Automating Golang Code Writing Using GPT
Updated on April 21, 2025

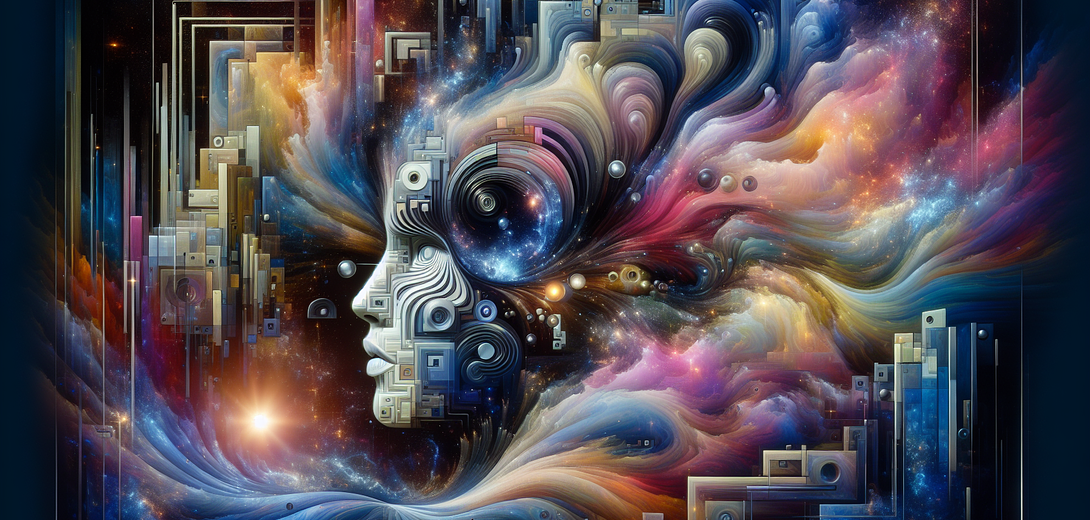
As a Golang developer, you’re no stranger to the efficiency and performance that comes with Go. However, even the most seasoned developers can benefit from streamlining their workflows with an AI-powered assistant. Enter Cloving CLI—an AI-powered command-line interface designed to boost productivity and code quality, specifically tailored for Golang developers. In this post, we’ll walk through how you can leverage Cloving CLI to automate your Go code writing, making your workflow smoother and more efficient.
Getting Started with Cloving CLI
Before diving into the code automation features, let’s ensure you have Cloving set up.
Installation and Configuration
First, install Cloving globally using npm:
npm install -g cloving@latest
With Cloving installed, configure it to work with your preferred AI model:
cloving config
Follow the prompts to input your API key and select the model best suited for Golang projects.
Initializing Your Go Project
Navigate to your Go project directory, and initialize Cloving:
cloving init
This command will analyze your project structure and create a cloving.json
file to store settings and metadata specific to your project.
Automating Code Generation
Cloving CLI can significantly enhance your coding experience by generating code snippets, complete functions, and even entire modules based on simple prompts.
1. Generating Golang Code
Suppose you’re building a REST API and need a handler function for user login. Use Cloving’s code generation ability as follows:
cloving generate code --prompt "Create a login handler for a REST API in Go"
This command will produce a code snippet tailored to your prompt. Here’s an example output:
package main
import (
"encoding/json"
"net/http"
)
type LoginRequest struct {
Username string `json:"username"`
Password string `json:"password"`
}
func loginHandler(w http.ResponseWriter, r *http.Request) {
var req LoginRequest
if err := json.NewDecoder(r.Body).Decode(&req); err != nil {
http.Error(w, "Invalid request payload", http.StatusBadRequest)
return
}
// Implement authentication logic here
w.WriteHeader(http.StatusOK)
json.NewEncoder(w).Encode("Login Successful")
}
2. Automating Unit Test Generation
Testing is crucial to maintain code quality. Cloving can automate unit test generation for your Go functions. For example, to create tests for the login handler:
cloving generate unit-tests -f main.go
This will generate a unit test file for your existing code:
package main
import (
"bytes"
"net/http"
"net/http/httptest"
"testing"
)
func TestLoginHandler(t *testing.T) {
handler := http.HandlerFunc(loginHandler)
t.Run("Valid login request", func(t *testing.T) {
reqBody := `{"username":"testuser", "password":"secret"}`
req, err := http.NewRequest("POST", "/login", bytes.NewBufferString(reqBody))
if err != nil {
t.Fatalf("Could not create request: %v", err)
}
rec := httptest.NewRecorder()
handler.ServeHTTP(rec, req)
if rec.Code != http.StatusOK {
t.Errorf("Expected status OK; got %v", rec.Code)
}
})
}
3. Using Cloving Chat for Complex Scenarios
For more complex tasks or when you need step-by-step assistance, the Cloving chat function offers real-time interaction with the AI:
cloving chat -f main.go
During the chat session, you can ask for code explanations, additional functionality, or modifications:
cloving> Enhance the login handler to support JSON Web Token for authentication
Sure! Here’s how you can modify the login handler to issue a JWT upon successful login:
...
4. Writing Effective Commit Messages
Cloving can help generate detailed commit messages which capture the essence of your changes, making version control more informative:
cloving commit
This command will analyze recent changes and suggest a descriptive commit message:
Implement login handler with basic request validation and token generation
Conclusion
By integrating Cloving CLI into your Golang development workflow, you can significantly enhance your efficiency and code quality. From generating boilerplate code and unit tests to improving commit messages, Cloving acts as a robust AI-powered assistant. Embrace this tool to automate repetitive tasks and keep your focus on crafting robust, high-performance Go applications.
Remember, while Cloving is a powerful tool, it’s there to assist and not replace your expertise. Use it to augment your capabilities and streamline your coding process. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.