Building Multi-Language Code Support in Java Applications with GPT
Updated on March 05, 2025

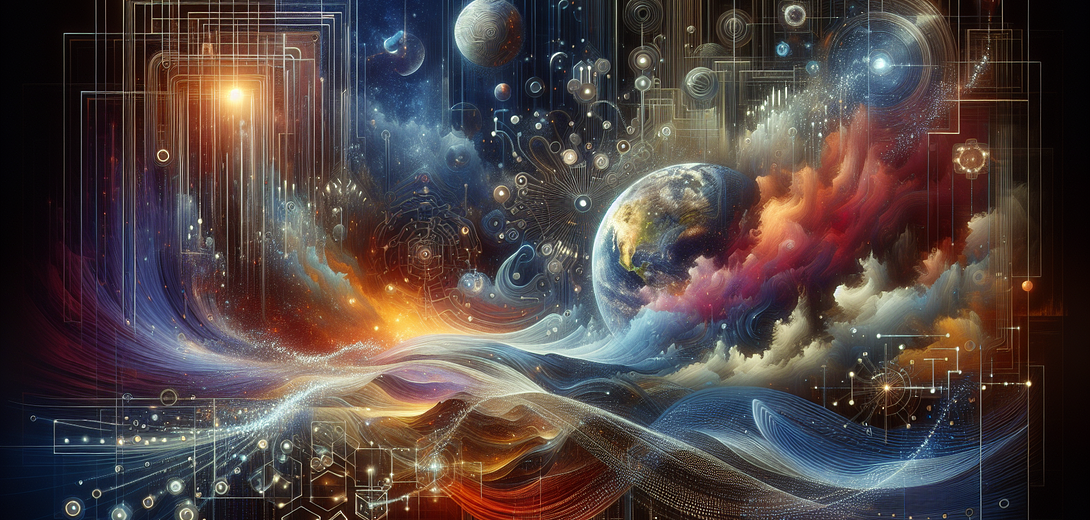
Streamlining Multi-Language Support in Java Applications with Cloving CLI
In today’s global development environment, it’s increasingly important for Java applications to handle multiple programming languages and internationalization (i18n) efficiently. Implementing these features can be complex, but tools like Cloving CLI simplify the process by automating code generation, providing real-time assistance, and ensuring high code quality. This guide explores how you can incorporate multi-language support into your Java applications using Cloving’s AI-powered capabilities.
1. Getting Started with Cloving CLI
1.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
1.2 Configuration
Next, configure Cloving to connect with your API key and select your AI model (e.g., GPT-3.5, GPT-4, etc.):
cloving config
Follow the on-screen prompts to finalize your setup. Once complete, Cloving can generate context-aware code tailored to your project’s needs.
1.3 Initializing Cloving in Your Project
To take full advantage of Cloving’s features, initialize it in your Java application’s root directory:
cloving init
This command analyzes your project structure and creates a cloving.json
file. Cloving uses this metadata to deliver accurate and project-specific AI suggestions.
2. Generating Multi-Language Support Code
Many Java applications need to execute multiple languages or handle internationalization. Cloving helps automate these tasks, whether you’re integrating something like GraalVM for polyglot scripting or implementing robust i18n.
2.1 Example: GraalVM Polyglot Support
Consider you want your Java application to run JavaScript and Python code side-by-side using GraalVM. You can prompt Cloving to generate a starter class:
cloving generate code --prompt "Set up multi-language support in a Java application using GraalVM" --files src/main/java/com/example/LanguageSupport.java
Sample Output:
// src/main/java/com/example/LanguageSupport.java
import org.graalvm.polyglot.*;
public class LanguageSupport {
public static void main(String[] args) {
// Create a context that can execute JavaScript and Python code
try (Context context = Context.newBuilder().allowAllAccess(true).build()) {
// Execute JavaScript code
context.eval("js", "console.log('Hello from JavaScript!');");
// Execute Python code
context.eval("python", "print('Hello from Python!')");
}
}
}
This boilerplate sets up a GraalVM context to run JavaScript and Python in a single Java application.
