Automating Tailscale VPN Setup with Terraform and AI
Updated on July 05, 2024

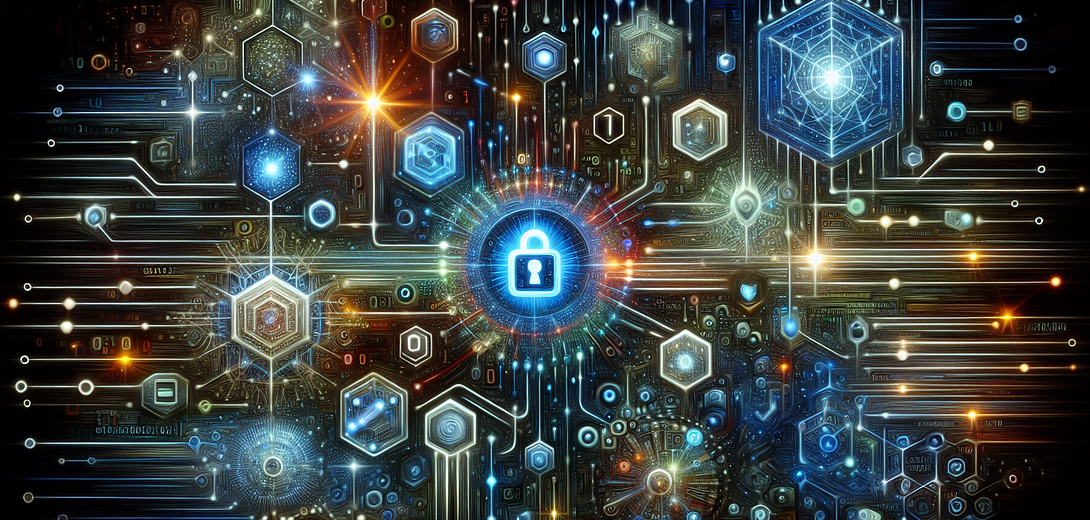
In today’s rapidly evolving tech landscape, efficiency and precision are paramount. One of the groundbreaking approaches to achieve this is cloving—integrating human creativity with the powerful processing capabilities of artificial intelligence.
This post focuses on how you, as a programmer, can incorporate GPT into your daily workflows to automate the setup of Tailscale VPN using Terraform and configure existing servers to be protected behind the new Tailscale configuration. By leveraging this fusion, you can expedite your tasks, ensure accuracy, and free up your time for more strategic initiatives.
Understanding Cloving
Cloving harnesses the complementary strengths of humans and AI: human intuition and creativity combined with AI’s analytical prowess. Instead of viewing AI as a mere tool, consider it a partner in achieving better, faster outcomes.
1. Generating Terraform Scripts
Terraform is great for infrastructure automation, but writing scripts from scratch can be tedious. GPT can generate initial templates, helping you get started quickly.
Example:
You want to initialize a Tailscale network. You can ask GPT:
Generate a Terraform template to set up a basic Tailscale VPN network.
GPT might provide a template like:
provider "tailscale" {
api_key = "your_api_key_here"
}
resource "tailscale_network" "example" {
name = "example-network"
}
resource "tailscale_device" "example" {
network_id = tailscale_network.example.id
device_id = "your_device_id_here"
}
2. Configuring Existing Servers Behind Tailscale
With the basic Tailscale network set up using Terraform, GPT can now help you configure existing servers to be protected behind the Tailscale VPN. This involves installing Tailscale on the servers and ensuring they are connected to the Tailscale network.
Example:
You need to secure an existing server behind Tailscale:
Generate a script to install Tailscale on an existing server and connect it to the Tailscale network.
GPT might generate a script like:
#!/bin/bash
# Update system and install required packages
sudo apt-get update
sudo apt-get install -y curl
# Install Tailscale
curl -fsSL https://tailscale.com/install.sh | sh
# Authenticate and connect to the Tailscale network
sudo tailscale up --authkey your_authkey_here
To integrate this with your Terraform setup, you can use remote-exec provisioners:
resource "null_resource" "configure_tailscale" {
connection {
type = "ssh"
host = "your_server_ip"
user = "your_ssh_user"
private_key = file("~/.ssh/id_rsa")
}
provisioner "remote-exec" {
inline = [
"curl -fsSL https://tailscale.com/install.sh | sh",
"sudo tailscale up --authkey ${var.tailscale_auth_key}",
]
}
}
3. Enhancing Configuration with Best Practices
To avoid potential pitfalls and ensure best practices, GPT can offer suggestions on how to enhance your Terraform scripts.
Example:
You want to ensure your Terraform configuration adheres to best security practices. Ask GPT:
What are the security best practices for configuring Tailscale with Terraform?
GPT may suggest:
### Security Best Practices
1. **Secure Storage for Secrets**
- Use secrets management systems like HashiCorp Vault or AWS Secrets Manager for Tailscale API keys.
- Avoid hardcoding secrets in Terraform files.
2. **Least Privilege Principle**
- Ensure Tailscale API keys have minimal necessary permissions.
- Use least privilege IAM roles for Terraform operations.
