Accelerating Swift Code Writing with GPT's Guidance
Updated on January 01, 2025

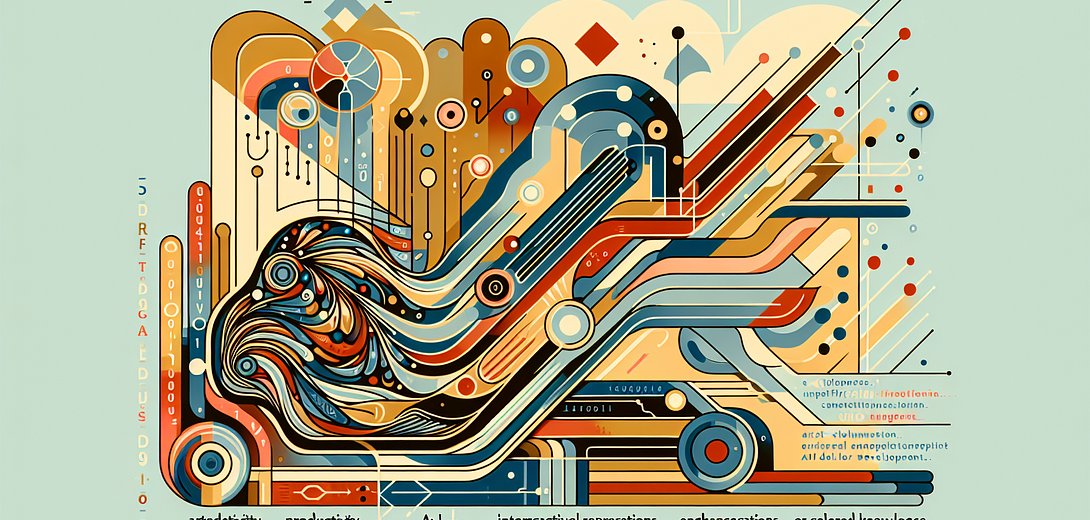
Swift is a powerful programming language that offers developers the ability to write expressive and efficient code. However, even the most skilled programmers can benefit from an AI-powered assistant to accelerate their coding workflow. Enter the Cloving CLI tool—a command-line interface that integrates AI into your development process, enhancing productivity and code quality. In this blog post, we’ll delve into how you can leverage Cloving CLI to speed up your Swift code writing, highlighting practical examples and best practices.
Setting Up Cloving for Swift Development
Before harnessing the power of Cloving, you’ll need to set it up within your Swift project environment.
Installation
First, install Cloving globally via npm:
npm install -g cloving@latest
Configuration
Next, configure Cloving with your API key and preferred AI model:
cloving config
Follow the prompts to input your API key and select an AI model that fits your needs.
Initializing Your Swift Project
To kick off your Cloving experience within your Swift project, initialize it in your directory:
cd your-swift-project
cloving init
This command will create a cloving.json
that stores project context, ensuring Cloving understands your project’s nuances and dependencies.
Generating Swift Code with Cloving
One of Cloving’s standout features is its ability to generate code snippets tailored to your prompts. Here’s how you can use it for Swift development.
Example: Creating a Swift Function
Let’s say you’re tasked with writing a Swift function for calculating the factorial of a number. Use the cloving generate
command to assist you:
cloving generate code --prompt "Write a Swift function to calculate the factorial of an integer" --files path/to/yourfile.swift
The tool will take your context into account and generate efficient Swift code:
func factorial(n: Int) -> Int {
if n < 0 { return -1 }
if n == 0 { return 1 }
return n * factorial(n - 1)
}
Enhancing Generated Code
After generation, Cloving allows you to revise or enhance the code further within an interactive prompt. You can request additional features or optimizations:
Revise the factorial function to handle input as an optional
Based on this request, GPT might modify the code as follows:
func factorial(n: Int?) -> Int {
guard let n = n else { return -1 }
if n < 0 { return -1 }
if n == 0 { return 1 }
return n * factorial(n: n - 1)
}
Cloving’s Code Review and Unit Test Features
A well-crafted codebase requires thorough testing and review, both of which Cloving can facilitate.
Generating Unit Tests
For testing, utilize Cloving to generate unit tests for your Swift code:
cloving generate unit-tests -f path/to/yourfile.swift
This command yields useful unit test cases that enhance your code’s reliability:
import XCTest
@testable import YourModule
class FactorialTests: XCTestCase {
func testFactorialOfPositiveNumber() {
XCTAssertEqual(factorial(n: 5), 120)
}
func testFactorialOfZero() {
XCTAssertEqual(factorial(n: 0), 1)
}
func testFactorialWithNil() {
XCTAssertEqual(factorial(n: nil), -1)
}
func testFactorialOfNegativeNumber() {
XCTAssertEqual(factorial(n: -5), -1)
}
}
Performing Code Reviews
Conducting AI-powered code reviews is simple with Cloving:
cloving generate review
This feature helps you identify potential improvements or issues in your Swift code, contributing to better code quality.
Using Cloving Chat for Real-Time Assistance
For more complex Swift tasks or ongoing guidance, engage with Cloving’s chat functionality:
cloving chat -f path/to/yourfile.swift
During the chat session, you can ask for explanations, examples, or even new Swift code snippets.
Best Practices for Swift Development with Cloving
- Configure Properly: Ensure Cloving is set up with the correct context for your Swift project to maximize code relevance.
- Interactive Revisions: Use the interactive prompt to tweak generated code, achieving the desired functionality.
- Leverage Unit Tests: Automatically generated tests are crucial for maintaining robust code.
- Explore Chat Functionality: For complex implementations, ongoing chat support can provide in-depth help and advice.
Conclusion
Cloving CLI is a transformative tool for Swift programmers, allowing you to write and maintain high-quality code more efficiently. By integrating AI into your development process, you can focus on creative programming tasks while Cloving handles repetitive and complex elements. Embrace the Cloving CLI and elevate your Swift coding experience today.
Remember, Cloving is an assistant designed to enhance, not replace, your expertise. Use it to augment your coding skills and speed up your workflow.
Whether you’re new to Swift or an experienced developer, Cloving CLI offers an invaluable addition to your toolkit. Start accelerating your Swift code writing with Cloving today!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.