Automating Rust Unit Test Creation with GPT
Updated on July 02, 2024

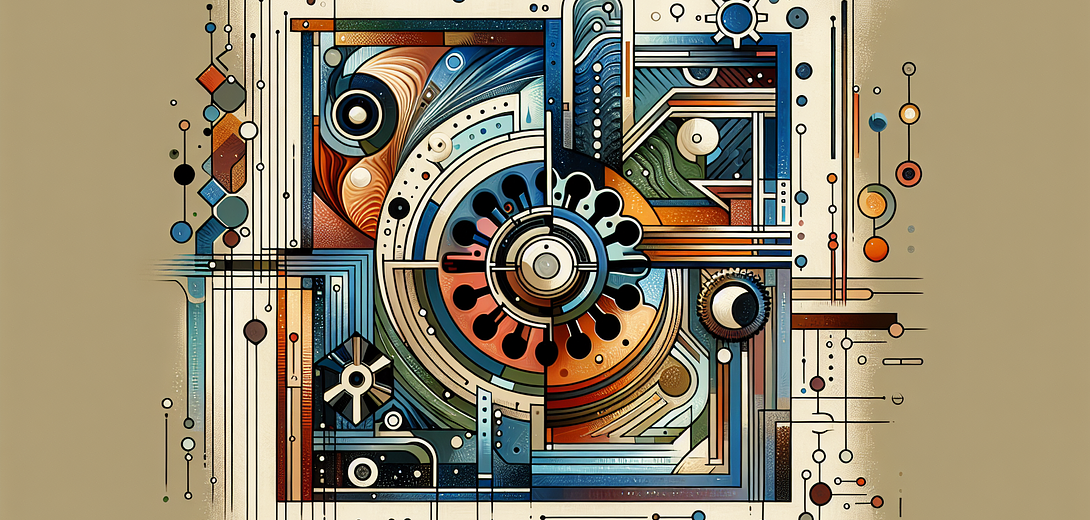
Debugging is often a time-consuming and frustrating process, especially when crafting unit tests to ensure your code behaves as expected. By integrating human intuition and creativity with the robust analytical capabilities of artificial intelligence, programmers can streamline their testing workflows.
In this post, we’ll explore how AI tools like GPT can help you automate Rust unit test creation, making your programming tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s computational power to achieve common goals more effectively. It’s not just about using AI tools; it’s about creating a symbiotic relationship where human and machine strengths are leveraged to solve problems more efficiently.
1. Generating Basic Unit Tests
Writing unit tests can be repetitive and tedious, but it’s crucial for ensuring code quality. GPT can automate this process by generating basic unit tests from your code snippets.
Example:
Suppose you have a function in your Rust project that sums two integers. You can prompt GPT to generate a unit test for this function:
Generate a unit test for the following Rust function that sums two integers:
```rust
fn sum(a: i32, b: i32) -> i32 {
a + b
}
GPT will generate a Rust unit test, saving you precious time:
// tests/test_sum.rs
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_sum() {
assert_eq!(sum(2, 3), 5);
assert_eq!(sum(-1, 1), 0);
assert_eq!(sum(0, 0), 0);
}
}
Running the Tests:
To run the tests, navigate to your project directory in the terminal and execute:
cargo test
This will automatically find and execute all the test modules within your Rust project.
2. Testing Edge Cases
Edge cases are often overlooked yet crucial for robust software. GPT can help identify and generate tests for these scenarios.
Example:
If you need to test edge cases for a function that calculates the factorial of a number, you can describe the function and ask GPT for test cases:
Generate edge case unit tests for the following Rust function that calculates the factorial of a number:
```rust
fn factorial(n: u32) -> u32 {
if n == 0 {
1
} else {
n * factorial(n - 1)
}
}
GPT will generate tests that cover edge cases:
// tests/test_factorial.rs
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_factorial() {
assert_eq!(factorial(0), 1); // Edge case: factorial of 0
assert_eq!(factorial(1), 1); // Edge case: factorial of 1
assert_eq!(factorial(5), 120);
assert_eq!(factorial(10), 3628800);
}
}
Running the Tests:
To run these newly created tests, you can use the same cargo command:
cargo test
3. Refining and Optimizing Tests
Keeping up with best practices in test writing can be challenging. GPT can offer insights and improvements to your existing tests.
