AI-Powered Swift Code Optimization for Advanced iOS Development
Updated on March 07, 2025

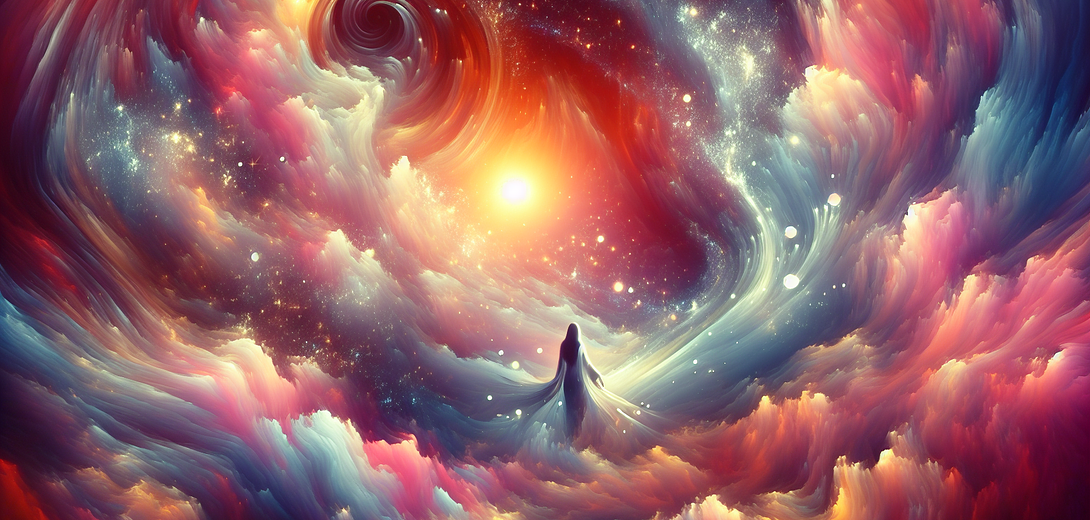
Enhancing Swift Code with Cloving CLI for Advanced iOS Applications
Swift has become the cornerstone of iOS development, balancing performance and readability to build robust applications. Yet as your codebase grows, maintaining optimal performance, clarity, and reliability can be challenging. Cloving CLI, an AI-powered command-line interface, streamlines your workflow by integrating GPT-based intelligence directly into your Swift project. From optimizing data parsing to generating thorough unit tests, Cloving can significantly boost productivity and code quality. Let’s explore how Cloving CLI can transform your iOS development with Swift.
1. Introduction to Cloving CLI
Cloving CLI is a powerful AI-driven tool designed to:
- Generate Swift code, ranging from entire classes to smaller utility methods
- Review existing code for optimizations
- Refine your code via an interactive chat environment
- Automate routine tasks, such as crafting commit messages
By recognizing your project’s context, Cloving ensures that generated or refactored code aligns with your architecture and development standards.
2. Getting Started with Cloving
2.1 Installation
Install Cloving globally with npm:
npm install -g cloving@latest
2.2 Configuration
Run Cloving’s configuration command:
cloving config
You’ll be prompted to provide your API key and select an AI model (e.g., GPT-3.5, GPT-4). This step is crucial to connecting Cloving to the AI backend.
3. Initializing Your Swift Project
To tailor AI suggestions to your codebase, navigate to your iOS or Swift project’s root and run:
cloving init
Cloving analyzes your folder structure, code style, and existing Swift files. It creates a cloving.json
with metadata, enabling more relevant outputs.
4. Generating and Optimizing Swift Code
4.1 Example: Optimizing a JSON Parser
Imagine you have a Swift function that parses JSON data for your iOS application, but you suspect it might be suboptimal or lacking clarity. Cloving can generate a refined version of this code:
cloving generate code --prompt "Optimize JSON data parsing for a Swift iOS app" --files Models/JsonParser.swift
Sample Output:
// Models/JsonParser.swift
import Foundation
func optimizedParseJSONData(from data: Data) -> Result<YourModel, Error> {
do {
let decoder = JSONDecoder()
decoder.keyDecodingStrategy = .convertFromSnakeCase
let result = try decoder.decode(YourModel.self, from: data)
return .success(result)
} catch {
return .failure(error)
}
}
Notes:
- Cloving automatically includes best practices (e.g.,
.convertFromSnakeCase
) for JSON decoding. - If your code references a
YourModel
struct or uses certain date decoding strategies, mention them in your prompt for more specialized generation.
