Building Full Stack Applications with NestJS and GPT
Updated on March 05, 2025

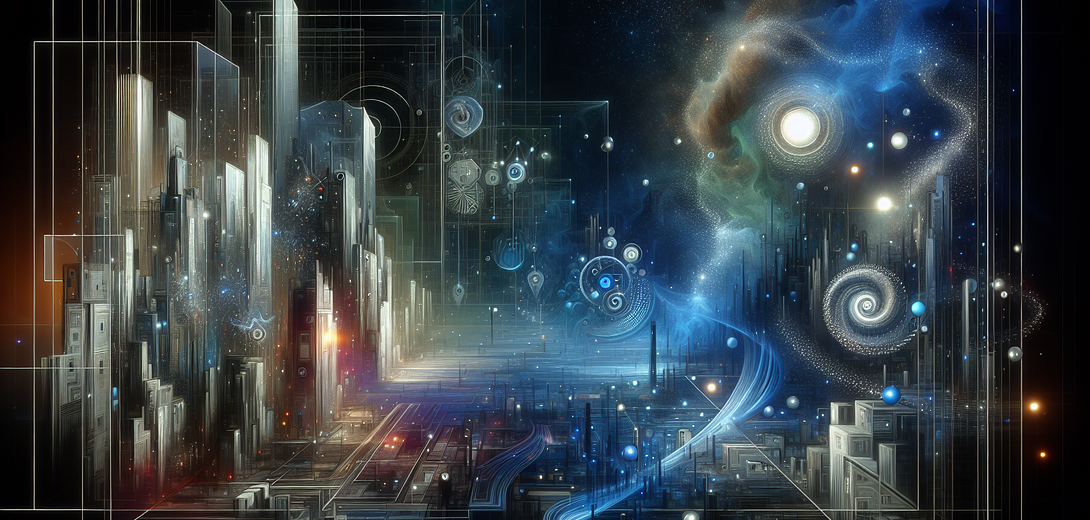
Supercharging Full Stack NestJS Development with Cloving CLI
In the modern development landscape, full stack applications have become the cornerstone for creating robust and dynamic web solutions. Integrating AI into this workflow can greatly enhance productivity, especially when using powerful tools like Cloving CLI with NestJS and GPT models. In this tutorial, we’ll explore how to build and enhance full stack applications using NestJS while leveraging Cloving CLI to generate high-quality code and insights.
1. Understanding the Cloving CLI
The Cloving CLI is an AI-powered command-line interface designed to streamline your coding experience. Leveraging GPT models, it enhances your workflow by generating code, unit tests, commit messages, and more—tailored to the specific context of your application.
Key Features of Cloving CLI:
- AI-Assisted Code Generation: Generate boilerplate and domain-specific code rapidly.
- Interactive Chat: Collaborate with GPT models directly in your terminal for real-time advice, debugging, or feature expansions.
- Automated Testing: Quickly scaffold unit tests to ensure your application remains robust.
- Commit Message Suggestions: Maintain clear and concise commit histories without repetitive manual effort.
2. Setting Up Cloving with NestJS
Before diving into building your application, confirm that both NestJS and Cloving are correctly installed.
2.1 Installation Steps
-
Install Cloving CLI globally:
npm install -g cloving@latest
-
Generate a NestJS Application (if you haven’t already):
npx @nestjs/cli new my-nestjs-app cd my-nestjs-app
This command scaffolds a standard NestJS project with a recommended file structure.
-
Configure Cloving within your NestJS project:
cloving init
Cloving creates a
cloving.json
file that captures metadata about your project. The more context Cloving has, the more accurate and relevant its AI-generated code will be.
3. Generating RESTful Components with Cloving
Full stack applications often begin with a RESTful API. Let’s use Cloving to bootstrap a simple NestJS service that handles CRUD operations for a “User” entity.
3.1 Example: Users Service
cloving generate code --prompt "Create a NestJS service for managing users with basic CRUD operations" --files src/users/users.service.ts
Cloving will generate something like the following snippet, which you can freely modify:
// src/users/users.service.ts
import { Injectable } from '@nestjs/common';
interface User {
id: number;
name: string;
}
interface UpdateUserDto {
name?: string;
}
@Injectable()
export class UsersService {
private users: User[] = [];
create(user: User): User {
this.users.push(user);
return user;
}
findAll(): User[] {
return this.users;
}
findOne(id: number): User | undefined {
return this.users.find(user => user.id === id);
}
update(id: number, updateUserDto: UpdateUserDto): User | undefined {
const user = this.findOne(id);
if (user) {
Object.assign(user, updateUserDto);
}
return user;
}
remove(id: number): void {
this.users = this.users.filter(user => user.id !== id);
}
}
You can further refine or expand this service using Cloving’s interactive chat feature or by generating additional files (e.g., a controller, DTOs, etc.).
