Accelerating RESTful API Development in Python with GPT
Updated on November 28, 2024

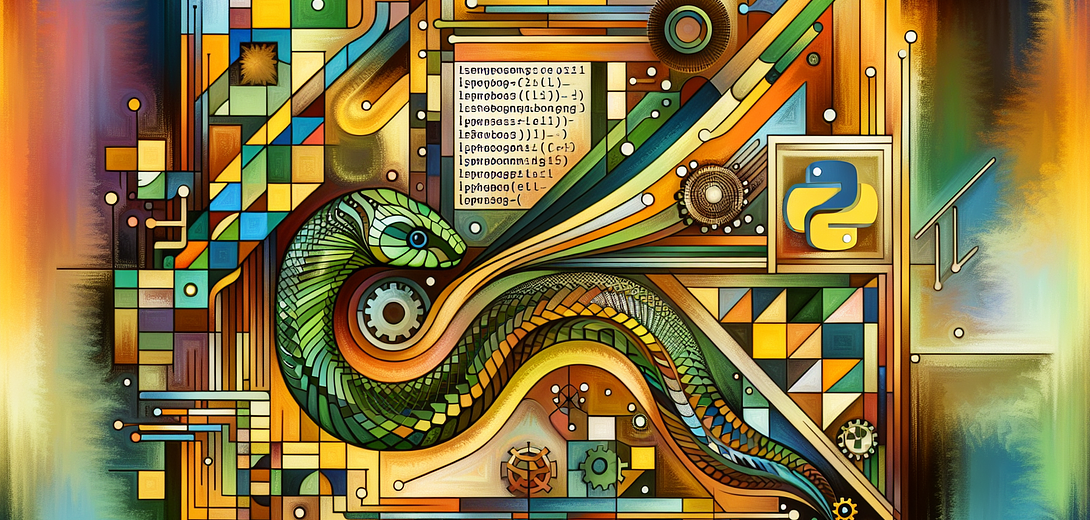
In the modern landscape of software development, building RESTful APIs efficiently is crucial. By leveraging AI tools like the Cloving CLI, developers can enhance their productivity and code quality, making the development process smoother and more efficient. In this tutorial, we’ll explore how Python developers can utilize Cloving CLI for RESTful API development, reducing repetitive tasks and allowing more focus on the functionality and quality of their applications.
Getting Started with Cloving CLI
Step 1: Install and Configure Cloving
Before accelerating your RESTful API development, make sure Cloving is installed and configured:
Installation:
Use npm to install Cloving globally:
npm install -g cloving@latest
Configuration:
Run the config
command to set up your API key and preferred models.
cloving config
Follow the prompts to input your API key and choose the AI model you’d like to use.
Step 2: Initialize Your Python Project
To leverage Cloving properly, initialize your project in the current directory. This enables Cloving to understand the structure and context of your application.
cloving init
This command generates a cloving.json
file containing metadata about your project, such as its dependencies, language, and framework.
Using Cloving for API Development
1. Generate Python Code Snippets
Cloving CLI can generate code snippets based on prompts. Whether you need to define a Flask route or a SQLAlchemy model, Cloving’s AI capabilities can assist:
Example:
Suppose you’re developing a Flask API and need to create a new route for a resource, such as handling a POST
request to add a new user.
cloving generate code --prompt "Create a Flask route to add a new user to the database" --files app.py
The AI will analyze the context and generate relevant code:
@app.route('/user', methods=['POST'])
def add_user():
data = request.get_json()
new_user = User(name=data['name'], email=data['email'])
db.session.add(new_user)
db.session.commit()
return jsonify({'message': 'User added successfully'}), 201
2. Interactive Development with Cloving Chat
For complex tasks or ongoing assistance, use the interactive chat feature. This is particularly valuable for discussing architectural decisions or brainstorming solutions.
cloving chat --files app.py
Inside the interactive session, you might ask a question like, “How can I implement error handling for the add_user function to manage exceptions such as duplicate email entries?” Here’s a sample GPT response with revised code:
@app.route('/user', methods=['POST'])
def add_user():
try:
data = request.get_json()
new_user = User(name=data['name'], email=data['email'])
db.session.add(new_user)
db.session.commit()
return jsonify({'message': 'User added successfully'}), 201
except IntegrityError as e:
db.session.rollback()
return jsonify({'error': 'Email already exists!'}), 400
This demonstrates GPT’s ability to provide useful, context-aware code suggestions that improve your application that is delivered to you on a silver platter by the Cloving CLI. Integrating this change is as easy as a “save” command and if you like the code that’s been generated you can follow it up with a “commit” command to easily generate an automagically GPT generated comprehensive commit message based on the changes made to the code.
3. Automated Unit Test Generation
To ensure your API functions correctly, Cloving can generate unit tests for your endpoints:
cloving generate unit-tests --files tests/test_user.py
Example output for a test_user
function:
def test_add_user(client):
response = client.post('/user', json={'name': 'John Doe', 'email': '[email protected]'})
assert response.status_code == 201
assert response.json['message'] == 'User added successfully'
4. Code Reviews to Maintain Quality
Use Cloving to perform AI-driven code reviews, highlighting potential errors or improvements for your RESTful API:
cloving generate review --files app.py
This command generates a detailed review:
# Code Review Summary
## Key Changes & Suggestions
1. **Database Transactions:** Ensure transactions are properly handled. Consider using context managers for DB operations.
2. **Input Validation:** Validate incoming data in the `add_user` endpoint to prevent unwanted data entry.
3. **Error Handling:** Implement error handling for database operations to manage exceptions such as unique constraint violations.
Recognizing and resolving these issues can help maintain the reliability and safety of the API.
Best Practices and Tips for Cloving CLI
-
Use Contextual Files: Always specify relevant files with the
-f
option to provide more context, enabling Cloving to generate precise code suited to your specific project. -
Interactive Prompting: When generating code, experiment using interactive mode with the
--interactive
flag. This allows for iterative development where you can receive, adjust, and save code snippets as needed. -
Continuous Improvement: Utilize the
generate review
command to constantly monitor and improve code quality by identifying potential issues early. -
Merge with Git: After Cloving assists in writing your code, use Cloving to generate commit messages. This ensures that your project’s history is both informative and consistent.
cloving commit
Conclusion
By incorporating Cloving CLI into your RESTful API development workflow, you can boost efficiency, reduce common programming errors, and produce more robust Python applications. As you continue to leverage Cloving’s AI capabilities, your capabilities as a developer will likewise grow—enabling you to take on bigger projects with confidence.
Cloving isn’t here to replace your skills but to augment them, empowering you to focus on the innovative parts of your projects. Embrace it and accelerate your development with the aid of AI!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.