Building Custom Data Visualization Components in D3.js with GPT
Updated on March 05, 2025

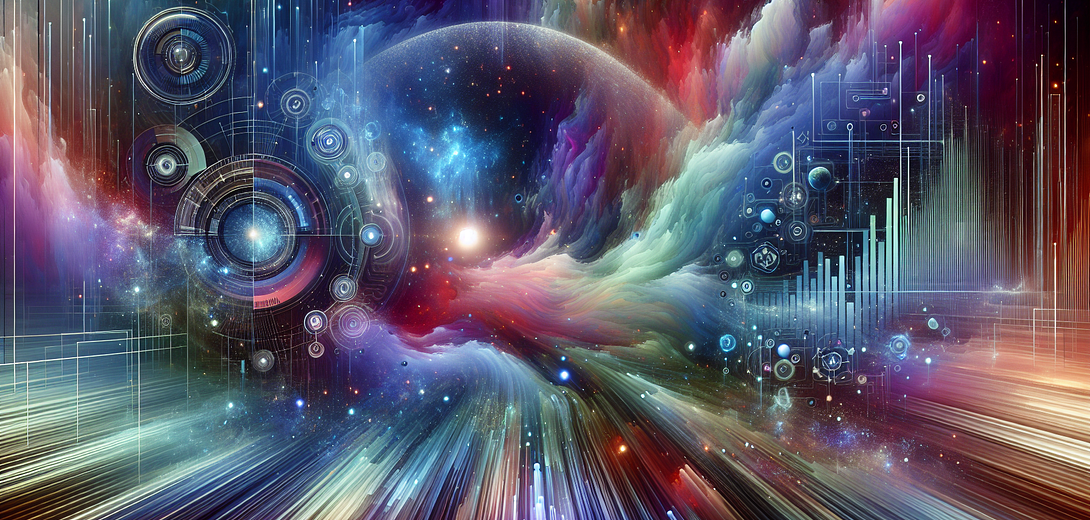
Supercharging Your D3.js Data Visualizations with Cloving CLI
Incorporating data visualization into your projects can significantly elevate the way you present information. With the power of D3.js and the Cloving CLI tool, you can efficiently create custom data visualization components by leveraging AI assistance. In this tutorial, we’ll explore how to harness the capabilities of Cloving CLI to streamline the development of stunning data visualizations in D3.js.
Getting Started with Cloving CLI and D3.js
Before diving into building components, ensure you have the Cloving CLI tool set up and ready to assist you in generating code for D3.js visualizations.
1. Setting Up Cloving
Begin by installing Cloving globally to integrate it into your workflow:
npm install -g cloving@latest
Configure the Cloving CLI to utilize the desired AI model:
cloving config
Follow the interactive setup to input your API key and select the appropriate models.
2. Initial Project Setup
Navigate to your project directory and initialize Cloving:
cloving init
This will allow the tool to understand the context of your project and aid in generating D3.js-specific visualization code.
3. Generating Your First D3.js Component
Let’s start with a simple example of creating a bar chart. Suppose you’re aiming to display quarterly sales data.
cloving generate code --prompt "Create a D3.js bar chart displaying sales data" --files src/components/BarChart.js
The command analyzes the context and generates a starting point for your bar chart. Below is a sample snippet Cloving might produce:
// src/components/BarChart.js
import * as d3 from 'd3';
const data = [
{ label: 'Q1', sales: 150 },
{ label: 'Q2', sales: 200 },
{ label: 'Q3', sales: 250 },
{ label: 'Q4', sales: 300 }
];
const BarChart = () => {
const width = 500;
const height = 300;
const margin = { top: 20, right: 30, bottom: 40, left: 40 };
const x = d3.scaleBand()
.domain(data.map(d => d.label))
.range([margin.left, width - margin.right])
.padding(0.1);
const y = d3.scaleLinear()
.domain([0, d3.max(data, d => d.sales)]).nice()
.range([height - margin.bottom, margin.top]);
return (
<svg width={width} height={height}>
<g>
{data.map(d => (
<rect
key={d.label}
x={x(d.label)}
y={y(d.sales)}
height={y(0) - y(d.sales)}
width={x.bandwidth()}
fill="steelblue"
/>
))}
</g>
</svg>
);
};
export default BarChart;
With this, you have a rudimentary bar chart component in D3.js that visualizes quarterly sales data.
4. Interacting and Refining with Cloving Chat
If the generated component requires adjustments or further enhancements, engage Cloving’s chat feature for more detailed interaction:
cloving chat -f src/components/BarChart.js
Example Chat Prompt
cloving> Could you add axis labels and a transition effect to the bars?
Cloving can refine the generated code to include an X-axis, a Y-axis, and transitions on your rectangles, helping make your visualization more informative and visually engaging.
5. Advanced Examples and Use Cases
Once you’re comfortable generating basic components, you can take advantage of Cloving’s capabilities for more sophisticated or specialized D3.js projects.
