AI-Powered Refactoring for Vue.js Codebases
Updated on July 01, 2024

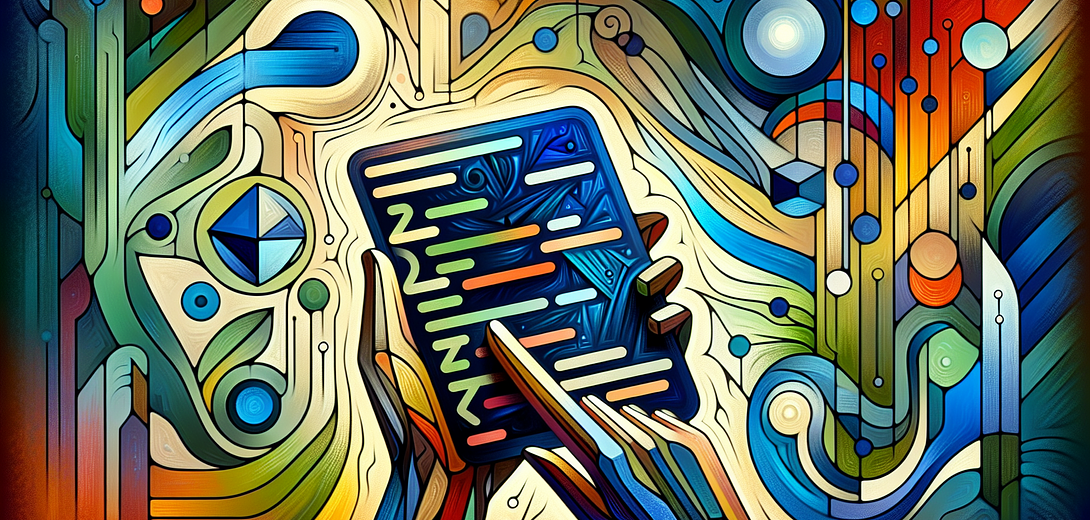
In the ever-evolving world of software development, keeping your codebase clean and maintainable can often feel like an uphill battle, especially with frameworks like Vue.js that have many moving parts. But what if there was a way to harness both human creativity and AI’s analytical prowess to make this task easier? Enter cloving—the integration of human and machine efforts to achieve a common goal more efficiently and effectively.
In this blog post, we will explore how to incorporate AI tools like GPT into your daily workflow to refactor Vue.js codebases, making your programming tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI capabilities to streamline problem-solving processes. This collaborative approach between humans and AI can significantly enhance productivity and efficiency, especially in refactoring complex codebases.
1. Automated Code Analysis and Refactoring
AI can quickly analyze your Vue.js code and identify areas in need of refactoring. By providing suggestions on improving code structure, AI tools like GPT can help maintain a cleaner codebase.
Example:
Suppose you have a Vue.js component with numerous nested elements and methods. You can describe the component to GPT and ask for refactoring recommendations:
I have a Vue.js component with deeply nested elements and numerous methods. Here's the component code:
<template>
<div>
<div v-if="showHeader">
<h1 @click="toggleHeader">{{ title }}</h1>
</div>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
showHeader: true,
title: "My Todo List",
items: ["Task 1", "Task 2", "Task 3"]
};
},
methods: {
toggleHeader() {
this.showHeader = !this.showHeader;
}
}
};
</script>
Can you suggest ways to refactor it?
GPT will analyze the component and provide suggestions such as breaking down the component into smaller, reusable components or optimizing methods.
2. Generating Optimized Code Snippets
Writing optimized code is an essential part of refactoring. GPT can assist you in generating more efficient code snippets based on your specific requirements.
Example:
If you need to optimize a method that fetches data from an API and updates the state, you can prompt GPT:
Optimize this Vue.js method that fetches data from an API and updates the state:
methods: {
async fetchData() {
try {
const response = await axios.get("/api/todos");
this.data = response.data;
} catch (error) {
console.error("Error fetching data:", error);
}
}
}
GPT will suggest a more efficient version of the method, reducing potential bottlenecks and improving overall performance.
3. Learning and Adapting Best Practices
Adhering to best practices is crucial for maintainable code. GPT can help you stay updated with the latest best practices in Vue.js development.
Example:
To understand the best practices for Vue.js component design, you can ask GPT:
What are the best practices for designing maintainable and efficient Vue.js components in 2024?
GPT will provide a list of best practices, ensuring that your code adheres to industry standards.
4. Assistive Debugging and Error Resolution
Identifying and resolving errors can be time-consuming. GPT can help you debug and fix errors by analyzing your code and suggesting potential solutions.
Example:
If you encounter a bug in your Vue.js application, you can describe the issue to GPT:
My Vue.js app is throwing an error: 'Cannot read property 'data' of undefined' in this component:
<template>
<div>
<h1>{{ data.title }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
data: null
};
},
mounted() {
this.fetchData();
},
methods: {
fetchData() {
axios.get("/api/todos")
.then(response => {
this.data = response.data;
})
.catch(error => {
console.error("Error fetching data:", error);
});
}
}
};
</script>
How can I fix this?
GPT will analyze the error and suggest possible fixes, such as checking for null or undefined values before accessing properties.
5. Automating Unit and E2E Test Generation
Testing is a critical part of refactoring, but writing tests can be tedious. GPT can help automate the creation of unit and E2E tests for your Vue.js components.
Example:
If you have refactored a component and need to generate tests for it, you can prompt GPT:
Generate unit tests for this Vue.js component using Jest:
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ["Task 1", "Task 2", "Task 3"]
};
}
};
</script>
GPT will generate a set of unit tests, ensuring your component works as expected and helping you catch any potential issues early.
6. Generating Documentation
Clear documentation is essential for maintaining a refactored codebase. GPT can help you generate detailed documentation for your Vue.js components.
Example:
When documenting a complex component, you can ask GPT:
Generate documentation for this Vue.js component:
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ["Task 1", "Task 2", "Task 3"]
};
}
};
</script>
GPT will create thorough documentation, making it easier for you and your team to understand and maintain the code.
7. Generating Tailwind Styling for Components
Tailwind CSS is a popular utility-first CSS framework that can help you design your Vue.js components more efficiently. GPT can assist you in generating Tailwind CSS styles for your components, ensuring they look great and stay consistent.
Example:
If you want to style a component using Tailwind CSS, you can prompt GPT:
Generate Tailwind CSS styles for this Vue.js component:
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ["Task 1", "Task 2", "Task 3"]
};
}
};
</script>
GPT will provide the component with Tailwind CSS classes:
<template>
<div class="p-4 bg-gray-100 rounded-lg">
<ul class="list-disc list-inside">
<li v-for="(item, index) in items" :key="index" class="py-1 px-2 bg-white shadow-sm rounded-md">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ["Task 1", "Task 2", "Task 3"]
};
}
};
</script>
By adding utility classes from Tailwind CSS, your component will have a clean and responsive design with minimal effort.
Conclusion
AI-powered refactoring for Vue.js codebases exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. By integrating GPT into your refactoring workflow, you can enhance your productivity, reduce errors, and maintain high standards in your codebase. Embrace cloving to discover how this synergistic approach can transform your programming experience.
Bonus Follow-Up Prompts
Here are a few extra prompts you could use to further aid your refactoring process:
How can I configure GitHub to run automated tests for these refactored components?
Generate accompanying test data factories for the refactored Vue.js components.
What are other GPT prompts I could use to make Vue.js refactoring more efficient?
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.