Building Django Views with the Assistance of GPT
Updated on January 07, 2025

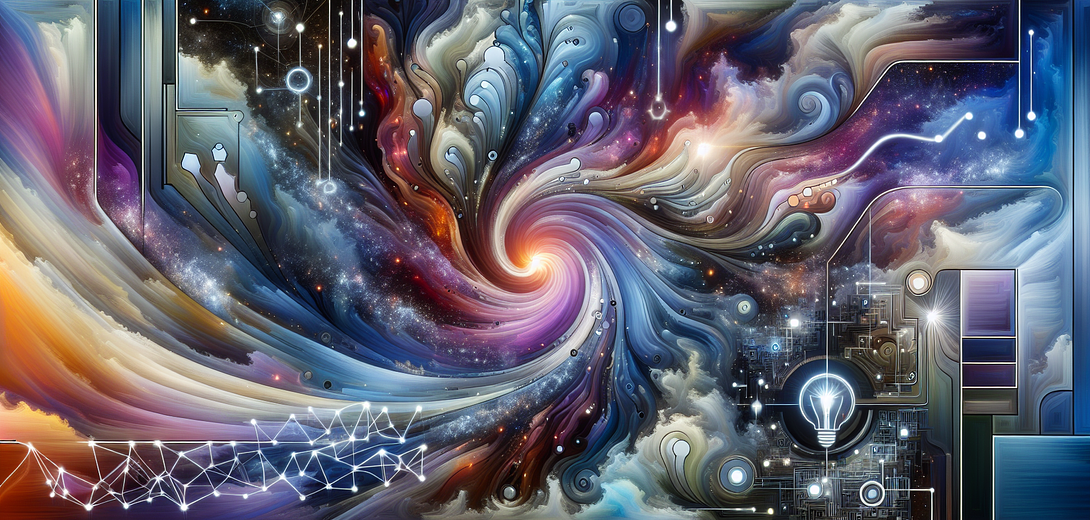
As developers, we often strive for ways to streamline our workflow and increase productivity. The Cloving CLI tool is one such innovation, integrating artificial intelligence into your development process. Today, we’re diving into how you can leverage the power of Cloving CLI to efficiently build Django views with support from AI. Through practical demonstrations, we’ll explore how this tool can enhance your Django development experience.
1. Get Started with Cloving
Before getting started with building Django views, ensure Cloving is correctly installed and set up in your development environment.
Installation and Configuration:
Install Cloving globally:
npm install -g cloving@latest
Configure Cloving:
cloving config
Follow the prompts to set up your API key, choose models, and adjust preferences.
Initialize Cloving in Your Project:
cloving init
This prepares your current directory, capturing the necessary context about your project in a cloving.json
file.
2. Generating Django Views
Let’s utilize Cloving to create a Django view for displaying a list of books. This view will retrieve data from the database and render it using a template.
Example: Generate a Django View
Use the cloving generate code
command to create the Django view:
cloving generate code --prompt "Create a Django view for displaying a list of books with pagination" -f app/views.py
Generated Code:
from django.shortcuts import render
from django.core.paginator import Paginator
from app.models import Book
def book_list(request):
book_objects = Book.objects.all()
paginator = Paginator(book_objects, 10) # 10 books per page
page_number = request.GET.get('page')
page_obj = paginator.get_page(page_number)
return render(request, 'app/book_list.html', {'page_obj': page_obj})
3. Review and Enhance the Generated Code
Incorporate Cloving to review and improve your Django views further. The AI can help suggest enhancements like adding filtering options or error handling.
Revise the View for Additional Features:
Revise the view to include filtering by author and error handling for pagination
Revised Code Example:
from django.db.models import Q
from django.core.paginator import EmptyPage, PageNotAnInteger
def book_list(request):
query = request.GET.get('q')
books = Book.objects.all()
if query:
books = books.filter(Q(title__icontains=query) | Q(author__icontains=query))
paginator = Paginator(books, 10)
page_number = request.GET.get('page')
try:
page_obj = paginator.page(page_number)
except PageNotAnInteger:
page_obj = paginator.page(1)
except EmptyPage:
page_obj = paginator.page(paginator.num_pages)
return render(request, 'app/book_list.html', {'page_obj': page_obj})
4. Generate Unit Tests for Your Views
Testing your Django views is essential for reliability. Cloving can assist in generating unit tests to streamline this process.
Generate Tests:
cloving generate unit-tests -f app/tests/test_views.py
Unit Tests Example:
from django.test import TestCase
from django.urls import reverse
from app.models import Book
class BookListViewTests(TestCase):
@classmethod
def setUpTestData(cls):
Book.objects.create(title='Book 1', author='Author A')
Book.objects.create(title='Book 2', author='Author B')
def test_view_url_exists_at_desired_location(self):
response = self.client.get('/books/')
self.assertEqual(response.status_code, 200)
def test_view_uses_correct_template(self):
response = self.client.get(reverse('book_list'))
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, 'app/book_list.html')
def test_pagination_is_ten(self):
response = self.client.get(reverse('book_list'))
self.assertTrue('is_paginated' in response.context)
self.assertTrue(response.context['is_paginated'] is True)
self.assertEqual(len(response.context['book_list']), 2)
5. Utilize Cloving Chat for Complex Queries
For additional customization or assistance, start a Cloving chat session. This feature acts as your AI pair programmer for more complex inquiries.
cloving chat -f app/views.py
In this interactive session, you can:
- Obtain code snippets
- Request explanations
- Retrieve guidance on Django best practices
6. Efficient Git Commits with Cloving
Furthermore, enhance your commit messages by leveraging the AI-powered insights of Cloving:
cloving commit
Cloving examines your changes and provides you with a well-structured commit message which you can edit before committing to git.
Conclusion
By integrating Cloving CLI into your Django development workflow, you can boost not only your productivity but also the quality of your code. This tool provides a powerful AI assistant, whether you’re generating views, writing unit tests, or refining existing code. The combination of Django’s robust stack with the AI-enhanced capabilities of Cloving creates a formidable set that’ll help you tackle your programming tasks efficiently.
Embrace Cloving in your projects and experience a significant enhancement in your development process, as machine intelligence becomes an integral part of your coding journey.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.