Automating C# ASP.NET Controllers with AI and GPT
Updated on March 30, 2025

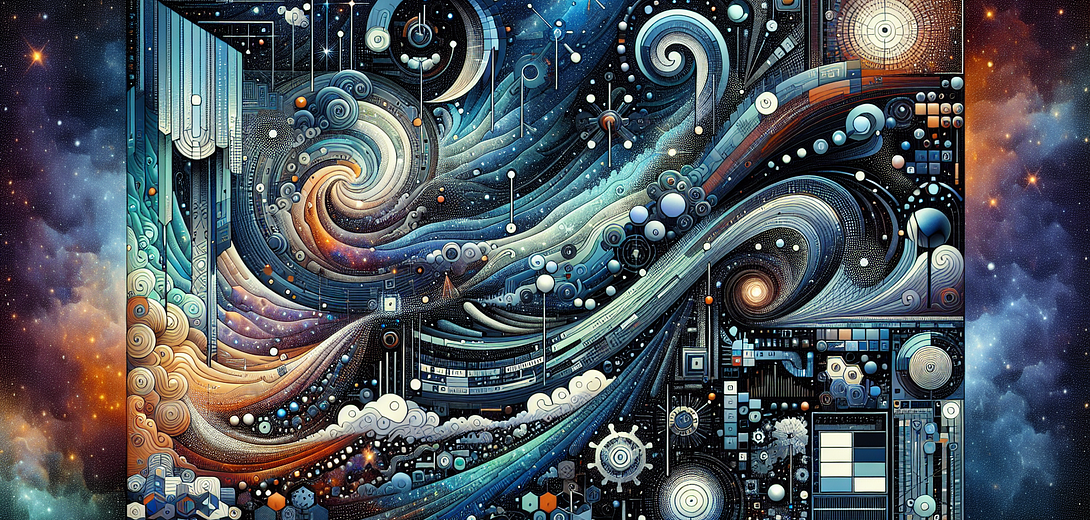
As a C# developer, automating repetitive tasks can greatly enhance your productivity. With the Cloving CLI tool, you can leverage AI, particularly GPT models, to automate and streamline the creation and management of ASP.NET Controllers. This guide will walk you through using Cloving to automate controller generation, manage your code with ease, and enhance your workflow.
Getting Started with Cloving CLI
1. Installation
First, ensure Cloving is installed on your system. Install it globally using npm:
npm install -g cloving@latest
2. Configuration
Configure Cloving to use your preferred AI model by entering:
cloving config
Follow the prompts to set up your API key and model preferences to get the best out of Cloving’s AI capabilities.
3. Initialize Your C# Project
Navigate to your C# project directory and initialize it with Cloving to set up the necessary context:
cloving init
This creates a cloving.json
file, which will help Cloving understand your project structure and context.
Automating Controller Generation
ASP.NET controllers are central to building web APIs. Automating their creation can save you significant time and effort.
Using Cloving to Generate a New Controller
Suppose you’re tasked with creating a new controller for managing a list of products. Use Cloving’s generate
command:
cloving generate code --prompt "Create an ASP.NET Controller for managing products with CRUD operations" --files Controllers/ProductController.cs --save
The command instructs Cloving to understand your project’s C# context, and generate an appropriate ASP.NET Controller. The --save
option ensures that the generated code is automatically saved to the specified file.
Example Generated Code
using System.Collections.Generic;
using Microsoft.AspNetCore.Mvc;
namespace YourNamespace.Controllers
{
[ApiController]
[Route("[controller]")]
public class ProductController : ControllerBase
{
[HttpGet]
public IEnumerable<Product> Get() => ProductService.GetAll();
[HttpGet("{id}")]
public Product Get(int id) => ProductService.GetById(id);
[HttpPost]
public IActionResult Post([FromBody] Product product)
{
ProductService.Add(product);
return CreatedAtAction(nameof(Get), new { id = product.Id }, product);
}
[HttpPut("{id}")]
public IActionResult Put(int id, [FromBody] Product product)
{
ProductService.Update(id, product);
return NoContent();
}
[HttpDelete("{id}")]
public IActionResult Delete(int id)
{
ProductService.Delete(id);
return NoContent();
}
}
}
With these few commands, Cloving generates a complete ASP.NET Controller with standard CRUD methods.
Enhancing Controller Functionality
Interactively Refining Controller Logic
If you want to refine the logic further, use Cloving’s interactive features. Start a chat session to iterate over the generated code:
cloving chat -f Controllers/ProductController.cs
In the session, you might ask for enhancements:
Add validation to the POST method to ensure the product name is not empty
Cloving can provide suggestions or code changes, helping you build robust controllers.
Generating Unit Tests for Controllers
To ensure quality and reliability, generate unit tests for your controller using Cloving:
cloving generate unit-tests -f Controllers/ProductController.cs
This command creates unit tests specific to your controller methods, ensuring your logic is well-tested.
Example Unit Test
using Xunit;
using YourNamespace.Controllers;
using System.Linq;
public class ProductControllerTests
{
private readonly ProductController _controller;
public ProductControllerTests()
{
_controller = new ProductController();
}
[Fact]
public void Get_ReturnsAllProducts()
{
var result = _controller.Get();
Assert.NotEmpty(result);
}
[Fact]
public void Get_ReturnsProduct_ById()
{
var result = _controller.Get(1);
Assert.NotNull(result);
Assert.Equal(1, result.Id);
}
}
Leveraging AI for Code Reviews
Improve your code quality by generating AI-powered code reviews with Cloving:
cloving generate review
The generated review points out potential improvements, suggests optimizations, and ensures your code adheres to best practices.
Best Practices
- Initialize Projects: Use
cloving init
in every new project directory for optimal context generation. - Iterative Refinement: Use Cloving chat sessions for ongoing enhancements and clarifications.
- Code Quality: Regularly generate unit tests and reviews to maintain high-quality code.
- Version Control: Utilize
cloving commit
to generate informative commit messages with Cloving’s AI features.
Conclusion
The Cloving CLI offers C# developers a significant productivity boost by automating routine tasks and incorporating AI intelligence into the workflow. By mastering Cloving commands, you can generate ASP.NET Controllers swiftly and focus on developing more critical aspects of your applications. Embrace AI-powered automation with Cloving to elevate your development process and create robust, efficient code.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.