Accelerating Frontend Development in Elm with GPT-guided Code Generation
Updated on March 05, 2025

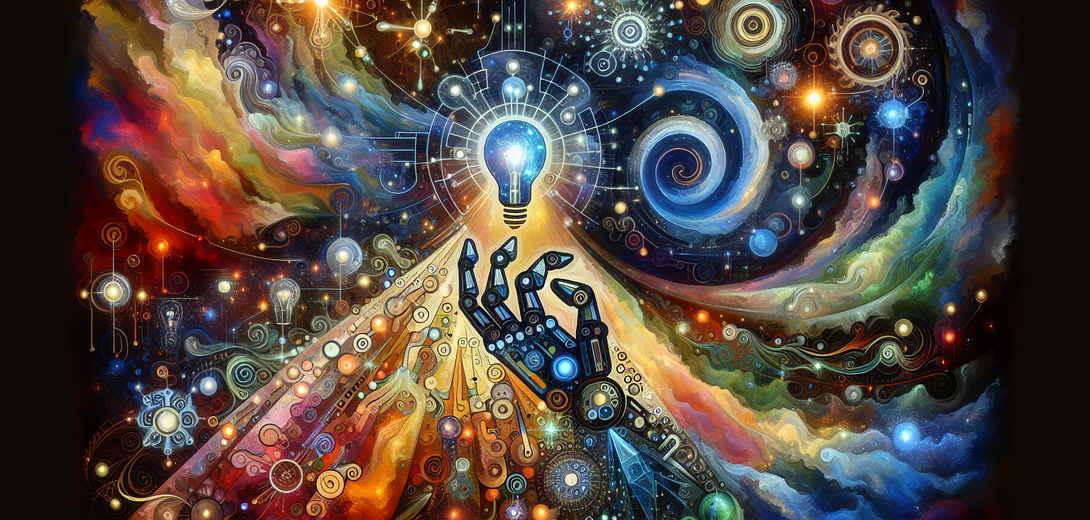
Supercharging Elm Development with Cloving CLI
Frontend development can be a tedious process, especially when working with strongly typed functional languages like Elm. Cloving CLI offers a way to accelerate your Elm development by integrating AI-assisted code generation into your workflow. Through various commands, Cloving allows you to enhance your productivity and improve code quality with ease. In this blog post, we will focus on how you can leverage Cloving CLI to streamline your Elm development process.
1. Getting Started with Cloving CLI
Before diving into code generation, let’s make sure Cloving CLI is set up in our environment.
1.1 Installation
First, you need to install Cloving globally using npm:
npm install -g cloving@latest
1.2 Configuration
Next, configure Cloving to use your preferred AI models by inputting your API key:
cloving config
Follow the on-screen prompts to set up your AI model, API key, and other preferences.
1.3 Initialize Your Project
To make the best use of Cloving, initialize it in your Elm project directory:
cloving init
This command will analyze your project and create a cloving.json
file to store important metadata about your application, ensuring Cloving understands your code structure and can provide more accurate suggestions.
2. Code Generation with Cloving
With Cloving set up, let’s look at how its AI-powered code generation can assist us in writing Elm code efficiently.
2.1 Example Task: Creating an Elm Module for a Counter
Assume you’re tasked with creating a simple counter component in Elm. Here’s how you could generate this using Cloving:
cloving generate code --prompt "Create an Elm module for a counter with increment and decrement functionalities" --module src/Counter.elm
Cloving will generate the Elm module, incorporating context like existing files and your specified prompt:
module Counter exposing (Model, Msg(..), update, view)
import Html exposing (button, div, text)
import Html.Events exposing (onClick)
type Msg
= Increment
| Decrement
type alias Model =
Int
update : Msg -> Model -> Model
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
view : Model -> Html.Html Msg
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (String.fromInt model) ]
, button [ onClick Increment ] [ text "+" ]
]
Within seconds, you have a fully functional Elm module for a counter component.
2.2 Adding Features: Reset Functionality
Cloving also simplifies incremental improvements. Suppose you want to add a reset button to set the counter back to zero:
- Regenerate the code with an updated prompt:
cloving generate code --prompt "Extend the Elm counter module to include a Reset button that resets the count to 0" --module src/Counter.elm
- Or, use the interactive chat to refine your existing file:
Then type your request:cloving chat -f src/Counter.elm
Add a reset button to reset the counter to zero
Cloving will produce updated code that includes a Reset
message and a corresponding button in the view
function.
3. Reviewing and Improving Code
Cloving makes it easy to not only generate code but also to review and revise it. After generation, you can:
- Review the generated code: Assess logic, style, or performance considerations.