3. Reviewing and Refining Code
3.1 Interactive Code Generation
If you need to extend multi-language support further (e.g., adding Ruby or R), you can refine your code interactively:
cloving chat -f src/main/java/com/example/LanguageSupport.java
In the chat prompt, request enhancements:
cloving> Add support for Ruby in the LanguageSupport class
Cloving may update LanguageSupport.java
to include Ruby code execution, ensuring your application evolves quickly without repetitive coding.
4. Internationalization and Localization
For a truly global audience, internationalization (i18n) and localization (l10n) are critical. Cloving can automate the initial setup for common Java i18n approaches.
4.1 Generating I18n Code with ResourceBundles
Prompt Cloving to create or update a class that uses ResourceBundle
:
cloving generate code --prompt "Add internationalization using ResourceBundle for a Java application" --files src/main/java/com/example/LanguageSupport.java
Sample Output:
// src/main/java/com/example/LanguageSupport.java
import java.util.Locale;
import java.util.ResourceBundle;
public class LanguageSupport {
public static void main(String[] args) {
// Load the French resource bundle
ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", Locale.FRENCH);
// Print a greeting message from the resource bundle
String greeting = messages.getString("greeting");
System.out.println(greeting); // e.g., Bonjour le monde!
}
}
Notes:
- You’d store translations in properties files named
MessagesBundle_fr.properties
,MessagesBundle_en.properties
, etc. - Cloving can also add fallback logic or dynamic locale switching.
5. Implementing Unit Tests for Multi-Language Code
Testing is vital when multiple languages and locales are involved. Cloving helps generate test suites to ensure everything runs smoothly.
5.1 Example: Test Generation
cloving generate unit-tests -f src/main/java/com/example/LanguageSupport.java
Possible Test Output:
// src/test/java/com/example/LanguageSupportTest.java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
import org.graalvm.polyglot.Context;
import java.util.ResourceBundle;
import java.util.Locale;
public class LanguageSupportTest {
@Test
public void testGraalVMPolyglot() {
try (Context context = Context.newBuilder().allowAllAccess(true).build()) {
assertDoesNotThrow(() -> {
context.eval("js", "console.log('Testing JS')");
context.eval("python", "print('Testing Python')");
});
}
}
@Test
public void testResourceBundle() {
ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", Locale.FRENCH);
String greeting = messages.getString("greeting");
assertEquals("Bonjour le monde!", greeting);
}
}
6. Real-Time Assistance with Cloving Chat
Cloving’s chat mode is invaluable when you need a dynamic back-and-forth approach, such as:
- Debugging cross-language issues
- Designing advanced workflows (e.g., caching compiled scripts)
- Handling complex locale fallback logic
cloving chat -f src/main/java/com/example/LanguageSupport.java
Ask questions or refine your approach in real time.
7. Commit Messages with Context
After finalizing changes, let Cloving propose meaningful commit messages:
cloving commit
Cloving analyzes your diffs and offers a succinct, context-aware summary—helpful for teams maintaining a clear Git history.
8. Best Practices and Tips
- Keep Dependencies Updated
For multi-language execution via GraalVM, ensure you have the latest GraalVM version or relevant libraries. - Minimize Complexity
Only enable the languages and locale features you truly need—excess can bloat your app. - Use ResourceBundle Patterns
Keep translations in neatly organized.properties
files or adopt frameworks like Spring Boot which build on ResourceBundles. - Encapsulate Polyglot Logic
Create a dedicated service or utility class for multi-language code execution, so the rest of your application remains clean. - Test Thoroughly
Multi-language code can introduce additional failure points—test error handling, performance, and edge cases.
9. Conclusion
By using Cloving CLI, you can seamlessly incorporate multi-language support into your Java applications—be it running scripts via GraalVM or managing i18n with ResourceBundle
. Cloving’s AI-driven approach saves time, ensures consistency, and streamlines maintenance.
Remember: Cloving augments—rather than replaces—your expertise. Lean on it to handle repetitive or boilerplate tasks, then apply your domain knowledge to polish and refine. With Cloving’s flexible code generation, interactive chat, and automated test creation, you’re well on your way to building robust, globally adaptable Java applications.
Explore the possibilities by integrating Cloving into your daily workflow, and watch your productivity and code quality climb to new heights!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.