Building Custom Web Components with Stencil and GPT
Updated on April 01, 2025

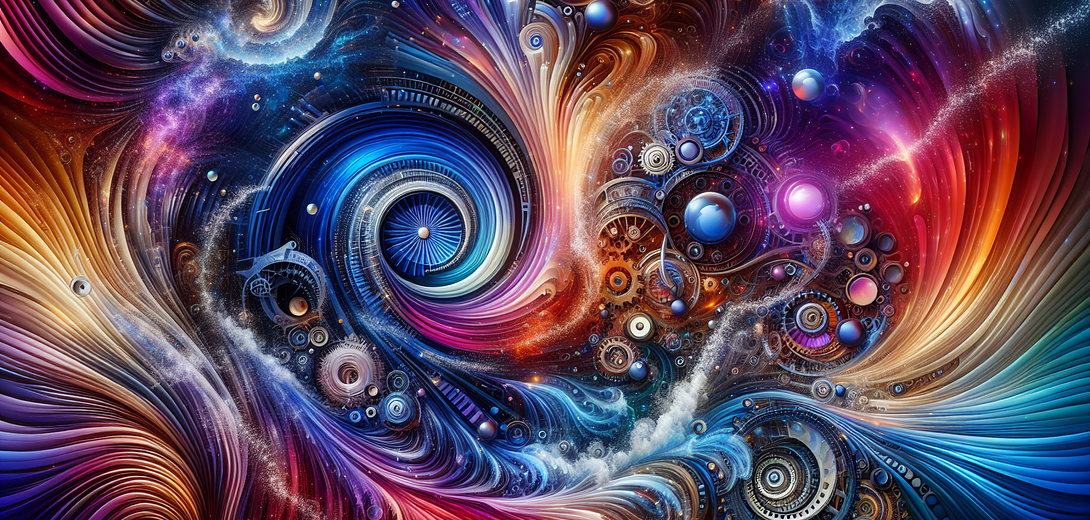
In the world of modern web development, building efficient and reusable components is crucial. Stencil, a web component compiler, facilitates building standards-compliant components that work seamlessly across different frameworks. When paired with the Cloving CLI, powered by GPT (Generative Pre-trained Transformer), developers can enhance productivity and code quality. In this tutorial, we will guide you through building custom web components using Stencil and Cloving CLI.
Introduction to Stencil and Cloving
Stencil is an open-source project that compiles Web Components (built on top of standard HTML, CSS, and JavaScript) to produce highly optimized and compatible UI parts. Combined with Cloving, a powerful AI-powered CLI tool, you can harness AI capabilities to generate code, conduct reviews, and even chat with an AI pair programmer to polish your Stencil components.
Step 1: Setting Up Your Environment
Before diving into component creation, ensure you have Node.js, npm, and Cloving CLI installed.
npm install -g @stencil/core cloving@latest
Configure Cloving to work with your AI model:
cloving config
Proceed with the interactive setup to select desired models and enter your API key.
Step 2: Initialize Your Project with Stencil
Create a new Stencil project:
npm init stencil
Choose the appropriate template for your project setup. Now, navigate into your project directory and initialize Cloving:
cd your-stencil-project
cloving init
This command will analyze your environment, and ensure Cloving has the necessary setup in your cloving.json
.
Step 3: Building a Custom Web Component
Let’s create a new component using Stencil. Assume you want to build a <my-todo-list>
component.
npm run generate component my-todo-list
This command creates a new component template typically found in src/components/my-todo-list/
.
Step 4: Using Cloving to Enhance Code Generation and Development
With Cloving, you can generate comprehensive feature implementations or even unit tests. Suppose you want to generate the code for rendering a todo list with an input field to add new tasks:
cloving generate code --prompt "Develop a Stencil component for a Todo list with input and list display" --files src/components/my-todo-list/my-todo-list.tsx
This might generate a snippet like this:
@Component({
tag: 'my-todo-list',
styleUrl: 'my-todo-list.css',
shadow: true,
})
export class MyTodoList {
@State() todos: string[] = [];
@State() newTodo: string = '';
handleInputChange(event: Event) {
this.newTodo = (event.target as HTMLInputElement).value;
}
addTodo() {
if (this.newTodo.trim()) {
this.todos = [...this.todos, this.newTodo];
this.newTodo = '';
}
}
render() {
return (
<div>
<input
type="text"
value={this.newTodo}
onInput={(event) => this.handleInputChange(event)}
/>
<button onClick={() => this.addTodo()}>Add Todo</button>
<ul>
{this.todos.map(todo => <li>{todo}</li>)}
</ul>
</div>
);
}
}
Step 5: Improving with Interactive Chat
For complex logic or additional features, use Cloving’s chat functionality for assistance:
cloving chat -f src/components/my-todo-list/my-todo-list.tsx
Interact with the AI to refine the logic, ask for suggestions, or even seek explanations about your existing code.
Step 6: Generating Unit Tests
Ensure robustness by generating unit tests with Cloving:
cloving generate unit-tests -f src/components/my-todo-list/my-todo-list.tsx
You might get unit tests such as:
import { newSpecPage } from '@stencil/core/testing';
import { MyTodoList } from './my-todo-list';
describe('my-todo-list', () => {
it('should add a todo when typed and button clicked', async () => {
const page = await newSpecPage({
components: [MyTodoList],
html: '<my-todo-list></my-todo-list>',
});
const input = page.root.querySelector('input');
const button = page.root.querySelector('button');
input.value = 'New Task';
input.dispatchEvent(new Event('input'));
button.click();
await page.waitForChanges();
const listItems = page.root.querySelectorAll('li');
expect(listItems).toHaveLength(1);
expect(listItems[0].textContent).toEqual('New Task');
});
});
Conclusion
By leveraging Stencil with the AI-powered Cloving CLI, developers can significantly streamline their workflow, boost productivity, and ensure high-quality code generation. Whether you need to create new components, improve existing logic, or automate testing, the seamless integration of AI into your development toolkit can enhance your capabilities and simplify the process.
Begin your journey with Stencil and Cloving and transform how you build modern web applications. Embrace the power of AI to revolutionize your development experience today!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.