Example:
If you already have some unit tests but want to optimize them for readability and performance, ask GPT:
Here are my current Rust unit tests for a factorial function. How can I improve them for readability and performance?
```rust
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_factorial() {
assert_eq!(factorial(0), 1);
assert_eq!(factorial(1), 1);
assert_eq!(factorial(2), 2);
assert_eq!(factorial(3), 6);
assert_eq!(factorial(4), 24);
assert_eq!(factorial(5), 120);
assert_eq!(factorial(6), 720);
}
}
GPT will provide suggestions to refine your tests:
// tests/test_factorial_optimized.rs
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_factorial() {
let test_cases = [
(0, 1),
(1, 1),
(2, 2),
(3, 6),
(4, 24),
(5, 120),
(6, 720),
];
for (input, expected) in test_cases {
assert_eq!(factorial(input), expected);
}
}
}
Running the Tests:
After optimizing your tests, run them with:
cargo test
4. Documentation and Explanation
Understanding and documenting your unit tests can be time-consuming. GPT can help by generating detailed documentation for your tests.
Example:
When you need to document your test cases, ask GPT:
Generate documentation for the following Rust unit test module:
```rust
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_factorial() {
let test_cases = [
(0, 1),
(1, 1),
(2, 2),
(3, 6),
(4, 24),
(5, 120),
(6, 720),
];
for (input, expected) in test_cases {
assert_eq!(factorial(input), expected);
}
}
}
GPT will generate useful documentation:
/// This module contains unit tests for the `factorial` function.
///
/// The `test_factorial` function tests various input values to ensure
/// the `factorial` function returns the expected results. It covers:
/// - Basic cases (factorial of 0 and 1)
/// - Small integers (2 through 6)
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_factorial() {
let test_cases = [
(0, 1),
(1, 1),
(2, 2),
(3, 6),
(4, 24),
(5, 120),
(6, 720),
];
for (input, expected) in test_cases {
assert_eq!(factorial(input), expected);
}
}
}
Running the Tests:
Execute cargo test
to run the tests and ensure they are well-documented for future reference.
5. Advanced Testing Scenarios
For more complex scenarios, such as integration tests or property-based testing, GPT can assist in generating and structuring the tests appropriately.
Example:
Assume you have a Rust function that processes a list of integers by applying a series of transformations. To write a property-based test, you can prompt GPT:
Generate a property-based test for the following Rust function that processes a list of integers:
```rust
fn process_list(list: Vec<i32>) -> Vec<i32> {
list.iter().map(|x| x * 2).filter(|x| x % 3 != 0).collect()
}
GPT will provide a property-based test using the quickcheck
library:
// tests/test_process_list.rs
extern crate quickcheck;
#[cfg(test)]
mod tests {
use super::*;
use quickcheck::quickcheck;
quickcheck! {
fn test_process_list(xs: Vec<i32>) -> bool {
let processed = process_list(xs.clone());
for x in processed {
if x % 3 == 0 {
return false; // Property: No element should be divisible by 3
}
if xs.contains(&(x / 2)) == false {
return false; // Property: Every processed element should be double an element in the input list
}
}
true
}
}
}
Running the Tests:
Run these property-based tests with:
cargo test
Conclusion
Automating Rust unit test creation with AI assistance exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. Integrating GPT into your testing workflow can enhance your productivity, reduce errors, and keep you up-to-date with best practices. Embrace cloving and discover how this synergistic approach can transform your programming experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the examples provided above:
How can I configure GitHub Actions to run these tests automatically for me?
And another:
Generate accompanying test data for edge cases.
And one more:
What are other GPT prompts I could use to make this process even more efficient?
By incorporating these additional prompts into your workflow, you’ll be able to leverage GPT more effectively, achieving an even greater level of productivity and code quality.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.