4. Enhancing the Application with AI-Powered Chat
Building a modern NestJS application often involves complex architectural decisions—like authentication, authorization, logging, and performance considerations. Cloving’s interactive chat lets you explore these areas in a conversational manner with an AI assistant.
4.1 Example: Interactive Refinement
cloving chat -f src/users/users.controller.ts
In the interactive session, you might ask:
cloving> How can I extend the UsersService to include JWT-based authentication?
Cloving can guide you through adding the @nestjs/jwt package, implementing guard classes, or updating your AuthModule
for best practices—directly in the chat window. This approach saves you from searching for disparate tutorials and references, consolidating the guidance into one location.
5. Generating Unit Tests
Quality assurance is essential for a production-ready application. With Cloving, you can quickly spin up relevant unit tests for your NestJS components.
5.1 Example: Test Generation
cloving generate unit-tests -f src/users/users.service.ts
Cloving often creates a file like users.service.spec.ts
:
// src/users/users.service.spec.ts
import { Test, TestingModule } from '@nestjs/testing';
import { UsersService } from './users.service';
describe('UsersService', () => {
let service: UsersService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
providers: [UsersService],
}).compile();
service = module.get<UsersService>(UsersService);
});
it('should create a user', () => {
const user = service.create({ id: 1, name: 'John Doe' });
expect(service.findOne(1)).toEqual(user);
});
it('should remove a user', () => {
service.create({ id: 2, name: 'Jane Doe' });
service.remove(2);
expect(service.findOne(2)).toBeUndefined();
});
});
Feel free to iterate on these tests by requesting more scenarios, such as edge cases or negative test flows, via Cloving chat or additional prompts.
6. Beyond CRUD: Building a Full Stack Experience
Because NestJS is a full-fledged framework, you can easily incorporate layers like:
-
Authentication & Authorization:
- JWT (JSON Web Tokens) for stateless auth
- Passport strategies (e.g., Local, JWT, OAuth2)
-
Real-Time Communication:
- WebSockets with the built-in
@nestjs/websockets
- GraphQL subscriptions for reactive data
- WebSockets with the built-in
-
Front-End Integration:
- Connect your NestJS REST or GraphQL endpoints to popular front-end frameworks like React, Angular, or Vue.
- Cloving can also help scaffold or refine front-end code based on your existing project structure.
6.1 Example: Generating a Simple Auth Guard
cloving generate code --prompt "Create a NestJS AuthGuard that checks for a valid JWT in the request header" --files src/auth/jwt-auth.guard.ts
The AI-generated code might look like this, which you can then refine or expand:
// src/auth/jwt-auth.guard.ts
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
@Injectable()
export class JwtAuthGuard implements CanActivate {
constructor(private jwtService: JwtService) {}
canActivate(context: ExecutionContext): boolean {
const request = context.switchToHttp().getRequest();
const token = request.headers.authorization?.split(' ')[1];
if (!token) return false;
try {
const payload = this.jwtService.verify(token);
request.user = payload;
return true;
} catch (err) {
return false;
}
}
}
7. AI-Assisted Commit Messages
Maintaining a well-organized commit history is a best practice in any team or solo project. Cloving can help craft concise yet descriptive commit messages reflecting the changes you’ve made.
cloving commit
Cloving analyzes your staged files and suggests a commit message. You can accept, modify, or discard it. For example:
Implement basic CRUD operations for UsersService and add initial unit tests
8. Pro Tips for Maximizing Productivity
- Provide Clear Prompts: The more specific you are with your
cloving generate code
prompt, the more targeted the generated code becomes. - Iterate with Chat: Use the
cloving chat
command for deeper explorations or iterative refinements. Cloving remembers context from previous messages within that session. - Combine Tools: Pair Cloving with NestJS’s CLI commands (e.g.,
nest generate
) for a robust scaffolding strategy. Each tool has strengths that complement the other. - Stay Organized: Keep a well-structured file layout so Cloving can easily discover existing code and generate relevant snippets.
- Regularly Update: Both NestJS and Cloving evolve quickly. Updating ensures you have the latest features and security patches.
- Review AI Output: Always review, test, and refine AI-generated code. While Cloving is a powerful productivity booster, it doesn’t replace human judgment.
9. Conclusion
Integrating Cloving CLI with NestJS and GPT models in your development process can markedly enhance productivity and accuracy. By automating code generation, logic refinement, unit tests, and commit messages, you can focus on the creative and logic-driven aspects of your code rather than repetitive boilerplate.
Remember: Cloving complements (rather than replaces) your development expertise. Use it to accelerate your NestJS application-building experience, from concept to deployment.
Happy building! Take advantage of Cloving’s AI-assisted environment to deliver sophisticated, high-quality full stack applications faster and with greater confidence.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.