Automating Java API Endpoints with GPT for Seamless Backend Integration
Updated on January 20, 2025

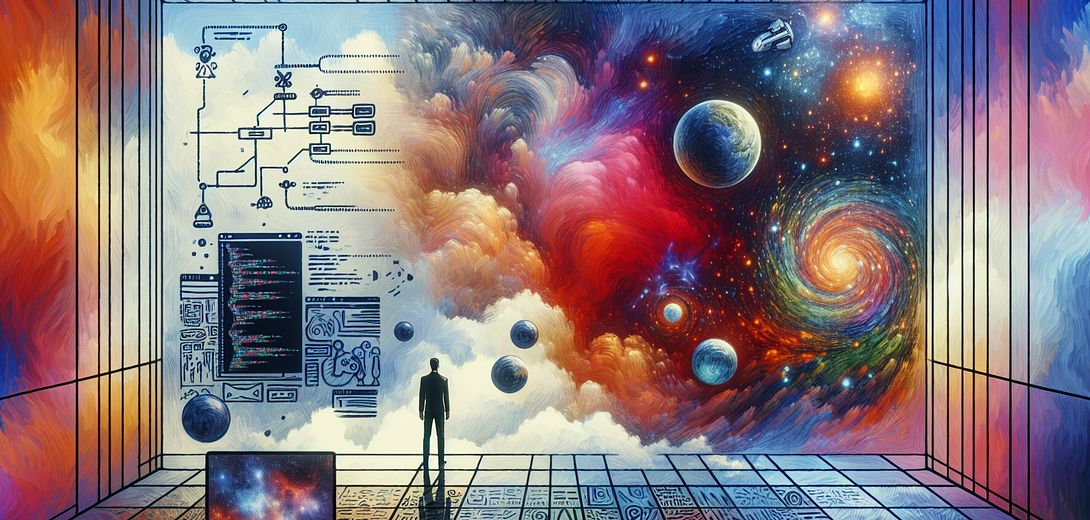
Backend development can often be a tedious process with repetitive tasks like setting up API endpoints and ensuring seamless integration. The Cloving CLI tool, powered by AI, can help to automate these tasks, allowing developers to focus on higher-level coding and logic. In this blog post, we’ll explore how you can automate Java API endpoints using the Cloving CLI, enhancing your productivity and streamlining backend development.
Understanding the Cloving CLI
Cloving is a command-line tool that leverages GPT models to generate context-aware code snippets, making it easier to complete various programming tasks efficiently. By integrating Cloving into your workflow, you can automate the creation of API endpoints in Java and more.
1. Setting Up Cloving
Before automating Java API endpoints, ensure that Cloving is installed and configured properly.
Installation:
Install Cloving globally:
npm install -g cloving@latest
Configuration:
Set up Cloving to use your preferred AI model and API key:
cloving config
Follow the prompts to enter your API key, model, and other settings.
2. Initializing Your Java Project
To start automating Java API endpoints with Cloving, initialize Cloving in your project:
cloving init
This command sets up Cloving in your project directory, allowing it to understand the project context.
3. Generating API Endpoints
Once everything is set up, you can generate Java API endpoints. For instance, suppose you’re developing an e-commerce backend and need endpoints for product management.
Example:
Generate a Java API endpoint for creating a new product:
cloving generate code --prompt "Generate a Java Spring Boot REST API endpoint to create a new product" --files src/main/java/com/example/product/ProductController.java
Cloving will generate code based on the context and prompt:
// src/main/java/com/example/product/ProductController.java
@PostMapping("/products")
public ResponseEntity<Product> createProduct(@RequestBody Product product) {
Product savedProduct = productService.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(savedProduct);
}
This snippet demonstrates a simple POST endpoint using Spring Boot to create a new product.
4. Reviewing and Revising Endpoint Code
After generating an endpoint, you can revise it if necessary. Cloving allows you to make tweaks and request explanations to better understand the generated code.
Example:
Revise the endpoint to add basic validation:
Revise to include validation for product name and price
You can then save or further refine the code with additional prompts and clarifications.
5. Generating Unit Tests for API Endpoints
Ensure your API endpoints are reliable by generating unit tests using Cloving.
Example:
Generate unit tests for the created endpoint:
cloving generate unit-tests -f src/test/java/com/example/product/ProductControllerTests.java
Cloving will provide relevant unit tests tailored to your endpoint, increasing test coverage and improving code quality.
// src/test/java/com/example/product/ProductControllerTests.java
@Test
public void testCreateProduct() throws Exception {
Product product = new Product("TestProduct", 50.0);
mockMvc.perform(post("/products")
.contentType(MediaType.APPLICATION_JSON)
.content(objectMapper.writeValueAsString(product)))
.andExpect(status().isCreated())
.andExpect(jsonPath("$.name").value("TestProduct"))
.andExpect(jsonPath("$.price").value(50.0));
}
6. Using Cloving Chat for Complex Endpoint Logic
For complex endpoints or when you require more in-depth assistance, use Cloving’s interactive chat feature:
cloving chat -f src/main/java/com/example/product/ProductController.java
This opens a chat session where you can ask for complex logic implementations, database integrations, or advice on best practices.
7. Generating Shell Scripts for Deployment
You can also generate scripts to automate the deployment of Java applications:
cloving generate shell --prompt "Create a shell script to deploy a Spring Boot application"
This provides an executable script to streamline your deployment process.
#!/bin/bash
echo "Building the Spring Boot application..."
mvn clean install
echo "Deploying the application..."
java -jar target/*.jar
Conclusion
Automating Java API endpoints with the Cloving CLI demonstrates how AI can revolutionize backend development. By generating context-aware code, streamlining testing, and offering continuous support through interactive chat, Cloving significantly enhances development workflows, liberating developers from repetitive tasks.
As you integrate Cloving into your workflow, remember to leverage its capabilities for various programming tasks and take full advantage of its AI-powered features to boost productivity and focus on creating robust, high-quality applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.