Accelerating Full-Stack Development with GPT in Node.js
Updated on January 05, 2025

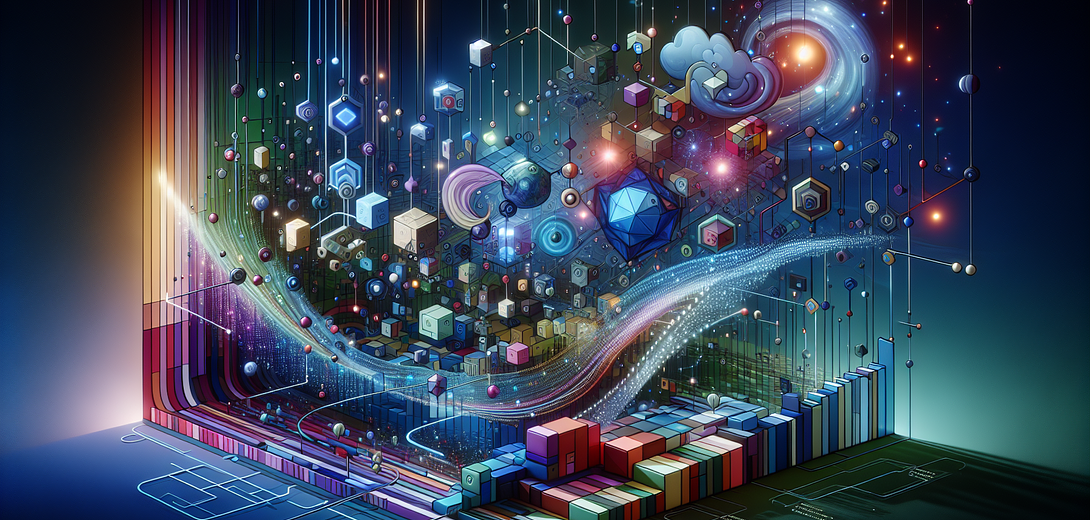
In the world of full-stack development, staying efficient and maintaining high-quality code is crucial. With AI-powered tools like Cloving CLI, developers can seamlessly integrate AI into their development process to accelerate their workflows. This blog post will guide you through using the Cloving CLI to optimize your full-stack development in Node.js, leveraging its powerful GPT models.
Introduction to Cloving CLI
Cloving CLI acts as an AI-powered pair-programmer that assists you in generating, reviewing, and enhancing code. By using Cloving’s commands, you can streamline your Node.js development tasks and improve the overall quality of your applications.
1. Getting Started with Cloving
To get started, you’ll need to install and configure Cloving in your development environment.
Installation:
Make sure you have Node.js and npm installed. Use the following command to install Cloving:
npm install -g cloving@latest
Configuration:
Set up Cloving by running:
cloving config
Follow the prompts to configure your API key and select your preferred GPT model.
2. Initializing Cloving in Your Project
Ensuring Cloving understands your project’s context is key to generating relevant code and reviews.
Command:
cloving init
This command initializes Cloving by creating a cloving.json
file, capturing metadata about your Node.js application and setting the default context for subsequent operations.
3. Generating Code for Node.js
Imagine you’re tasked with adding an authentication system to your application. Instead of starting from scratch, you can leverage Cloving to generate the necessary code quickly.
Example:
cloving generate code --prompt "Create an authentication middleware for Express.js" --files src/middleware/authMiddleware.js
This command will parse your existing codebase and generate an authentication middleware based on your current project structure. Here’s a sample snippet:
const jwt = require('jsonwebtoken');
require('dotenv').config();
function authenticateToken(req, res, next) {
const token = req.header('Authorization');
if (!token) return res.status(401).send('Access Denied');
try {
const verified = jwt.verify(token, process.env.TOKEN_SECRET);
req.user = verified;
next();
} catch (err) {
res.status(400).send('Invalid Token');
}
}
module.exports = authenticateToken;
4. Reviewing and Revising Code
After generating code, you can refine it using Cloving’s interactive chat feature.
$ cloving chat -f src/middleware/authMiddleware.js
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
What would you like to do?
cloving> Revise the middleware to support role-based access
You can refine the code by specifying additional requirements or revising parts of it until it fits your needs perfectly.
5. Generating Unit Tests
Unit tests are essential for maintaining code quality. Cloving can help generate comprehensive tests for your Node.js components.
Command:
cloving generate unit-tests -f src/middleware/authMiddleware.js
The above command results in test cases like this:
const request = require('supertest');
const express = require('express');
const authenticateToken = require('../src/middleware/authMiddleware');
const app = express();
app.use(authenticateToken);
describe('GET /', () => {
it('should return 401 if no token is provided', async () => {
await request(app)
.get('/')
.expect(401);
});
it('should return 400 for invalid token', async () => {
await request(app)
.get('/')
.set('Authorization', 'invalidtoken')
.expect(400);
});
});
6. Efficient Code Commits
Enhance your workflow with AI-generated commit messages to emphasize clarity and context.
cloving commit
Cloving will analyze changes in your code and suggest detailed commit messages that can further be refined by you.
Conclusion
Integrating GPT models into your Node.js development process using the Cloving CLI can significantly boost your productivity and code quality. Whether you are generating new features, revising current ones, or ensuring robust unit tests, Cloving provides a seamless experience. Use Cloving as a powerful ally to accelerate your full-stack development duties and produce applications that stand out in reliability and performance.
Embrace the future of development with Cloving CLI and shine in your full-stack Node.js projects!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.