Automating API Integration in React with GraphQL Codegen with AI
Updated on July 01, 2024

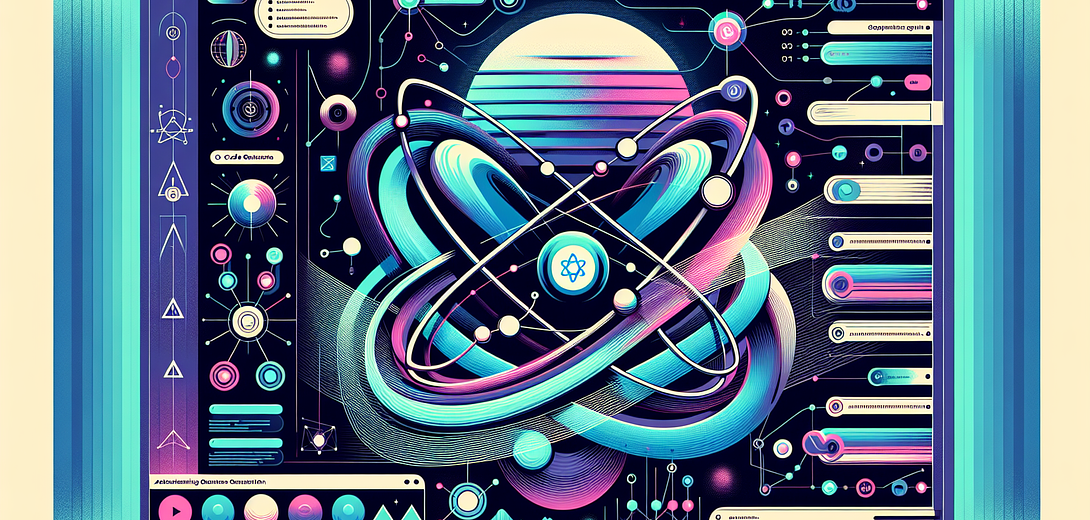
As a computer programmer, you likely know that integrating APIs with your React applications can be a complex and time-intensive task, especially when dealing with GraphQL. But what if you could streamline this process by combining your creativity with the powerful capabilities of artificial intelligence? This is where cloving comes in, allowing you to leverage AI tools like GPT to automate and enhance your workflow.
In this post, we’ll explore the concept of cloving and how you can use GPT for automating API integration in React with GraphQL Codegen, making your development tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. By integrating tools like GPT into your daily workflows, you can significantly improve your programming efficiency and effectiveness.
1. Setting Up GraphQL Codegen
GraphQL Codegen is a powerful tool that generates TypeScript typings and code based on your GraphQL schema. GPT can assist you in setting up and configuring GraphQL Codegen in your React project.
Example:
Suppose you’re starting a new React project and want to integrate GraphQL Codegen. You can prompt GPT for the initial setup steps:
How can I set up GraphQL Codegen in my React project?
GPT will provide you with detailed instructions, like the following:
- Install dependencies:
npm install @graphql-codegen/cli @graphql-codegen/typescript @graphql-codegen/typescript-operations @graphql-codegen/typescript-react-apollo @apollo/client graphql
- Create a configuration file:
touch codegen.yml
- Populate
codegen.yml
with basic configuration:generates: ./src/generated/graphql.tsx: plugins: - typescript - typescript-operations - typescript-react-apollo schema: "https://your-graphql-endpoint.com/graphql" documents: "./src/**/*.graphql"
2. Generating Types and Hooks
Once GraphQL Codegen is set up, it’s time to generate types and hooks to use in your React components. GPT can help automate this process by generating and suggesting the necessary GraphQL queries and mutations.
Example:
If you need a query to fetch user data, you can ask GPT:
Generate a GraphQL query to fetch user data by ID, and show how to use the generated hooks in a React component.
GPT will provide you with a query and a sample React component:
# src/queries/getUser.graphql
query GetUser($id: ID!) {
user(id: $id) {
id
name
email
}
}
// src/components/UserProfile.tsx
import React from 'react';
import { useGetUserQuery } from '../generated/graphql';
interface UserProfileProps {
userId: string;
}
const UserProfile: React.FC<UserProfileProps> = ({ userId }) => {
const { data, loading, error } = useGetUserQuery({ variables: { id: userId } });
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const user = data?.user;
return user ? (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
) : null;
};
export default UserProfile;
3. Debugging and Error Handling
AI can help you catch errors and suggest fixes. By analyzing your code, GPT can identify potential issues and offer solutions.
Example:
Suppose you encounter an error with your GraphQL query. You can describe the issue to GPT:
I'm getting a GraphQL error that says 'Cannot query field "email" on type "User". Here's the query: [code snippet]. How can I fix this?
GPT will analyze your code and suggest solutions, such as verifying the GraphQL schema or correcting the field names.
4. Optimizing Performance
GPT can help you optimize the performance of your GraphQL queries and suggest best practices to minimize latency and improve user experience.
Example:
You want to optimize a query to fetch a list of users with their latest posts. You can ask GPT:
How can I optimize a GraphQL query to fetch a list of users with their latest posts?
GPT will provide you with an optimized query and best practices, such as batching multiple requests and using data loaders:
query GetUsersWithPosts {
users {
id
name
posts(limit: 1, sort: "desc") {
id
title
content
}
}
}
5. Documentation and Explanation
Understanding and documenting your GraphQL schema and the generated code is crucial. GPT can assist by generating clear explanations and documentation for your queries and mutations.
Example:
When you need to document a complex query, you can ask GPT:
Generate documentation for this GraphQL query: [code snippet].
GPT will create detailed documentation, making it easier for you and your team to understand and maintain the code.
### GetUsersWithPosts Query
Fetches a list of users along with their most recent posts.
**Arguments:**
- `limit` (int): The number of posts to fetch per user.
- `sort` (string): The order to sort posts by (e.g., "desc" for descending).
**Response:**
- `users` (array): List of users.
- `id` (ID): Unique user identifier.
- `name` (string): User's name.
- `posts` (array): List of posts.
- `id` (ID): Unique post identifier.
- `title` (string): Title of the post.
- `content` (string): Content of the post.
Conclusion
By incorporating the principles of cloving, you can enhance your API integration process in React with GraphQL Codegen. Leveraging GPT allows you to automate code generation, optimize performance, and maintain clear documentation, ultimately transforming your development experience.
Bonus Follow-Up Prompts
Here are a few extra prompts to refine and enhance your workflow:
How can I configure GitHub Actions to run these code generation scripts and tests automatically?
Generate mock data for the user query to use in my tests.
What are other GPT prompts I could use to make this GraphQL integration more efficient?
Embrace cloving and discover how the symbiotic relationship between human creativity and AI capabilities can revolutionize your programming workflow.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.