3. **Version Control**
- Store Terraform configurations in Git.
- Implement code reviews for changes.
4. **State File Security**
- Use remote backends (e.g., AWS S3) for storing state files.
- Ensure state files are encrypted.
5. **Regular Updates**
- Pin and regularly update Terraform and provider versions.
6. **Network Security**
- Configure firewall rules to restrict access.
- Adopt zero-trust network principles.
7. **Monitoring and Auditing**
- Enable logging and monitor Terraform operations.
- Maintain and review audit trails.
8. **Multi-Factor Authentication (MFA)**
- Use MFA for accessing Tailscale and Terraform environments.
9. **Secure Communication**
- Ensure TLS for all communications.
- Use SSH keys for accessing instances.
10. **Backup and Recovery**
- Regularly back up Terraform state and Tailscale configurations.
- Develop and test disaster recovery plans.
### Example Terraform Configuration
```hcl
provider "tailscale" {
api_key = var.tailscale_api_key
}
resource "tailscale_device" "example" {
name = "example-device"
key = var.tailscale_device_key
}
variable "tailscale_api_key" {
type = string
sensitive = true
}
variable "tailscale_device_key" {
type = string
sensitive = true
}
terraform {
backend "s3" {
bucket = "your-tf-state-bucket"
key = "path/to/your/statefile"
region = "your-region"
encrypt = true
dynamodb_table = "your-lock-table"
}
}
This summary highlights essential practices and provides a concise Terraform configuration example.```
4. Optimizing Network Configuration
GPT can help recompute and optimize network configurations, ensuring you use resources most efficiently.
Example:
You need to optimize the network settings for better performance:
How can I optimize network settings in Tailscale for better performance using Terraform?
GPT might recommend adjustments like:
### Optimizing Tailscale Network Settings for Performance with Terraform
1. **Enable Derp**
- Use Tailscale's DERP (Detour Encrypted Routing Protocol) for better latency and reliability.
2. **Set Preferred Routes**
- Define preferred routes to optimize traffic flow.
3. **Use Exit Nodes**
- Designate exit nodes for centralized internet traffic management.
4. **Adjust MTU Size**
- Set the MTU (Maximum Transmission Unit) to an optimal size for your network.
5. **Monitor and Adjust Peering**
- Regularly review and adjust peering configurations for optimal paths.
### Example Terraform Configuration
```hcl
provider "tailscale" {
api_key = var.tailscale_api_key
}
resource "tailscale_device" "example" {
name = "example-device"
key = var.tailscale_device_key
// Enable DERP
derp = true
// Set preferred routes
routes = ["192.168.1.0/24"]
// Designate exit node
exit_node = true
// Adjust MTU size
mtu = 1280
}
variable "tailscale_api_key" {
type = string
sensitive = true
}
variable "tailscale_device_key" {
type = string
sensitive = true
}
terraform {
backend "s3" {
bucket = "your-tf-state-bucket"
key = "path/to/your/statefile"
region = "your-region"
encrypt = true
dynamodb_table = "your-lock-table"
}
}
### Key Actions
- Enable DERP for improved latency.
- Set preferred routes and exit nodes for traffic management.
- Adjust MTU for network efficiency.
- Monitor and optimize peering settings.
5. Automated Documentation
Keeping documentation up-to-date is crucial but often neglected. GPT can auto-generate and maintain comprehensive documentation for your Terraform scripts.
Example:
Generate documentation for your Terraform configuration:
Generate documentation for this Terraform configuration: [Terraform script contents]
GPT will produce detailed documentation, ensuring every aspect of your setup is well-documented and easy to understand:
# Tailscale VPN Terraform Setup
### Providers
- **tailscale**:
- `api_key`: Your API key for accessing Tailscale.
### Resources
- **tailscale_network**:
- `name`: The name of your Tailscale network.
- **tailscale_device**:
- `network_id`: The ID of the configured network.
- `device_id`: The unique identifier of the device.
6. Generating and Running Automatic Tests
Automated tests ensure your configurations work as intended. GPT can help write test cases and guide you on configuring CI/CD pipelines to automatically apply and test these configurations.
Example:
Generate test cases for your Terraform configuration:
Generate test cases for the Terraform Tailscale setup using Terratest.
GPT might generate something like:
package test
import (
"testing"
"github.com/gruntwork-io/terratest/modules/terraform"
)
func TestTailscaleVPN(t *testing.T) {
opts := &terraform.Options{
TerraformDir: "../path/to/your/terraform/code",
}
defer terraform.Destroy(t, opts)
terraform.InitAndApply(t, opts)
// Add additional tests below
output := terraform.Output(t, opts, "network_id")
if output == "" {
t.Fatal("Expected an ID but got an empty string")
}
}
Conclusion
Automating Tailscale VPN setup with Terraform, empowered by the synergy of cloving, can significantly enhance your workflow. By integrating human creativity and AI’s analytical capabilities, you can create efficient, effective, and reliable infrastructure setups faster and with greater precision. Embrace the practice of cloving and witness a transformation in your productivity and problem-solving approach.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.