- Ask Cloving for explanations or further revisions: For example, “Explain how the view function works” or “Refactor this to be more modular.”
This feedback loop helps you maintain high-quality Elm code and fosters learning about language features and best practices.
4. Generating Tests
Ensuring your Elm components work as expected is crucial. Cloving can generate unit tests tailored to your module:
cloving generate unit-tests -f src/Counter.elm
The tool will generate relevant test cases:
module CounterTest exposing (..)
import Test exposing (..)
import Expect
import Counter exposing (update, Msg(..))
suite : Test
suite =
describe "Counter.update"
[ test "Increment increases counter" <|
\() -> update Increment 0 |> Expect.equal 1
, test "Decrement decreases counter" <|
\() -> update Decrement 0 |> Expect.equal -1
]
You can refine these tests further by prompting Cloving to add additional scenarios—for example, testing boundary conditions or your new Reset
message.
5. Complex Tasks and Interactive Chat
5.1 Chat for Architecture Guidance
Beyond simple commands, if you encounter advanced Elm concepts like Navigation, HTTP requests, or Elm Ports, you can rely on Cloving’s interactive chat to provide insights, examples, or architectural suggestions:
cloving chat
Inside the interactive session, ask questions like:
I'm building an Elm SPA with multiple pages. How can I best structure my Main.elm to handle routing using Browser.application?
Cloving can provide you with code snippets, best practices, or references to official Elm patterns.
5.2 Chat for Debugging
When something isn’t working as expected, use the chat to troubleshoot:
The update function for my user form is not updating the model as expected. Could you review this code and suggest fixes?
6. Efficient Git Commit Messages
When your Elm components are complete, let Cloving help you generate insightful commit messages that accurately reflect your changes:
cloving commit
Cloving reviews your staged changes to create a descriptive commit message. You can accept or modify it before committing:
Add initial counter module with increment, decrement, and reset functionalities
This can save time and ensure clear, consistent commit logs.
7. Advanced Use Cases and Best Practices
7.1 Integration with Existing Elm Architecture
Elm follows a strict architecture with Model, Update, and View. Cloving’s code generation can adhere to this pattern, but you can guide it further by providing context:
cloving generate code --prompt "Create a Login module in Elm, adhering to our existing architecture, with a separate file for authentication messages" --module src/Login.elm
Including details about your architecture in the prompt helps Cloving produce code that fits seamlessly into your project.
7.2 Generating HTTP Request Functions
Working with external APIs can be tricky in Elm. Cloving can help you quickly generate HTTP request code—complete with decoders:
cloving generate code --prompt "Create an Elm module that fetches a list of products from a JSON API and decodes them into a list of records" --module src/Products.elm
7.3 Testing Complex Edge Cases
For more extensive coverage, prompt Cloving to generate tests for edge cases:
cloving generate unit-tests -f src/Counter.elm --prompt "Include tests for negative values and large increments"
7.4 CI/CD Integration
For teams or larger projects, integrating Cloving commands into your CI/CD pipeline can automate generation of new modules or test stubs whenever new features are added. This ensures consistent patterns across the codebase.
8. Tips to Maximize Productivity with Cloving
- Provide Clear Prompts: Include essential details in your
--prompt
so Cloving can tailor the generated code to your exact use case. - Iterate in Small Steps: Use the
chat
feature to refine code gradually rather than regenerating entire files from scratch. - Keep Cloving Updated: AI models and features are continually improving—updating Cloving ensures you’re leveraging the latest capabilities.
- Combine Human Expertise and AI: Cloving accelerates repetitive or boilerplate tasks, but your knowledge of Elm best practices remains invaluable.
- Embrace Elm’s Tooling: Pair Cloving with other Elm ecosystem tools (e.g., elm-format, elm-analyse) to keep your codebase clean, idiomatic, and error-free.
9. Conclusion
By integrating Cloving into your Elm development workflow, you can significantly accelerate your creation process using AI-guided code generation. Cloving offers a range of capabilities:
- Generating New Modules: Quickly spin up fully functional Elm code for things like counters, forms, or pages.
- Creating Unit Tests: Automate initial test scaffolding for your modules.
- Interactive Chat: Get on-demand help for complex Elm concepts or debugging assistance.
- Commit Message Generation: Maintain high-quality commit logs without extra effort.
Each of these features helps you focus more on designing a stellar user experience and less on repetitive coding tasks. Embrace Cloving CLI to transform your Elm development process, enhancing both productivity and code quality. And remember, while Cloving provides invaluable assistance, it’s ultimately your expertise that guides its AI to produce the best possible results.
Happy coding with Elm and Cloving!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.