Automating CRUD Operations in Laravel with GPT
Updated on April 14, 2025

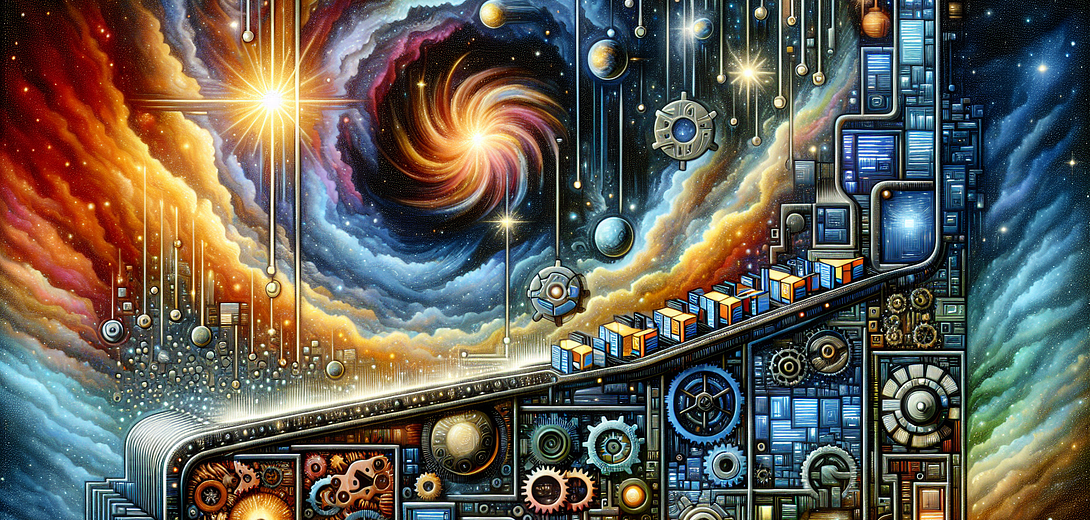
In modern web development, creating Create, Read, Update, and Delete (CRUD) operations is a ubiquitous task, especially when working with frameworks like Laravel. Automating these operations can significantly reduce development time and improve code quality. In this blog post, we’ll demonstrate how to use the Cloving CLI, an AI-powered command-line tool, to seamlessly automate CRUD operations in Laravel projects. By integrating AI, you can boost productivity and ensure consistent, high-quality code across your applications.
Setting Up Cloving CLI
Before venturing into automation, make sure Cloving is installed and configured.
Installation:
Begin by installing Cloving globally via npm:
npm install -g cloving@latest
Configuration:
Next, configure Cloving to work with your API key and preferred AI model:
cloving config
Follow the interactive setup steps to complete the configuration process.
Initializing Your Laravel Project
To enable Cloving to recognize the context of your Laravel project, initialize Cloving in your project directory.
cd path/to/your/LaravelProject
cloving init
This creates a cloving.json
file containing metadata about your Laravel application.
Automating CRUD Operations
To automate CRUD operations, we will use Cloving’s generate
command, which requires a clear and concise prompt, alongside the context provided by your files.
1. Creating a Laravel Model and Migration
Suppose you want to create a model and migration for a Product
entity. Use the cloving generate
command as follows:
cloving generate code --prompt "Create a Laravel model and migration for Product with fields: name (string), price (decimal), and quantity (integer)" --files app/Models/Product.php
Example Output:
Cloving considers your prompt and the Laravel framework context, generating files similar to the following:
app/Models/Product.php:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = ['name', 'price', 'quantity'];
}
database/migrations/xxxx_xx_xx_create_products_table.php:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateProductsTable extends Migration
{
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->decimal('price', 8, 2);
$table->integer('quantity');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('products');
}
}
2. Generating CRUD Controller
To automate the creation of a controller handling CRUD operations, execute:
cloving generate code --prompt "Generate a Laravel CRUD controller for Product" --files app/Http/Controllers/ProductController.php
Example Output:
app/Http/Controllers/ProductController.php:
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
public function index()
{
return Product::all();
}
public function store(Request $request)
{
$product = Product::create($request->all());
return response()->json($product, 201);
}
public function show($id)
{
return Product::findOrFail($id);
}
public function update(Request $request, $id)
{
$product = Product::findOrFail($id);
$product->update($request->all());
return response()->json($product, 200);
}
public function destroy($id)
{
Product::destroy($id);
return response()->json(null, 204);
}
}
3. Customizing and Revising Generated Code
Feel free to refine generated code by asking through Cloving’s interactive props. You can run the generate
command in interactive mode to auto-save and allow for further revisions:
cloving generate code --prompt "Update ProductController to validate data" --files app/Http/Controllers/ProductController.php --interactive
4. Running Migrations
Once you’ve generated the model and migration files, run the migrations to apply changes:
php artisan migrate
Conclusion
Through Cloving CLI, automating CRUD operations in a Laravel project is streamlined, allowing developers to focus more on unique, challenging aspects of their applications. By incorporating AI into your workflow, you not only save time but also maintain a consistent, high-quality codebase. Remember, Cloving is a supportive tool, meant to amplify your coding proficiency without replacing your expertise.
Explore Cloving CLI today, and empower your Laravel development journey!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.