references your existing code structure (if available) for naming conventions or patterns.
- Replace placeholders (like
[]
) with real API calls or local storage logic.
3.2 Integrating the Module
In your main store file (e.g., store/index.js
), register the products
module:
import Vue from 'vue';
import Vuex from 'vuex';
import * as products from './modules/products';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
products,
},
});
4. Generating Unit Tests for Your Vuex Store
Testing ensures your Vuex module behaves correctly, especially as your application scales. Cloving can scaffold essential unit tests quickly:
cloving generate unit-tests -f store/modules/products.js
Sample Output:
// store/modules/products.test.js
import { state, mutations } from './products';
const { SET_PRODUCTS, SET_SELECTED_PRODUCT } = mutations;
describe('Products Module Mutations', () => {
it('should set products', () => {
const localState = { products: [] };
const productsMock = [{ id: 1, name: 'Product A' }];
SET_PRODUCTS(localState, productsMock);
expect(localState.products).toEqual(productsMock);
});
it('should set selected product', () => {
const localState = { selectedProduct: null };
const productMock = { id: 1, name: 'Product A' };
SET_SELECTED_PRODUCT(localState, productMock);
expect(localState.selectedProduct).toEqual(productMock);
});
});
Notes:
- Cloving’s generated tests cover the core behavior for your mutations or actions.
- Expand the coverage by adding negative tests, edge cases, or asynchronous test scenarios.
5. Enhancing Code with Cloving Chat
For more complex logic or iterative improvements, Cloving’s chat provides real-time AI-assisted guidance:
cloving chat -f store/modules/products.js
Ask questions like:
cloving> How can I handle errors in fetchProducts when the API fails?
Cloving might respond with a snippet that includes error handling logic in your fetchProducts
action.
6. Generating Meaningful Commit Messages
Maintain clarity in your version control history by letting Cloving propose commit messages:
cloving commit
Cloving reviews staged changes and offers a concise, context-relevant commit message. For instance:
Add products Vuex module with basic tests for inventory management
You can accept or modify the suggestion before finalizing your commit.
7. Best Practices for Using Cloving with Vuex
-
Maintain Clear Module Structure
Keep your Vuex modules organized (e.g., separatestate.js
,mutations.js
,actions.js
, etc.) so Cloving can better parse and integrate new code. -
Iterative Development
Generate or update modules in small increments, validating each step with unit tests and interactive chat sessions. -
Refine Generated Code
AI-generated code is a starting point—apply your domain logic, error handling, or naming conventions for a polished final result. -
Leverage Official Vue/CLI Tools
Combine Cloving with the official Vue CLI,vuex-map-fields
, or other community libraries for best practices in large-scale Vue apps. -
Test Thoroughly
While Cloving can generate baseline tests, you should expand them to cover edge cases, negative paths, and performance scenarios.
8. Example Workflow with Cloving and Vuex
Below is an outline of how you might integrate Cloving into your day-to-day Vuex work:
- Initialize:
cloving init
in your project root. - Create Module:
cloving generate code --prompt "Create a users Vuex module" -f store/modules/users.js
- Test:
cloving generate unit-tests -f store/modules/users.js
for initial coverage. - Iterate: Use Cloving chat to refine or enhance logic.
- Review: Optionally run
cloving generate review
to catch potential issues. - Commit: Summarize changes with
cloving commit
.
9. Conclusion
Cloving CLI transforms the way Vue.js developers handle Vuex state management by providing an AI-driven approach to code generation, testing, and iterative refinement. Whether you’re creating new modules or strengthening existing ones, Cloving can help you maintain scalable, reactive, and efficient stores with minimal manual effort.
Remember: Cloving is a supportive tool that complements your expertise, enabling you to focus on crafting robust application logic and user experiences. Give Cloving a try and discover how AI-augmented coding can elevate your Vuex store architecture and overall development workflow.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.