Accelerating Java Code Generation with GPT's Assistance
Updated on November 28, 2024

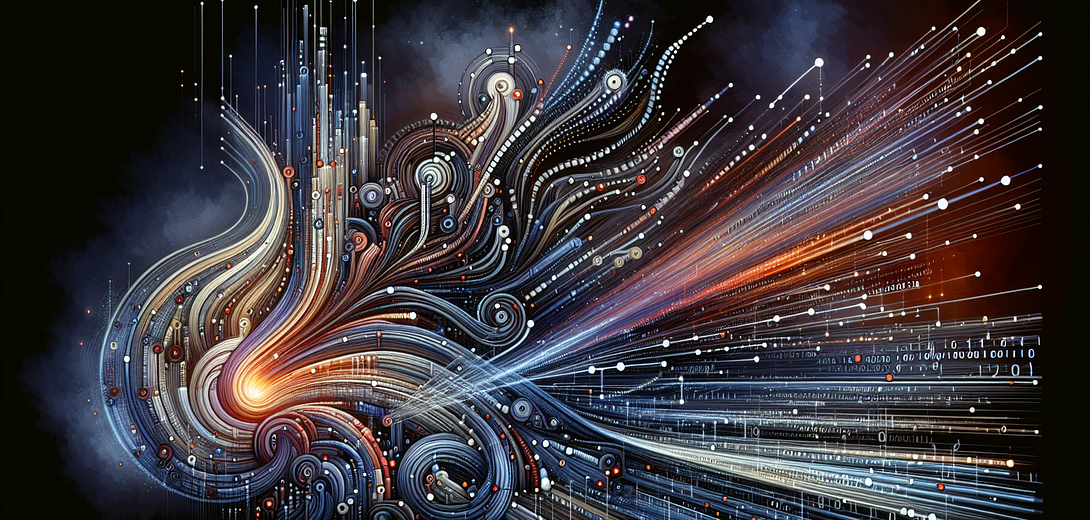
In the realm of software development, speed and efficiency are crucial. The Cloving CLI tool, powered by AI, streamlines the coding process and boosts productivity, which is especially useful when generating code for Java projects. This tutorial will walk you through the essential steps to accelerate your Java code generation using Cloving, making your development experience more efficient.
Setting Up Cloving
Before you can start generating Java code with Cloving, you need to ensure it’s properly set up in your environment.
Installation
First, install Cloving globally via npm:
npm install -g cloving@latest
Configuration
Next, configure Cloving with your AI model settings:
cloving config
During this process, you’ll enter your API key and select the preferred AI models which will guide the code generation process, ensuring contextually relevant responses.
Initializing Your Java Project
For Cloving to efficiently generate Java code, ensure it’s initialized in the project directory:
cloving init
This command sets up Cloving by analyzing your project and creating a cloving.json
file, effectively capturing your project’s metadata.
Generating Java Code
With Cloving seamlessly set up, it’s time to explore how you can efficiently generate Java code snippets with Cloving’s help.
Generating Java Functions
Let’s say you’re working on a Java project and need a basic utility function, like calculating the factorial of a number:
cloving generate code --prompt "Write a Java function to calculate the factorial of a number" --files src/utils/MathUtils.java
Cloving will utilize the project context and generate a suitable code snippet:
// src/utils/MathUtils.java
public class MathUtils {
public static long factorial(int number) {
if (number < 0) {
throw new IllegalArgumentException("Number must be non-negative.");
}
return number <= 1 ? 1 : number * factorial(number - 1);
}
}
Revising and Reviewing Code
Once Cloving generates the code, you have several options:
- Revise: Request improvements or modifications to the code.
- Explain: Ask for detailed explanations of the generated code.
- Save: Automatically save your generated code to specified files.
For example, request a revision to handle input validation more robustly:
Please add input validation to ensure the number is positive
The revised code snippet might look like this:
// src/utils/MathUtils.java
public class MathUtils {
public static long factorial(int number) {
if (number < 0) {
throw new IllegalArgumentException("Number must be non-negative.");
}
if (number == null || number.isNaN()) {
throw new IllegalArgumentException("Input must be a valid number.");
}
return number <= 1 ? 1 : number * factorial(number - 1);
}
}
Generating Unit Tests
Cloving also assists in bolstering your code’s reliability through unit tests.
cloving generate unit-tests -f src/utils/MathUtils.java
This command generates corresponding unit test cases, which can then be added to your project:
// src/utils/MathUtilsTest.java
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class MathUtilsTest {
@Test
void testFactorial() {
assertEquals(1, MathUtils.factorial(0));
assertEquals(1, MathUtils.factorial(1));
assertEquals(120, MathUtils.factorial(5));
}
@Test
void testFactorialNegativeNumber() {
assertThrows(IllegalArgumentException.class, () -> MathUtils.factorial(-1));
}
}
Utilizing Cloving Chat for Complex Tasks
For intricate Java projects or specific coding challenges, leverage Cloving’s chat feature:
cloving chat -f src/Main.java
This begins an interactive session allowing you to ask for coding advice, interpret code snippets, or receive guidance on Java-specific concerns:
cloving> Can you help me create a method in Java to reverse a linked list?
Cloving will provide comprehensive assistance by generating code snippets and offering explanations as needed.
Enhancing Git Commits
Cloving simplifies crafting meaningful commit messages. Instead of writing your own, let Cloving help:
cloving commit
Cloving generates a context-aware commit message, maintaining coding standards and documentation quality.
Conclusion
Accelerating Java code generation with Cloving exemplifies a productive integration of AI into your development workflow. By following this guide, you will enhance your coding efficiency, reduce development time, and ensure higher code quality. Embrace the full potential of Cloving and let it transform how you generate Java code.
Harnessing AI with Cloving CLI: A Revolutionary Change for Java Code Developers
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.