Building a Flask Application with Help from GPT
Updated on June 26, 2024

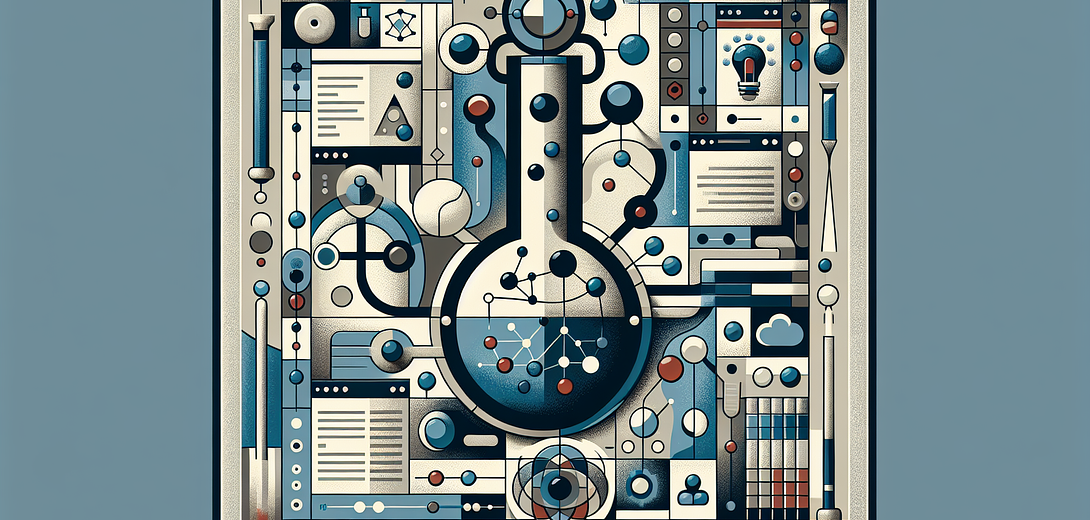
In the dynamic world of software development, building web applications can often be a daunting task, especially when working with frameworks like Flask. By adopting the principles of cloving—integrating human creativity and intuition with the powerful capabilities of artificial intelligence (AI)—developers can streamline their workflows and enhance productivity. In this post, we’ll explore how using AI tools like GPT can help you build a Flask application, making your development process more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. It’s not just about using AI tools; it’s about creating a symbiotic relationship where both human and machine strengths are leveraged to solve problems more effectively.
1. Project Setup and Initialization
Starting a new Flask project involves setting up the environment, creating necessary directories, and installing dependencies. GPT can assist in generating the boilerplate code and guiding you through the setup process.
Example:
You can ask GPT for help with initializing a new Flask project:
How do I set up a new Flask project with virtual environment and basic structure?
GPT’s response might include steps like:
-
Create a project directory and navigate into it:
mkdir my_flask_app cd my_flask_app
-
Set up a virtual environment:
python3 -m venv venv source venv/bin/activate
-
Install Flask:
pip install Flask
-
Create the basic project structure:
my_flask_app/ ├── app/ │ ├── __init__.py │ └── routes.py ├── venv/ ├── .gitignore └── run.py
2. Building Routes and Views
Creating routes and views in Flask is a core part of developing your application. GPT can help you generate the necessary code for common functionalities.
Example:
Suppose you want to create a route for the home page:
How do I create a home route in Flask that returns a 'Hello, World!' message?
GPT will generate the following code:
# app/routes.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello, World!'
Additionally, GPT can help you understand and implement more complex routes and view functions.
3. Database Integration
Integrating a database into your Flask application is essential for handling data. GPT can guide you through setting up and interacting with a database using SQLAlchemy.
Example:
You can ask GPT to help with setting up a SQLite database:
How do I set up a SQLite database with SQLAlchemy in my Flask application?
GPT will provide instructions and code snippets:
-
Install Flask-SQLAlchemy:
pip install Flask-SQLAlchemy
-
Update
__init__.py
to configure the database:from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///site.db' db = SQLAlchemy(app)
-
Define a model:
# app/models.py from app import db class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(20), unique=True, nullable=False) email = db.Column(db.String(120), unique=True, nullable=False) password = db.Column(db.String(60), nullable=False) def __repr__(self): return f"User('{self.username}', '{self.email}')"
4. Form Handling and Validation
Handling forms and validating user input are common tasks in web development. GPT can help you create forms and validate data using Flask-WTF.
Example:
Ask GPT for help with creating a registration form:
How do I create a registration form with Flask-WTF?
GPT will suggest:
-
Install Flask-WTF:
pip install Flask-WTF
-
Create a form class:
# app/forms.py from flask_wtf import FlaskForm from wtforms import StringField, PasswordField, SubmitField from wtforms.validators import DataRequired, Length, Email, EqualTo class RegistrationForm(FlaskForm): username = StringField('Username', validators=[DataRequired(), Length(min=2, max=20)]) email = StringField('Email', validators=[DataRequired(), Email()]) password = PasswordField('Password', validators=[DataRequired()]) confirm_password = PasswordField('Confirm Password', validators=[DataRequired(), EqualTo('password')]) submit = SubmitField('Sign Up')
5. Testing and Debugging
Testing is crucial for ensuring the reliability of your Flask application. GPT can help you write tests and debug issues.
Example:
Generate tests for your home route:
How do I write a test for the home route in Flask?
GPT will provide a test case:
# tests/test_routes.py
import unittest
from app import app
class FlaskTestCase(unittest.TestCase):
def test_home(self):
tester = app.test_client(self)
response = tester.get('/')
self.assertEqual(response.status_code, 200)
self.assertIn(b'Hello, World!', response.data)
if __name__ == '__main__':
unittest.main()
Conclusion
Building a Flask application with the assistance of GPT exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. Integrating GPT into your development workflow can enhance your productivity, reduce errors, and keep up with best practices. Embrace cloving and discover how this synergistic approach can transform your programming experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.