4.2 Enhancing or Revising Generated Code
If you need more advanced features—like error handling for missing fields or custom date decoders—ask Cloving to refine it:
Revise the JSON parser to handle missing keys gracefully
Cloving updates the snippet, perhaps returning a default value or logging a warning for missing keys.
5. Reviewing and Refining Swift Code
5.1 Interactive Chat Mode
For advanced or iterative tasks, rely on Cloving chat:
cloving chat -f Controllers/ViewController.swift
Inside the chat environment, you can:
- Ask for concurrency improvements (e.g., using
async/await
or GCD for background tasks) - Refine existing logic to reduce complexity
- Explore performance considerations in areas like table views, collection views, or data transformations
Example:
cloving> Optimize the data fetching method in ViewController for concurrency
Cloving can propose an async method or a background queue approach, along with best practices in Swift concurrency.
5.2 Code Review
Use Cloving’s generate review feature to identify potential pitfalls or best practice deviations:
cloving generate review
Cloving scans your code for possible performance issues, stylistic inconsistencies, or unhandled errors, offering recommended improvements.
6. Generating Unit Tests for Swift
6.1 Example: Unit Testing a JSON Parser
To ensure reliability, prompt Cloving to produce initial tests:
cloving generate unit-tests -f Models/JsonParser.swift
Sample Output:
// Models/JsonParserTests.swift
import XCTest
@testable import YourApp
class JsonParserTests: XCTestCase {
func testOptimizedParseJSONDataSuccess() throws {
let jsonString = """
{
"id": 1,
"name": "Test Model"
}
"""
guard let data = jsonString.data(using: .utf8) else {
XCTFail("Failed to convert jsonString to Data")
return
}
let result = optimizedParseJSONData(from: data)
switch result {
case .success(let model):
XCTAssertEqual(model.id, 1)
XCTAssertEqual(model.name, "Test Model")
case .failure:
XCTFail("Expected parse success, got failure")
}
}
func testOptimizedParseJSONDataFailure() throws {
let invalidData = Data()
let result = optimizedParseJSONData(from: invalidData)
switch result {
case .success:
XCTFail("Expected parse failure, got success")
case .failure(let error):
XCTAssertNotNil(error)
}
}
}
Notes:
- Replace
YourApp
andYourModel
references with your actual module and data type. - Expand the tests for negative scenarios (missing fields, partial data, etc.) or concurrency edge cases if your code is asynchronous.
7. Managing Commits with Cloving
7.1 Generating Commit Messages
Ensure clarity in your commit history by letting Cloving propose commit messages:
cloving commit
Cloving examines staged changes (e.g., new or updated Swift files, tests) and suggests a succinct message:
Optimize JSON parsing in Swift and add coverage with unit tests
You can accept or refine it before finalizing the commit.
8. Best Practices for AI-Driven Swift Enhancements
- Initialize in Each Project
If you manage multiple iOS apps, runcloving init
in each directory to ensure Cloving recognizes each codebase’s structure. - Iterate
For complex tasks (e.g., advanced concurrency, complex data models), rely on multiple prompts or the interactive chat to refine code over time. - Review
Usecloving generate review
to maintain high code quality, scanning for potential memory leaks, unhandled errors, or performance pitfalls. - Test Thoroughly
AI-generated tests are a starting point. Expand them to handle concurrency, edge cases (like nil objects), and special device behaviors. - Maintain Conventions
Swift style guidelines (like the SwiftLint rules or Apple recommended naming) can be enforced by referencing them in your prompts, ensuring consistent results.
9. Example Workflow
Here’s a typical approach to using Cloving for Swift code enhancement:
- Initialize:
cloving init
at the root of your iOS project. - Generate: e.g.
cloving generate code --prompt "Optimize JSON data parsing for a Swift iOS app" --files Models/JsonParser.swift
. - Test:
cloving generate unit-tests -f Models/JsonParser.swift
for coverage. - Refine:
cloving chat -f Controllers/ViewController.swift
to integrate concurrency or advanced logic. - Review:
cloving generate review
to scan for improvements in your codebase. - Commit: Summarize your changes with
cloving commit
.
10. Conclusion
By leveraging Cloving CLI in your Swift development workflow, you can offload routine code generation and optimization tasks to AI, freeing your team to focus on crafting innovative iOS features. Cloving’s GPT-based intelligence ensures the code it produces or refines is relevant to your context, improving performance and maintainability across your codebase.
Remember: Cloving’s outputs serve as a foundation – always review them for domain-specific correctness, performance constraints, and style guidelines. By merging your expertise with AI-driven suggestions, you achieve a robust, forward-thinking development approach for advanced iOS applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.