5.1 Multi-Series Line Chart
For data that changes over time across multiple categories, you might want a multi-series line chart. For instance, imagine you have data for different product lines over several months. You can prompt Cloving to generate a starting file:
cloving generate code --prompt "Create a multi-series line chart in D3.js for multiple product lines over time" --files src/components/MultiLineChart.js
The generated code might include:
- A time scale on the X-axis.
- A linear scale on the Y-axis.
- A line generator for each product category.
Cloving can also help you add a legend or interactive features like tooltips (more on that below).
5.2 Scatter Plot with Tooltips
If you have (x, y) coordinate data—maybe to track items by cost and popularity—Cloving can generate a scatter plot. Use the interactive chat to add features like tooltips that display the exact coordinates or additional metadata when hovering over a point:
cloving chat --prompt "Create tooltips for scatter plot circles that show cost and popularity data" -f src/components/ScatterPlot.js
Cloving might produce code that utilizes D3’s .append('title')
method, or even more sophisticated tooltip libraries, depending on your prompt.
5.3 Data Loading from External Sources
To load data from an external CSV or JSON file, you can ask Cloving for assistance:
cloving generate code --prompt "Load data from a CSV file and create a line chart using D3.js" --files src/components/CsvLineChart.js
Cloving may generate a snippet that uses d3.csv
or d3.json
, complete with asynchronous logic to fetch your data before rendering.
6. Including Additional Features
6.1 Basic Tooltip Example
Imagine you want to integrate a feature like tooltips for the bar chart above. You could do the following:
cloving chat --prompt "Add tooltips to display sales figures on hover to the bar chart" -f src/components/BarChart.js
Cloving might respond with a code snippet like this:
// src/components/BarChart.js (excerpt)
d3.select('svg')
.selectAll('rect')
.data(data)
.enter()
.append('title')
.text(d => `Sales: ${d.sales}`);
This snippet appends a simple title to each rectangle. When you hover over a bar in the browser, you’ll see a text tooltip that displays the sales figure.
6.2 Animated Transitions
For added visual flair, you can prompt Cloving to include transitions, such as animating the bars from zero height to their actual height:
cloving chat --prompt "Animate the bar chart so that bars grow from zero to their full height on load" -f src/components/BarChart.js
Cloving will typically add transition()
blocks to your existing code, helping you produce a more dynamic and engaging chart.
7. Best Practices for a Smooth Cloving + D3.js Workflow
- Use Clear Prompts: When asking Cloving to generate or refine code, be explicit about the features you want (e.g., “Add a legend,” “Animate the X-axis,” “Use React hooks,” etc.).
- Combine with Interactive Mode: The
cloving chat
command is a powerful way to refine code in real time. Ask Cloving how to fix errors, add new features, or optimize performance. - Experiment with Different Models: The
--model
flag lets you switch AI models if you’re not satisfied with the default suggestions. - Iterate with AI Feedback: Cloving can offer suggestions to improve code readability, structure your data, or handle edge cases—take advantage of its review features.
- Leverage Code Organization: Large visualizations often get unwieldy. Split logic into smaller components or utility functions, and allow Cloving to help you create them.
- Stay Up-to-Date: Both D3.js and Cloving evolve quickly. Regularly update Cloving and your D3.js version to access the latest features and improvements.
8. Integration with Modern Frameworks
If your setup involves React, Vue, or Svelte, Cloving can help you scaffold out D3.js components tailored to those frameworks. For example, you can ask:
cloving generate code --prompt "Create a React D3.js component for a responsive pie chart" --files src/components/PieChart.jsx
Cloving’s generated code might include best practices for hooking D3’s lifecycle into React’s hooks or Vue’s reactive API.
9. Automating Your Workflow with Cloving
If you’re working with multiple visualizations or a team, consider automating Cloving within your CI/CD pipeline. For instance, you can run Cloving commands on pull requests to generate or review D3.js components automatically.
name: Build and Test
on:
push:
branches: [ "main" ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install Node
uses: actions/setup-node@v2
with:
node-version: '16'
- name: Install Cloving
run: npm install -g cloving@latest
- name: Generate D3.js Code
run: cloving generate code --prompt "Update bar chart with new dataset" --files src/components/BarChart.js
- name: Build Project
run: npm run build
This approach ensures visualizations remain consistent and receive automated updates or refinements, particularly in a fast-moving development environment.
10. Conclusion
By integrating Cloving CLI into your D3.js visualization workflow, you optimize productivity and precision in developing custom data visualizations. Whether it’s bar charts, multi-series line charts, or complex interactive scatter plots, Cloving’s intelligent suggestions and interactive guidance help you quickly iterate on complex visuals and confidently handle tasks like loading external data, adding animations, and refining user interactions.
Experience the synergy of AI-driven development and D3.js by leveraging Cloving CLI—you’ll amplify your capacity to create visually compelling, data-driven applications that stand out. As with any AI-assisted tool, Cloving complements your expertise rather than replaces it, allowing you to push the boundaries of your front-end projects with speed and confidence.
Happy coding and visualizing!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.