Building Modular NodeJS Codebase Using GPT Assistance
Updated on January 20, 2025

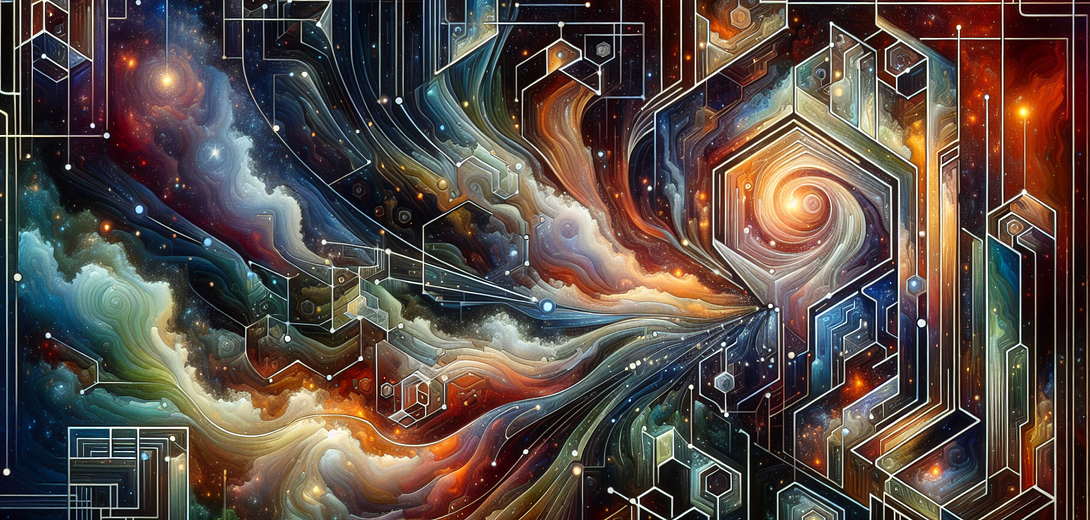
In the world of software development, creating modular and maintainable code is crucial for long-term success. The Cloving CLI tool, powered by advanced AI models like GPT, provides the perfect synergy between human ingenuity and artificial intelligence. Whether you are a seasoned developer or just starting in the NodeJS ecosystem, this blog post will guide you on building a robust modular NodeJS codebase with the help of Cloving CLI.
Getting Started
1. Setting Up Cloving CLI
Before we dive into building your NodeJS codebase, let’s ensure Cloving is set up properly.
Installation:
First, install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving with your API key and preferred models:
cloving config
Follow the interactive prompts to set up your AI model, API key, and preferences.
2. Initializing Your Project
Once Cloving is configured, set up your NodeJS project directory to leverage Cloving’s capabilities:
cloving init
This creates a cloving.json
file that holds metadata about your application context.
Building a Modular Codebase with Cloving
3. Generating Code for Reusable Modules
A modular codebase is often based on reusable modules. Let’s generate a NodeJS module using Cloving:
Example:
Suppose you need a utility module for handling JSON files.
cloving generate code --prompt "Create a NodeJS module to read and write JSON files in a directory" --files utils/jsonHandler.js
The generated code might look like:
// utils/jsonHandler.js
const fs = require('fs');
const path = require('path');
function readJSONSync(filePath) {
const fullPath = path.resolve(filePath);
const data = fs.readFileSync(fullPath, 'utf-8');
return JSON.parse(data);
}
function writeJSONSync(filePath, data) {
const fullPath = path.resolve(filePath);
fs.writeFileSync(fullPath, JSON.stringify(data, null, 2), 'utf-8');
}
module.exports = {
readJSONSync,
writeJSONSync,
};
4. Interactive Code Reviews
Keeping your code clean with regular reviews is part of maintaining a modular codebase. Use Cloving for AI-powered code reviews:
cloving generate review
Receive feedback on code quality, potential bugs, and improvements. Implement the suggested changes to keep your code modular.
5. Using Cloving Chat for Complex Requests
Integrate complex modules or solve deep-rooted bugs using Cloving’s interactive chat:
cloving chat -f handlers/requestHandler.js
Here you can ask Cloving for additional features or improvements, such as:
Add error handling to the requestHandler module.
6. Automating Test Creation
Automatically generate tests for your modules to ensure they are functioning correctly.
Example for the JSON handler module:
cloving generate unit-tests -f utils/jsonHandler.js
Generated tests might look like:
// utils/jsonHandler.test.js
const { readJSONSync, writeJSONSync } = require('./jsonHandler');
const fs = require('fs');
jest.mock('fs');
describe('JSON Handler', () => {
beforeEach(() => {
fs.readFileSync.mockClear();
fs.writeFileSync.mockClear();
});
test('readJSONSync reads JSON correctly', () => {
const mockData = '{"key": "value"}';
fs.readFileSync.mockReturnValue(mockData);
const data = readJSONSync('test.json');
expect(data).toEqual({ key: 'value' });
});
test('writeJSONSync writes JSON correctly', () => {
writeJSONSync('test.json', { key: 'value' });
expect(fs.writeFileSync).toHaveBeenCalledWith('test.json', JSON.stringify({ key: 'value' }, null, 2), 'utf-8');
});
});
7. Streamlining Git Commits
Maintain clear and descriptive commit histories by using Cloving for commits:
cloving commit
Review AI-generated commit messages that capture the essence of your code changes.
Conclusion
Integrating Cloving CLI into your NodeJS development process empowers you to build a cleaner, more modular codebase. Use AI assistance from Cloving to generate reusable code snippets, automate tedious tasks like test creation, and maintain code quality with interactive code reviews and chat.
Embrace Cloving’s capabilities to augment your NodeJS projects, seamlessly blending AI insights with your development expertise. For more tips and features, explore Cloving’s documentation and discover how this AI-powered tool can redefine your development process.
Remember, while Cloving enhances productivity, it is your expertise that gives the code its true value. Use Cloving to save time and ensure quality, allowing you to focus on the innovative aspects of your projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.