Building Performant Flask APIs with AI Insight and GPT
Updated on April 01, 2025

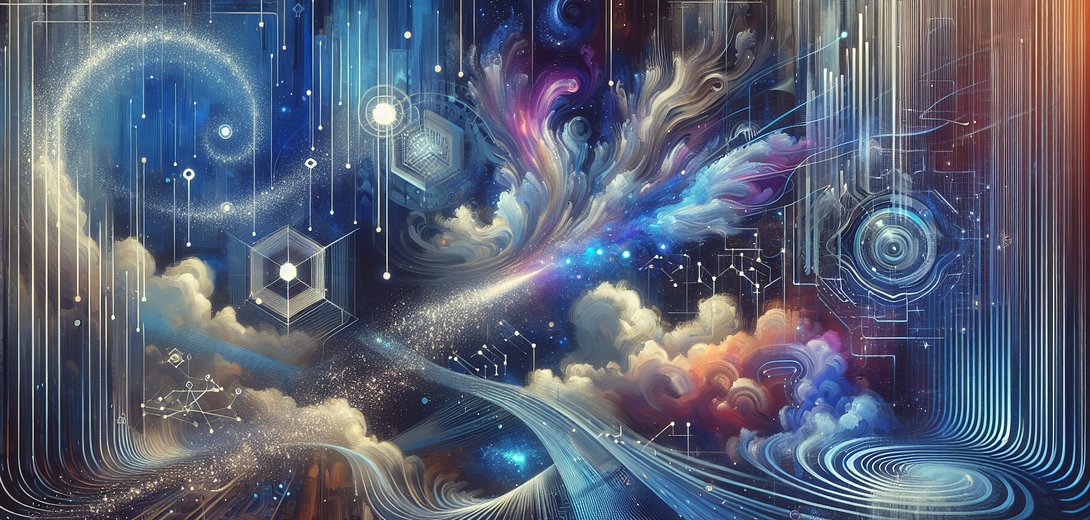
In the ever-evolving world of web development, creating efficient and performant APIs is essential for a smooth user experience and scalable applications. With the power of AI, you can enhance your Flask development process, making it more efficient and insightful. This blog post will guide you through using the Cloving CLI tool, integrated with AI, to build performant Flask APIs. By utilizing Cloving’s features and commands, you can significantly optimize your development workflow and code quality.
Understanding the Cloving CLI
Cloving is a command-line interface tool designed to integrate AI into your development workflow. It can act as an AI-powered assistant to help you generate code, improve performance, and maintain optimal code quality.
1. Setting Up Cloving for Flask Development
Before leveraging Cloving for your Flask APIs, let’s ensure it’s properly set up in your development environment.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving to use your preferred AI model by configuring it with your API key:
cloving config
Follow the interactive prompts to configure Cloving with your API key and model preferences.
2. Initializing Your Flask Project
To get Cloving up and running with your Flask project, initialize it in your project directory:
cloving init
This command prepares your project for Cloving by creating a cloving.json
file and loading necessary metadata about your project.
3. Generating API Endpoints with AI Assistance
Let’s explore how to generate Flask API endpoints using Cloving’s powerful AI capabilities.
Example:
Imagine you need to create an API endpoint to manage user data in your Flask application. You can use the cloving generate code
command:
cloving generate code --prompt "Create a Flask API endpoint to handle user data with CRUD operations" --files app/routes/user.py
Cloving will understand the context and generate a relevant Flask API endpoint, which might look something like this:
from flask import Flask, jsonify, request
app = Flask(__name__)
users = []
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(users)
@app.route('/users', methods=['POST'])
def add_user():
user = request.get_json()
users.append(user)
return jsonify(user), 201
@app.route('/users/<int:user_id>', methods=['PUT'])
def update_user(user_id):
user = request.get_json()
users[user_id] = user
return jsonify(user)
@app.route('/users/<int:user_id>', methods=['DELETE'])
def delete_user(user_id):
users.pop(user_id)
return '', 204
This generated code provides a functional API for performing CRUD operations on user data.
4. Optimizing Performance
Once you’ve generated your API endpoints, it’s crucial to ensure they perform optimally. Cloving can assist in reviewing and refining your code.
Example:
Suppose you want to optimize the performance of the user API endpoint. You can engage in a deeper discussion with Cloving:
cloving chat -f app/routes/user.py
In the Cloving chat session, you might type:
How can I optimize the performance of these user endpoints?
Cloving can provide insights and suggestions to improve the performance by recommending caching strategies, database optimizations, or identifying potential bottlenecks.
5. Reviewing and Refactoring Code
Keep your Flask application maintainable by periodically reviewing and refactoring your code. Cloving can assist with this through automated code reviews.
cloving generate review -f app/routes/user.py
This command prompts Cloving to generate an AI-powered code review, highlighting areas for improvement and offering suggestions for refactoring.
6. Enhancing Testing with AI-generated Unit Tests
To ensure robustness, generate unit tests for your Flask APIs with Cloving:
cloving generate unit-tests -f app/routes/user.py
An example test might look like:
import unittest
from app import app
class UserAPITestCase(unittest.TestCase):
def setUp(self):
self.client = app.test_client()
def test_get_users(self):
response = self.client.get('/users')
self.assertEqual(response.status_code, 200)
def test_add_user(self):
response = self.client.post('/users', json={'name': 'Alice'})
self.assertEqual(response.status_code, 201)
self.assertIn('Alice', response.get_json())
if __name__ == '__main__':
unittest.main()
These auto-generated tests form the baseline for comprehensive testing of your API functionality.
7. Efficient API Deployment Using Shell Scripts
Create deployment scripts for efficient API deployment:
cloving generate shell --prompt "Script to deploy Flask API to a production server"
Cloving could generate something like:
#!/bin/bash
echo "Deploying Flask API..."
# Activate virtual environment
source venv/bin/activate
# Run database migrations
flask db upgrade
# Start the Flask application
gunicorn app:app --workers 3 --bind 0.0.0.0:8000
echo "Deployment complete."
By automating deployment processes, you minimize the risk of human error and streamline your deployment workflow.
Conclusion
Incorporating the Cloving CLI into your Flask API development can dramatically enhance your productivity and efficiency. By leveraging AI insights, you can build, optimize, and maintain performant APIs seamlessly. Embrace Cloving to unlock the potential of AI in your development workflow and transform how you build web applications.
Remember, while Cloving is a powerful assistant, it complements rather than replaces your expertise. Use it to augment your capabilities and deliver high-quality, performant Flask APIs.
Additional Resources
For more comprehensive guidance and support, refer to the Cloving documentation and continue exploring the tutorials to deepen your understanding and mastery of this AI-powered tool.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.