Building Efficient GraphQL Endpoints with GPT's Help
Updated on March 30, 2025

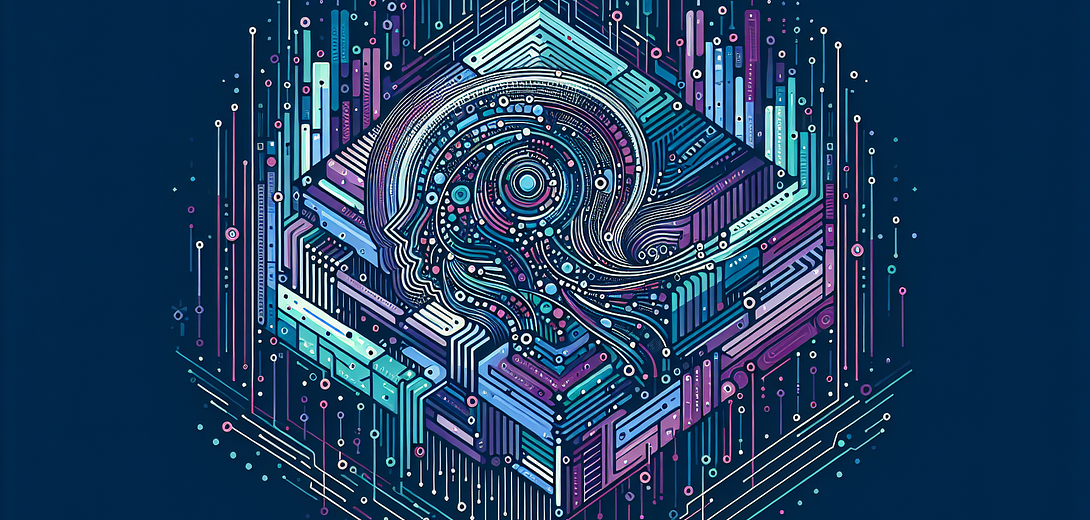
Integrating AI tools into development has transformed how we approach coding tasks, and using the Cloving CLI for building GraphQL endpoints is no exception. With its AI-driven insights, Cloving simplifies the process of creating efficient, scalable GraphQL APIs. In this tutorial, we’ll walk through practical steps and examples to harness the power of Cloving CLI for building GraphQL endpoints.
Getting Started with Cloving CLI
First, ensure Cloving is installed and set up in your development environment.
Installation
Begin by installing Cloving globally using npm:
npm install -g cloving@latest
Configuration
Configure Cloving to use your preferred AI model and API key:
cloving config
Follow the prompts to enter your API key and choose the models you’ll be working with.
Initializing Your Project
To provide Cloving with the context it needs about your project, run the init command in your project directory:
cloving init
This step creates a cloving.json
file with important metadata about your GraphQL project.
Generating GraphQL Endpoints
1. Using Code Generation for Endpoints
Cloving’s powerful AI can generate boilerplate code for your GraphQL endpoints, allowing you to focus on fine-tuning them.
Suppose you are working on a project that involves a user management system. You can use Cloving to create a basic user query:
cloving generate code --prompt "Create a GraphQL query for fetching user data" --files src/graphql/UserQuery.ts
The generated query could look like this:
// src/graphql/UserQuery.ts
import { gql } from 'apollo-server-express'
export const USER_QUERY = gql`
query getUser($id: ID!) {
user(id: $id) {
id
name
email
role
}
}
`
Cloving analyzes your project context to generate code that integrates seamlessly with your existing GraphQL setup.
2. Generating Unit Tests for GraphQL
To maintain the quality of your endpoints, it’s crucial to have unit tests in place. Cloving can assist in generating these tests, ensuring your queries and mutations work as expected:
cloving generate unit-tests -f src/graphql/UserQuery.ts
An example test for the UserQuery
might be:
// src/graphql/UserQuery.test.ts
import { USER_QUERY } from './UserQuery'
import { graphql } from 'graphql'
import schema from '../schema'
describe('User Query', () => {
it('fetches user data', async () => {
const result = await graphql(schema, USER_QUERY, null, null, { id: '123' })
expect(result.data.user).toHaveProperty('id', '123')
expect(result.data.user).toHaveProperty('name')
expect(result.errors).toBeUndefined()
})
})
3. Using Interactive Chat for Complex Schema Modifications
If you encounter a complex task or need further insights while building your GraphQL API, the Cloving chat feature is invaluable:
cloving chat -f src/graphql/UserQuery.ts
This launches an interactive session with the AI, where you can request further enhancements or explanations:
cloving> Add a mutation to update user email
Cloving might suggest an update like this:
// src/graphql/UpdateUserEmailMutation.ts
import { gql } from 'apollo-server-express'
export const UPDATE_USER_EMAIL_MUTATION = gql`
mutation updateUserEmail($id: ID!, $email: String!) {
updateUserEmail(id: $id, email: $email) {
success
message
user {
id
email
}
}
}
`
Best Practices for Using Cloving with GraphQL
-
Initial Setup: Always run
cloving init
to ensure it understands your project’s context and environment. -
Model Selection: Choose the right AI model according to your project’s complexity and requirement using
cloving config
. -
Interactive Development: Use
cloving chat
for hands-on assistance, especially helpful for developers new to GraphQL. -
Code Quality: Regularly run
generate unit-tests
to catch potential issues early. -
Commit Messages: Enhance your commit history with meaningful messages using
cloving commit
.
By leveraging Cloving CLI, you’re integrating a powerful AI assistant into your GraphQL development workflow, significantly boosting productivity and code quality. Whether you’re a novice or an experienced developer, Cloving offers the tools to create efficient and scalable GraphQL APIs seamlessly.
Embrace Cloving and see how it transforms your approach to coding GraphQL endpoints, making development not just efficient but also an engaging process!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.