Building a RESTful API with Django and GPT
Updated on June 26, 2024

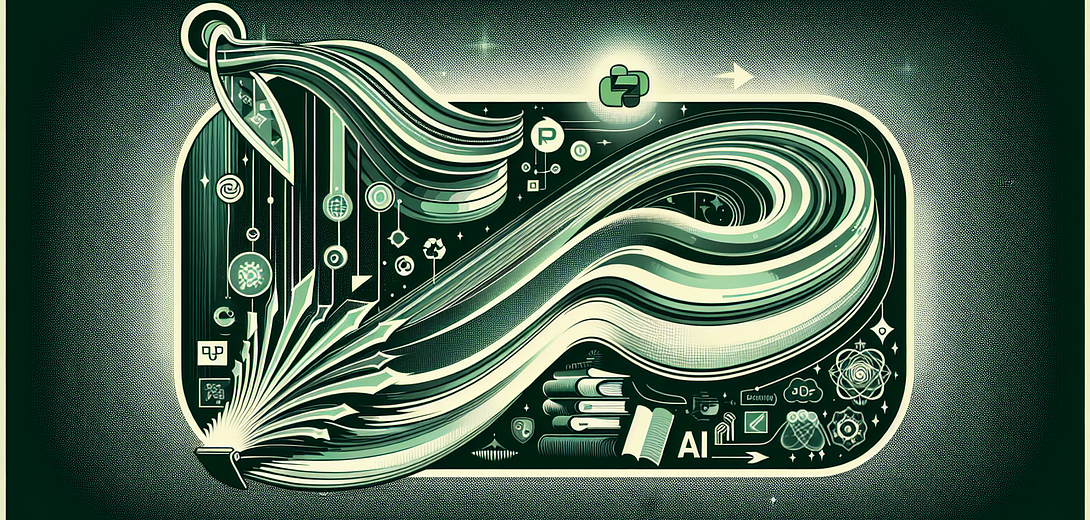
In today’s developing ecosystem, building RESTful APIs efficiently is crucial for the success of web applications. With the emergence of artificial intelligence (AI) tools like GPT, there’s a new paradigm in software development known as “cloving”—integrating human creativity and intuition with the computational power of AI. Through cloving, programmers can now achieve their development goals faster and more effectively.
In this blog post, we’ll explore how to incorporate GPT into your daily workflow while building a RESTful API with Django, offering practical examples, tips, and best practices to enhance your efficiency and productivity.
Understanding Cloving
Cloving is the strategic combination of human expertise and AI capabilities to solve problems more efficiently. It’s about harnessing the analytical power of AI while integrating human creativity and decision-making processes seamlessly.
Setting Up Your Django Project
Before diving into API construction, you need to set up your Django project. Here’s a quick way to get started:
I want to create a new Django project named "MyAPIProject." Can you provide the initial commands and basic setup?
GPT Response:
# Install Django
pip install django
# Create a new Django project
django-admin startproject MyAPIProject
# Navigate into your project directory
cd MyAPIProject
# Create a new Django app
python manage.py startapp api
# Add 'api' to your INSTALLED_APPS in MyAPIProject/settings.py
INSTALLED_APPS = [
...
'api',
]
1. Endpoint Scaffolding
Structuring your API endpoints correctly from the start is key to maintainable codebases. GPT can assist with the high-level structure and basic functions.
Example:
You want to create a simple RESTful API for managing books in your library:
I'm building an API to manage books in a library. I need endpoints for listing books, viewing details, creating new books, updating, and deleting. Can you provide the initial Django views and urls?
GPT Response:
# api/views.py
from rest_framework import generics
from .models import Book
from .serializers import BookSerializer
class BookListCreate(generics.ListCreateAPIView):
queryset = Book.objects.all()
serializer_class = BookSerializer
class BookDetail(generics.RetrieveUpdateDestroyAPIView):
queryset = Book.objects.all()
serializer_class = BookSerializer
# api/urls.py
from django.urls import path
from .views import BookListCreate, BookDetail
urlpatterns = [
path('books/', BookListCreate.as_view(), name='book-list-create'),
path('books/<int:pk>/', BookDetail.as_view(), name='book-detail'),
]
2. Model Generation
Creating models is central to Django applications. GPT can help generate models based on your requirements.
Example:
You need a model for the books in your library:
I need a Django model for books with the following attributes: title, author, published_date, and ISBN. Can you generate the model?
GPT Response:
# api/models.py
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=255)
author = models.CharField(max_length=255)
published_date = models.DateField()
ISBN = models.CharField(max_length=13, unique=True)
def __str__(self):
return self.title
# After creating the model, run the migrations
# python manage.py makemigrations api
# python manage.py migrate
3. Serializers
Serializers enable you to convert model instances to JSON and vice versa. Let GPT help you generate the necessary serializers.
Example:
Create a serializer for your Book model:
Can you provide a Django REST framework serializer for the Book model?
GPT Response:
# api/serializers.py
from rest_framework import serializers
from .models import Book
class BookSerializer(serializers.ModelSerializer):
class Meta:
model = Book
fields = ['id', 'title', 'author', 'published_date', 'ISBN']
4. Documentation
Clear documentation is essential for team collaboration and maintenance. Ask GPT to help generate concise documentation for your API endpoints.
Example:
Generate documentation for the Book API endpoints:
Can you create example documentation for the Book API endpoints you provided earlier?
GPT Response:
# Book API
## Endpoints
### List and Create Books
- **URL:** /books/
- **Method:** GET, POST
- **Description:**
- **GET:** Retrieve a list of all books.
- **POST:** Create a new book.
- **Request Body (POST):**
```json
{
"title": "Book Title",
"author": "Author Name",
"published_date": "YYYY-MM-DD",
"ISBN": "ISBN Number"
}
- Response:
[{ "id": 1, "title": "Book Title", "author": "Author Name", "published_date": "YYYY-MM-DD", "ISBN": "ISBN Number" }]
Retrieve, Update, and Delete Book
- URL: /books/:id/
- Method: GET, PUT, DELETE
- Description:
- GET: Retrieve details of a specific book by ID.
- PUT: Update details of a specific book.
- DELETE: Remove a specific book.
- Request Body (PUT):
Same as POST request. - Response:
Same as GET request for specific book.
### Conclusion
Building a RESTful API with Django becomes significantly more efficient and effective when integrating the power of AI like GPT. Through cloving, you can combine your intuitive understanding of the application domain with GPT's robust analytical capabilities to streamline your development workflow.
Embrace the synergy of cloving and integrate GPT into your programming toolkit to achieve enhanced productivity, reduced errors, and maintain high standards in your codebase.
### Bonus Follow-up Prompts
To further refine and enhance your workflow, here are a few extra prompts:
```plaintext
How can I configure GitHub to run these tests automatically for me?
Generate factory data for the Book model.
What are other useful GPT prompts I could integrate into my Django REST API development process?
By employing these prompts, you can further optimize your use of GPT, making your development process smoother and more efficient. Use cloving to its full potential and transform your AI development experience!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.