Adding i18n To A React App with GPT's Help
Updated on July 03, 2024

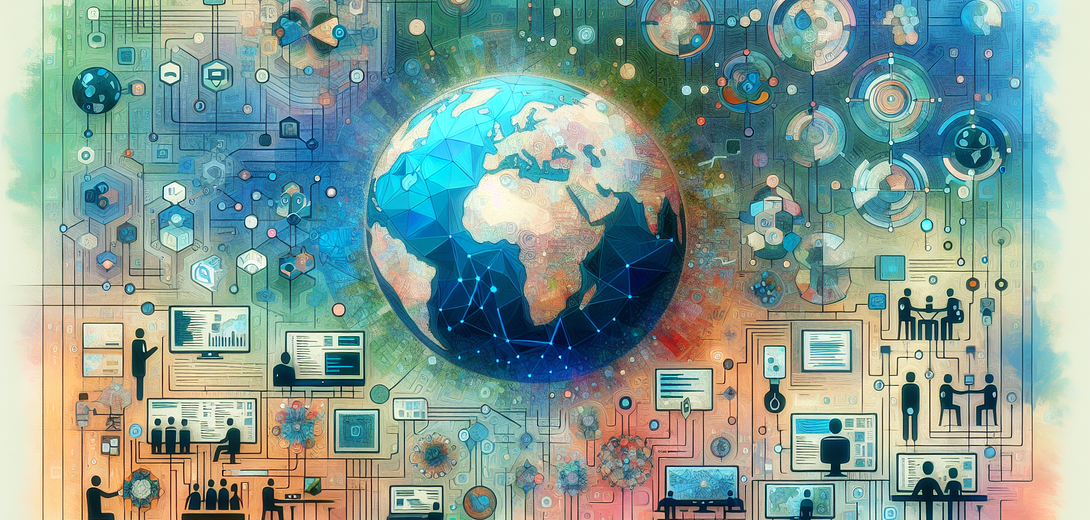
Internationalization (i18n) is essential for creating applications that cater to a global audience. By incorporating human creativity and the robust AI capabilities of GPT, programmers can make their i18n process for React apps more efficient and effective. In this post, we’ll explore how you can integrate GPT into your daily workflow to streamline the internationalization process.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. It’s not just about using AI tools; it’s about creating a symbiotic relationship where human and machine strengths are leveraged to solve problems more effectively.
1. Setting Up i18n in a React App
The first step in adding i18n to a React application is to set up the necessary libraries and configuration. GPT can help you get started by providing clear instructions and sample code.
Example:
You can ask GPT for a step-by-step guide to set up i18n in your React app:
How do I set up i18n for a React application?
GPT will provide the following instructions:
-
Install the necessary package:
npm install i18next react-i18next i18next-http-backend i18next-browser-languagedetector
-
Initialize i18next:
import i18n from 'i18next'; import { initReactI18next } from 'react-i18next'; import HttpApi from 'i18next-http-backend'; import LanguageDetector from 'i18next-browser-languagedetector'; i18n .use(HttpApi) .use(LanguageDetector) .use(initReactI18next) .init({ fallbackLng: 'en', detection: { order: ['queryString', 'cookie', 'localStorage', 'navigator', 'htmlTag', 'path', 'subdomain'], caches: ['cookie'], }, backend: { loadPath: '/locales/{{lng}}/{{ns}}.json', }, }); export default i18n;
2. Generating Translation Files
Creating translation files manually can be tedious. GPT can automate this process by generating JSON files for different languages based on your text content.
Example:
If you need Spanish translations for your app, prompt GPT:
Generate a Spanish translation file for the following English text content in JSON format: {"welcome": "Welcome", "logout": "Logout"}
GPT will provide a JSON file like this:
{
"welcome": "Bienvenido",
"logout": "Cerrar sesión"
}
You can save this content in a file named es.json
under a locales
directory.
3. Implementing Translation in Components
After setting up i18n and generating translation files, you need to update your components to use these translations. GPT can help you refactor your code to integrate i18n.
Example:
If you have a component that displays a welcome message and logout button, you can ask GPT how to use i18n in this component:
How can I use i18n to translate text in my React component? Here's the existing component code:
import React from 'react';
const WelcomeComponent = () => (
<div>
<h1>Welcome</h1>
<button>Logout</button>
</div>
);
export default WelcomeComponent;
GPT will suggest the following changes:
import React from 'react';
import { useTranslation } from 'react-i18next';
const WelcomeComponent = () => {
const { t } = useTranslation();
return (
<div>
<h1>{t('welcome')}</h1>
<button>{t('logout')}</button>
</div>
);
};
export default WelcomeComponent;
4. Automating Testing for i18n
Testing is crucial to ensure that the translations are working correctly. GPT can help automate the creation of test cases for i18n.
Example:
You can ask GPT for help in creating Jest test cases for your WelcomeComponent
.
Generate Jest test cases for the i18n implementation in the WelcomeComponent.
GPT will generate:
import React from 'react';
import { render } from '@testing-library/react';
import { I18nextProvider } from 'react-i18next';
import i18n from 'i18next';
import WelcomeComponent from './WelcomeComponent';
import Backend from 'i18next-http-backend';
import LanguageDetector from 'i18next-browser-languagedetector';
import { initReactI18next } from 'react-i18next';
i18n
.use(Backend)
.use(LanguageDetector)
.use(initReactI18next)
.init({
fallbackLng: 'en',
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
},
});
test('renders welcome message and logout button', () => {
const { getByText } = render(<I18nextProvider i18n={i18n}><WelcomeComponent /></I18nextProvider>);
expect(getByText('Welcome')).toBeInTheDocument();
expect(getByText('Logout')).toBeInTheDocument();
});
5. Extracting Text Components into i18n Files with GPT
A great way to streamline your workflow is to use GPT to extract text components from your React components and generate corresponding i18n translation files. This can save you the time and effort of manually extracting and creating key-value pairs for translations.
Here is an example of how GPT can help you in this process:
Example:
You have a simple React component and you want to extract its text content into an i18n file.
// WelcomeComponent.js
import React from 'react';
const WelcomeComponent = () => (
<div>
<h1>Welcome</h1>
<button>Logout</button>
</div>
);
export default WelcomeComponent;
You can prompt GPT as follows:
Extract the text from the following React component and generate an i18n JSON file for it. Here's the existing component code:
import React from 'react';
const WelcomeComponent = () => (
<div>
<h1>Welcome</h1>
<button>Logout</button>
</div>
);
export default WelcomeComponent;
GPT will provide the following i18n JSON file:
// en.json
{
"welcome": "Welcome",
"logout": "Logout"
}
GPT will also suggest the necessary changes to the React component to use the i18n translations:
// WelcomeComponent.js
import React from 'react';
import { useTranslation } from 'react-i18next';
const WelcomeComponent = () => {
const { t } = useTranslation();
return (
<div>
<h1>{t('welcome')}</h1>
<button>{t('logout')}</button>
</div>
);
};
export default WelcomeComponent;
Conclusion
Integrating GPT into your daily workflow for adding i18n to a React app can make the process more streamlined and less error-prone. By leveraging cloving, you can combine human ingenuity with AI’s efficiency to create applications that cater to a global audience seamlessly.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.